- Create a new image
- Open, Show & Save images (PIL)
- Paste, Merge, Blend images (PIL)
- How to Draw shapes images (PIL)
- How to Crop an image (PIL)
- How to Add Text to images (PIL)
- How to Add Frames to images (PIL)
- How to Resize an image (PIL)
- Brightness, Contrast, Saturation
- Convert image to Grayscale | B&W
- Create Photo Collages
- Digital Image Color Modes
- Digital Image Basics
- Image Manipulation w/ PIL
- Batch Resize Multiple Images (PIL)
- Watermarking Images
- Difference Between 2 Images (PIL)
Color Modes Explained for Digital Image Processing in Python (PIL) – with Python Examples
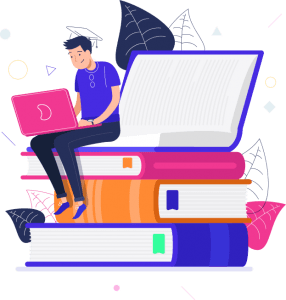
Introduction
An image’s color profile can be constructed in numerous ways.
RGB is a very common color mode for digital images. It uses 3 channels to represent color Red-Green-Blue. It also works with image file types such as jpg and bpm.
RGBA on the other hand is a color mode that can represent “transparency” through its Alpha channel. This type of image can only be saved as PNG or GIF image file formats which support transparency.
There are many other color modes and it can be crucial to know about color modes if you’re working with digital image processing. In this tutorial we’re going to explain you all the major color modes that are used in digital imaging and photography.
1 mode
1 color mode in Python PIL represents 1-bit image. As 1-bit can only take 2 values; 0 and 1, 1-bit images only have white or black colors without any shades of gray.
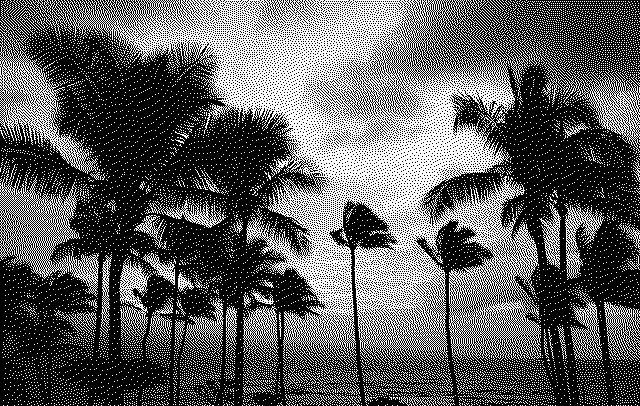
L mode
If you take a look at data of a L grayscale image you will get pixels 1-channel which can take any value between 0-255 inclusive.
You may ask why 255? It comes from 2^8 = 256. When you count 0 as well there are 256 total values an 8 bit image pixel can take.
LA mode
LA mode is very similar to LA mode except it has an extra channel called Alpha for transparency. This channel can also take 256 values which makes LA color mode 16-bit (8-bit plus 8-bit for alpha channel) or 32-bit (24-bit plus 8-bit for alpha) depth.
La mode
La grayscale color mode is very similar to LA grayscale color mode. The difference is alpha channel in La mode is predetermined.
Two different versions of alpha has to do with how alpha values are blended with the image.
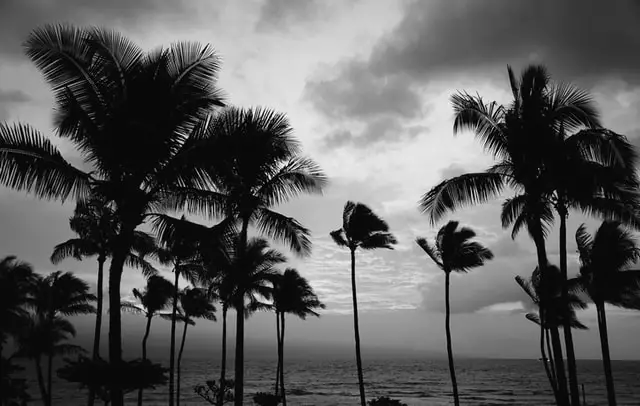
alpha vs premultiplied alpha
alpha (or unassociated or non-premultiplied) preserves both color or luminosity information and additionally stores an alpha channel which allows recovering color data or making new adjustments conveniently later on. This can be called as lossless transparency method.
premultiplied alpha on the other hand doesn’t preserve color data and instead contains the end result from blending transparency and colors on pixels hence the name premultiplied alpha. Other alpha is more common and regularly used in general since it’s a safer approach for digital image editing. In most cases you don’t want to not be able to adjust transparency independently later on.
alpha and file type compatibility:
- While png and bmp allow image mode with alpha (or unassociated or non-premultiplied) only such as LA or RGBA,
- TIFF and TGA file formats allow images with both alpha type color modes such as La, LA, RGBa or RGBA.
For example let’s say we have two pixels; one with alpha and one with premultiplied alpha. Let’s also say color modes are LA and La and they both have 50% transparency and are fully white color (value of 255). Here is how they will appear in different modes:
In alpha case: 255, 127 (LA mode)
In premultiplied alpha case: 127, 127 (La)
Alpha is already premultiplied. This is the reason why it’s impossible to recover the color after this operation as there is no way of knowing if blue channel had a value of 200 or 255 before (or any value above 127).
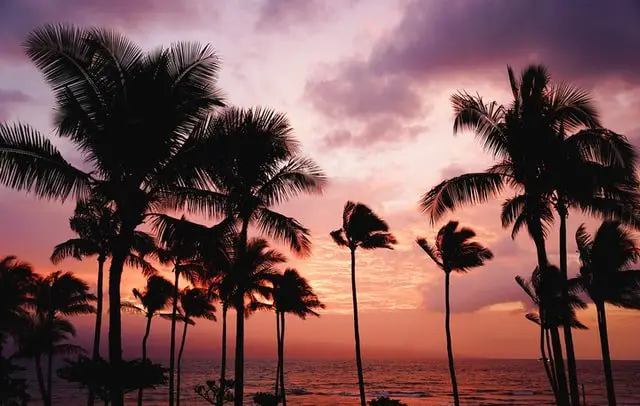
RGB Images
RGB images can take 3 values for each pixel. Each of these values represent one of the channels of RGB color mode which are red, green and blue. It’s probably safe to say that RGB images are the most common ones in use.
Since each of these three values for each pixel can take any value between 0 and 255, RGB images are also 24-bit images.
(8-bit * 8-bit * 8-bit)
RGB images can be saved as a number of file types such as: jpg, png, gif, bmp, tiff, tga etc.
Most electronic devices such as monitors, TVs, phone screens, projectors, wearable tech, car dashboards etc. use RGB mode because this method of color production is more convenient for physical production of these devices.
Interestingly, each pixel on your screen consist of 3 lamps (red-green-blue) and every time a digital image is projected on your screen each pixel’s color is controlled through the values that control these three lamps. Obviously we’re talking about tiny micro structures. Here is a really interesting video.
RGBA Images
Additionally RGBA is RGB with alpha transparency channel. It works similar to the LA mode explained above and in this case we end up with 4 channels of 8-bit which combined makes a 32-bit image.
RGBa Images
RGBa is RGB with premultiplied alpha transparency channel. It works similar to the La mode. You can find more information about premultiplied alpha above.
RGBX Images
X in RGBX represents an extra channel for an additional light source to support the image.
An example to RGBX can be RGBW technology which adds an extra white source to the RGB spectrum. This can have advantages such as achieving a more ideal white or warmer light colors etc.
Obviously in practice utilization of this mode will depend on the electronic constraints. If you have a panel that produces color with 3 led lamps you’re still restricted with RGB in practice regardless of your data.
CMYK color mode
CMYK is another color mode that’s capable of producing most ranges of the color scale. As it’s more suitable for physical printing most printers today use CMYK color mode. This doesn’t mean you have to convert your work’s color mode before printing as it’s automatically done by the printer during or right before printing takes place regardless of the source color mode.
Recommended Reading:
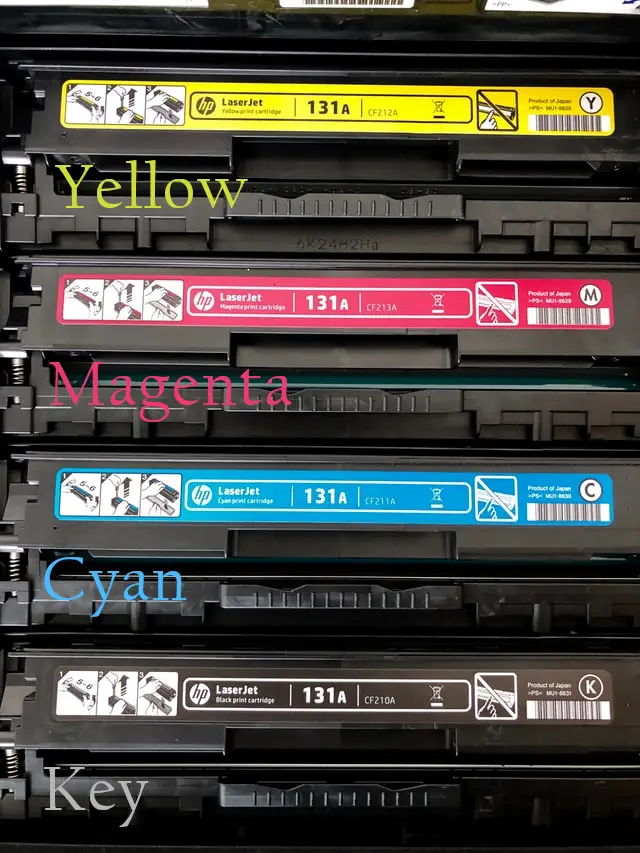
Additive Coloring vs Subtractive Coloring
CMYK uses subtractive coloring while RGB uses additive coloring.
Additive coloring produces colors by adding them. Primary colors (RGB) are additive because we get them directly from the light source and by adding them together we get light (full white color).
Subtractive coloring produces colors by subtracting certain wavelengths from the material such as paint or paper. Once the physical paint or ink mixes we don’t have the light from its source any more and colors we get are reflections from the material (or subtractions from the light source and not direct feed).
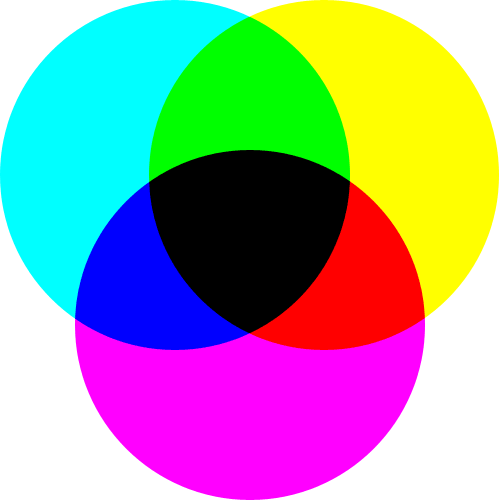
P mode
P mode is an interesting 8-bit color mode. It has one channel which takes 256 values that are used to map each value to a color. Technically it uses a palette of colors from 0 to 255. Compared to other color modes such as RGB which can produce ~16 million colors (2^24) P color mode images can appear crude.
However, with the amount of efficiency it’s still a very cool image mode that can represent a color mode decently for a fraction of the memory or computation resources. In fact P mode and similar indexed color modes were heavily used in the early days of personal computers due to hardware limitations.
Indexed color modes like P use a CLUT which is programmed to help the hardware register the right colors. CLUTs are Color Look-Up Tables that can return which value is mapped to which color.
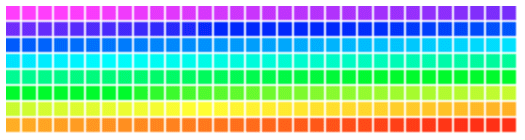
I mode (32-bit signed integer pixels)
This is the technical territory but roughly, integer mode represents colors with fixed points such as integers. This means no matter how big a range of values a pixel can take, there will be limited number of color representation.
However, in reality light (and basically color) can have infinite amount of variations. That’s where float mode comes in.
F mode (32-bit floating point pixels)
LAB mode
LAB color mode focuses on perception of colors with the human eye instead of production of colors by electronic devices (RGB) or material (CMYK). In LAB color mode:
- L stands for luminosity or light
- A is an axis of green and red
- B is an axis of blue and yellow
LAB’s numerical representation of colors is all about how a human would see a color and it doesn’t concern what machine or technology is being used to produce a color.
YCbCr Color Mode
Yet another color mode YCbCr uses a different numerical method to represent colors.
- Y stands for Luma component which is the light,
- Cb represents blue in relation to chroma (green) component,
- Cr represents red in relation to chroma (green) component.
In other words, Cb channel of YCbCr is an axis between green-blue (or Y-B) while Cr channel of YCbCr is an axis between green-red (or Y-R). This sort of color difference is sometimes also called chrominance.
Chroma means color intensity or saturation or vividness of a color that’s why YCbCr’s Y component is also called Achromatic and Cb and Cr are the chromatic components.
Another important point about YCbCr is that Y component in this mode is non-linear. This is an attempt to adjust the light relative to the color since human eye is more sensitive to light differences and less sensitive to color differences.
Why YCbCr Color Mode?
Technically RGB is losslessly convertible to YCbCr and vice versa. If you are interested in these conversions you can refer to another article’s Manual Pixel Manipulation section which uses Recommendation 601 and 709 to convert between RGB and grayscale images. The same standards from ITU can be used to convert between RGB and YCbCr images.
The usefulness of YCbCr mostly comes down to saving bandwidth during data transmission over the air. Over the air technology was a big deal before we had 4K streaming or even cable TV.
The ability to turn up light (Y) separately from chroma gives the ability to save bandwidth by turning down other two color channels (Cb and Cr) without being too recognized by the viewers.
HSV Color Mode
HSV stands for Hue, Saturation and Value color mode.
In this mode Hue represents the different colors while saturation is the vividness of the color and value is the value of the color itself.
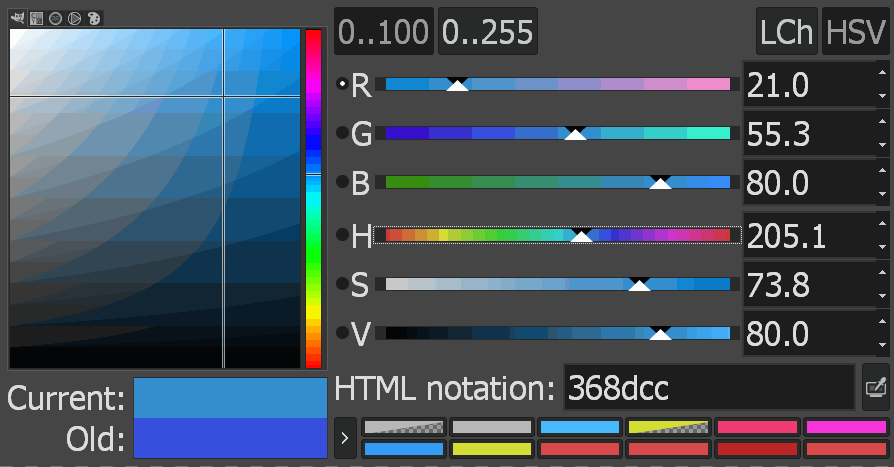
Digital Image Data Examples with Different Color Modes
Below you can see several examples representing different color modes such as 1, L, LA, RGB and RGBA
Example 1: 1-bit Image Data
If you take a look at data of a 1-bit image you will only get pixels with 1 or 0 values.
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
This is an example of a plain black image.
from PIL import Image
file = "C://Users/ABC/Motorbike.jpg"
img = Image.open(file)
Example 2: L mode
Here is a gray image
[122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122, 122]
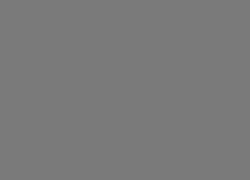
Here is a lighter gray image
[200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200, 200]
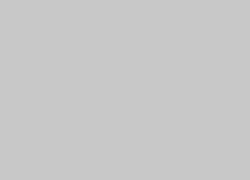
As 255 is white and 0 represents black, higer values mean lighter colors.
Example 3: RGB Image Data
Here is a green image’s partial data
[(100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10), (100, 200, 10)]
Each pixel is taking 100 for red, 200 for green and 10 for blue which results in a variety of green color as below.
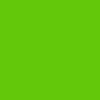
Example 4: RGBX Image Data
Here is a blueish RGBX image’s partial data
[(50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255), (50, 100, 255, 255)]
Each pixel is taking 50 for red, 100 for green, 255 for blue and an additional 255 for X channel.
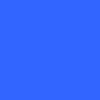
Example 5: CMYK Image Data
Here is an interesting practice you can also try. CMYK channels can be used to produce image examples below by maximizing each channel (CMYK) in each trial. Here is how the data for each image will look:
Cyan Channel:
[(255, 0, 0, 0), (255, 0, 0, 0), (255, 0, 0, 0), (255, 0, 0, 0)]
[(0, 255, 0, 0), (0, 255, 0, 0), (0, 255, 0, 0), (0, 255, 0, 0)]
Yellow Channel:
[(0, 0, 255, 0), (0, 0, 255, 0), (0, 0, 255, 0), (0, 0, 255, 0)]
Key Channel:
[(0, 0, 0, 255), (0, 0, 0, 255), (0, 0, 0, 255), (0, 0, 0, 255)]
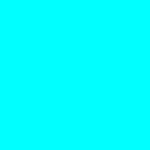
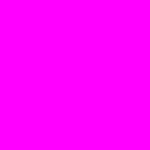
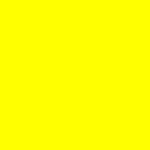
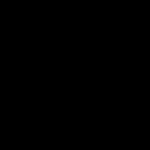
Here is the Python code that can be used to acquire the data above.
from PIL import
img = Image.new("CMYK", (150,150), color=(255,0,0,0))
img_data = img.getdata()
# img.show()
img.save("Desktop/colormode20e.jpg")
print(list(img_data))
Resources
Color modes are a very intriguing topic in digital imaging. It deals with light which is a very charming and curious topic by itself.
If you’d like to see more digital imaging tutorials with Python you can check out some of these resources:
Recommended Python PIL Tutorials
- How to open, show and save images with PIL
- How to Resize an Image and Get Size in Python (PIL)
- How to adjust brightness, contrast, sharpness and saturation of images (PIL)
- How to convert an image to B&W or Grayscale
- Digital Image Color Modes
- Image Manipulation w/ PIL
- Digital Image Basics
- How to Batch Resize Multiple Images (PIL)
- How to Watermark Images
- Finding the Difference Between 2 Images (PIL)
Summary
In this Python Tutorial, we’ve seen a number of color modes including monochrome color modes and colored color modes.
We have explained some interesting differences between multi-channel color modes such as RGB and CMYK.
We have also seen different varieties of channels such as RGBA, RGBa, RGBX, L, LA, La etc.
We have demonstrated the image data of some of these color modes through Python code examples and shared Python code in case you’re interested to get the same results yourself.