- Create a new image
- Open, Show & Save images (PIL)
- Paste, Merge, Blend images (PIL)
- How to Draw shapes images (PIL)
- How to Crop an image (PIL)
- How to Add Text to images (PIL)
- How to Add Frames to images (PIL)
- How to Resize an image (PIL)
- Brightness, Contrast, Saturation
- Convert image to Grayscale | B&W
- Create Photo Collages
- Digital Image Color Modes
- Digital Image Basics
- Image Manipulation w/ PIL
- Batch Resize Multiple Images (PIL)
- Watermarking Images
- Difference Between 2 Images (PIL)
How to Resize an Image and Get Size in Python (PIL)
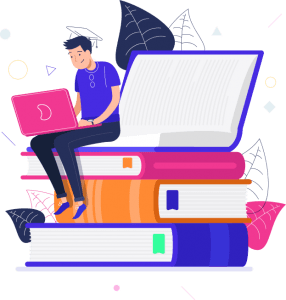
Introduction
- In this tutorial we will look at the PIL methods that can be used to resize an image.
- We will also check out another method that can be used to get the size (resolution) of an image.
Let’s first open an image so we can resize it.
from PIL import Image
file = "C://Users/ABC/Boat.jpg"
img = Image.open(file)
img.show()
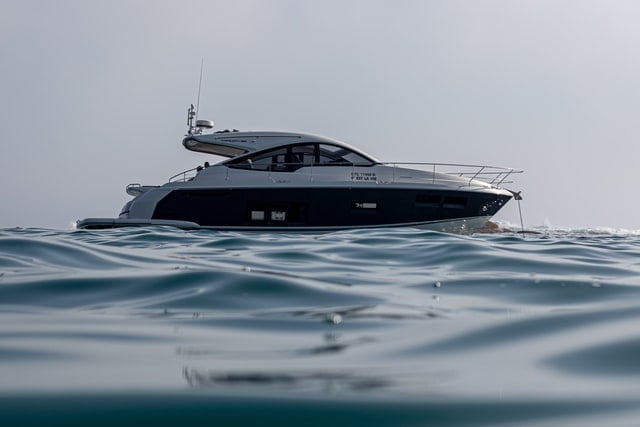
.resize() method
After opening an image you can use the .resize() method on it to create a new image with a new size.
- Don’t forget to pass the new size in tuples:
img.resize((x, y))
Example 1: Proportionate Resizing
After opening an image you can use the .resize() method on it to create a new image with a new size.
- Don’t forget to pass the new size in tuples:
img.resize((x, y))
- Also. don’t forget that you will have to assign the new image with new size to a new variable or you can also reassign it to the same variable and overwrite it.
img = img.resize((448,299))
img.show()
Example 2: Resizing with New Proportion
So, what happen if new size being passed to resize method is not proportionate to the original size?
In this case PIL will simply stretch the image to make it fit the new size. Let’s see an example.
- In this case we will pass (700,300) as the new size.
img = img.resize((700,300))
img.show()
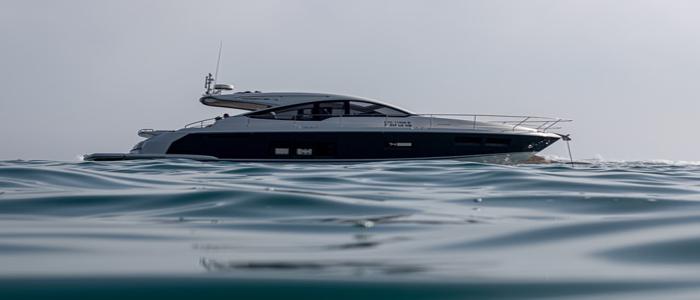
.size method
You can also get the size of an image using the .size property in PIL.
You don’t need to use parenthesis and you can simply print an image’s size as: print(img.size)
Example 3: How to get an image’s size
Let’s demonstrate getting the size of an image with an example:
from PIL import Image
file = "C://Users/ABC/Boat.jpg"
img = Image.open(file)
print(img.size)
(640, 427)
Example 4: How to resize an image as a percentage using size property
from PIL import Image
file = "C://Users/ABC/Boat.jpg"
img = Image.open(file)
img = img.resize((int(img.size[0]*0.2),int(img.size[1]*0.2)))
img.show()
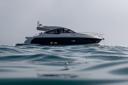
Summary
In this Python Tutorial, we’ve seen how to resize an image and different scenarios regarding the new size.
We have also seen hot to get the size of an image and we saw an example regarding how to make use of this information.
You can really see the power of PIL library in image processing as well as basic image editing tasks through these operations.