- Create a new image
- Open, Show & Save images (PIL)
- Paste, Merge, Blend images (PIL)
- How to Draw shapes images (PIL)
- How to Crop an image (PIL)
- How to Add Text to images (PIL)
- How to Add Frames to images (PIL)
- How to Resize an image (PIL)
- Brightness, Contrast, Saturation
- Convert image to Grayscale | B&W
- Create Photo Collages
- Digital Image Color Modes
- Digital Image Basics
- Image Manipulation w/ PIL
- Batch Resize Multiple Images (PIL)
- Watermarking Images
- Difference Between 2 Images (PIL)
How to Create a New Image with PIL
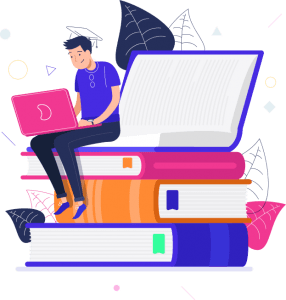
Introduction
- In this tutorial we will create new images using Python’s PIL library.
- Creating new image in PIL can be compared to creating new layers in photo editor’s such as GIMP or Adobe Photoshop. In most cases new image is created to create alpha transparency layer, edit the dimensions of an existing image, create a color tint, create text, draw on an image and/or blend combine multiple image layers in one image.
- In addition to simple new image creating operations with PIL library we will also look at some of the finer details such as creating new images with color or transparency.
Let’s first import the PIL library in Python and start with some simple operations.
.Image.new method
After importing PIL library’s Image module, we can create an image straight away.
from PIL import Image
You’ll have to pass two arguments minimum by default. These are:
- mode: common image color modes are RGB and RGBA. Feel free to check out this extensive tutorial for a detailed explanation of all color modes in Python’s PIL library.
- size: finally image size is another parameter that’s needed. You can go for the same size as an existing image you’re working on or some custom or standard sizes such as: (300, 300) or (1920, 1080)
Example 1: How to create new image
Let’s create a new image with transparency channel. It will have to be in RGBA mode which includes A (alpha) channel for transparency compared to RGB mode which doesn’t have transparency option.
Keep in mind though, while RGB works with common image file formats such as jpg RGBA only works with some special image formats like png.
from PIL import Image
new = Image.new(mode="RGBA", size=(1920,1080))
new.show()
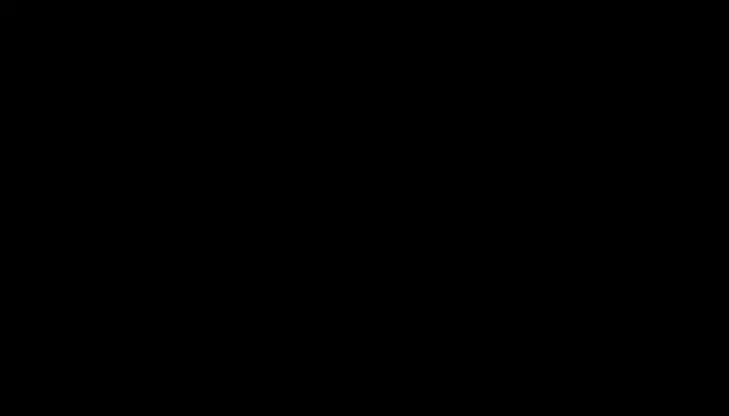
As you can see, color defaults to black when not specified.
Alternatively, you can even omit parameter names mode and size as following as long as you follow the right order:
from PIL import Image
new = Image.new("RGBA", (1920,1080))
new.show()
Example 2: How to create new image with color name
We can use “color” parameter to create an image with a colored background.
Check out the following Python code example:
from PIL import Image
new = Image.new(mode="RGBA", size=(1920,1080), color="pink")
new.show()
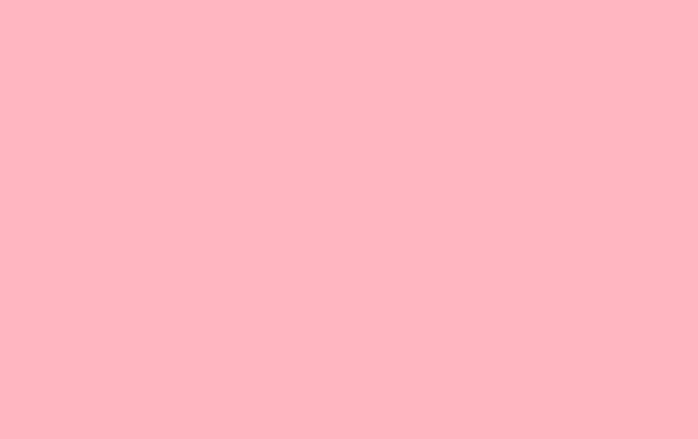
Example 3: How to create new image with RGB color
Although using color names is practical and works perfectly fine, you might want to use more specific color for your new image. In this example, let’s create an image in RGBA mode and specify a bluish color with approximately 50% transparency.
A bluish color can be created with a high value for B band of the RGBA channels. We’ll also use 127 for the A band which is roughly half of the full value 255.
Check out the following Python code example:
from PIL import Image
new = Image.new(mode="RGBA", size=(1920,1080), color=(10,10,255,127))
new.show()
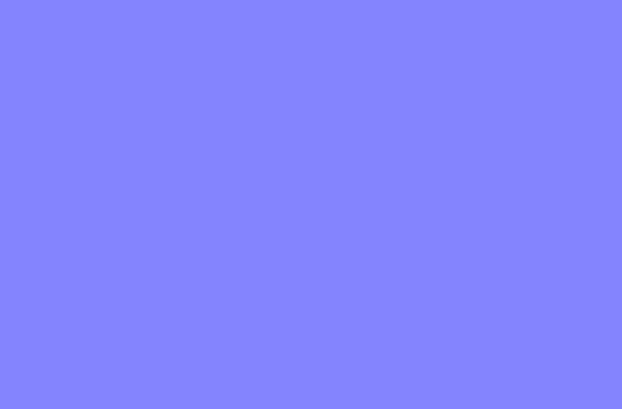
Although blue band is completely maximized we get a purple shade since transparency is at 50%.
Example 4: How to create new image with hex color
That’s not it yet. Python PIL has more tricks up its sleeves. You can also use specific hex color codes to create new images with very specific colors.
You can find a hex code of a color from free online color converters or color palette features of the photo editing applications such as GIMP.
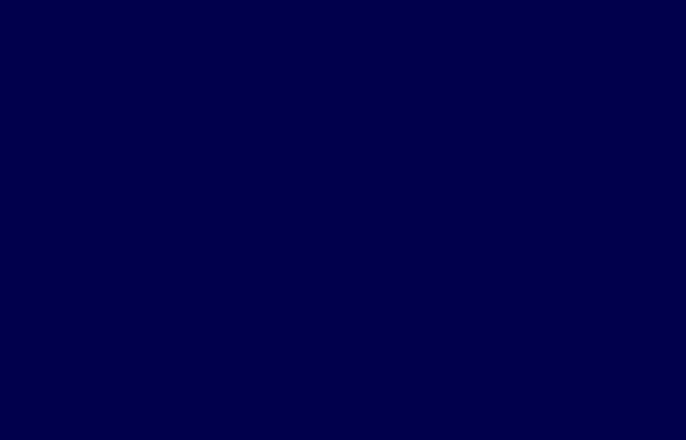
Here, this dark blue is created with hex code: #00004c
from PIL import Image
new = Image.new(mode="RGB", size=(1920,1080), color='#00004c')
new.show()
Summary
In this Python Tutorial, we’ve seen new method of Image module from PIL which can be used to create new images or layers depending on how you use it.
Since PIL has lots of rich features we have demonstrated multiple examples to give color to our new images. We have seen color name method and hex code method as well as RGB color methods.
Additionally we have created an image with alpha transparency band and manipulated the transparency feature to 50%.
Thank you for following out PIL image processing tutorials.