Lesson 6: Python Lists
- ESTIMATED TIME: 30 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: .append(), .insert(), .index(), .clear(), .remove(), .reverse(), .count(), sum(), min(), max(), random.shuffle()
- 14 EXERCISES
Lists are a great first step to advance your Python programming knowledge.
It allows collecting data. Or accessing data collections and working on them. So, congratulations, really. It may feel like nothing is happening but you are learning some very powerful concepts that you can use in future when coding amazing solutions.
Previously, we have seen lists in Python Data Structures. In this course we have included plenty of list methods that can be applied to Python lists These can be useful when working with lists which is more often than one would think.
It’s sort of a heavy Python course but it’s designed to get you comfortable with Python lists and every lesson that preceded it. It’s not so important that you memorize every single Python list method, but it’s important that you are comfortable working with lists. So try your best, there will also be plenty of other opportunities to master Python lists in future.
As we have seen, in Python lists are represented inside brackets and its elements are separated with commas.
Example : Simple List
>>> lst = [“Upper East”, “Wall Street”, “Broadway”]
>>> print(lst)
“Upper East”, “Wall Street”, “Braodway”
This is a simple Python list which consists of three string elements. Lists can also hold integers, floats, strings, dictionaries, tuples or even other lists or a combination of any of them.
Important Characteristics of Python Lists
Composite Nature: List is a very useful composite data structure in Python meaning it can hold multiple types of data. You can also call it a sequence, data sequence or collection.
Lists have an index order. This means every element inside a list has an index and this doesn’t change unless you change it. For some other data structures such as dictionaries this can be different. Think of index of a list as people on a line. You can give everyone a number from beginning to the end this would be indexing. And you can call them with their index number this would be accessing the element via index number.
So how does dictionaries have no index? They have something else called keys. But we will see that a bit later. Think of it like having a bag of mixed goods with no particular order.
Indexing: It’s important to note that indexing for lists starts with 0 (zero). Meaning first element in the list is actually zeroth element in Python world and last element’s index will always be one less than total element number since we started counting from zero. This is important to remember.
Mutability: Lists are also mutable, it’s kind of a fancy way of saying changeable. This means you can add and remove their elements as you wish. This is not the case with all Python Data Structures, for instance: tuples. You can’t just remove the last element of a tuple, once they are created they are final. This is sometimes useful when you know you won’t want any changes in your collection, such as month names in a year. But with Python lists you can change them, append to them, remove from them without any problem. We will also see Python tuples a bit later.
Let’s look at some examples.
Used Where?
- .append(): used for adding elements to a list
- .insert(): used for inserting element to a particular index of the list
- .index(): used to show the index of an element
- .clear(): clears all the elements of the list
- .remove(): used for removing an element of the list
- .reverse(): reverses the order inside the list
- .count(): used for counting how many elements there are in the list
- sum(): sums all the elements in the list
- min(): shows the element with lowest value in the list
- max(): shows the element with highest value in the list
Syntax do(s)
1) Lists are constructed using brackets: []
2) Elements inside your list should be separated with commas.
3) Syntax regarding data types need to be followed inside the lists. So if you have a string, it should be in quotes and integer, float and bool types shouldn't be in quotes.
Syntax don't(s)
1) Elements inside your list should be separated with commas
Let’s see a few simple Python list examples first.
Below is a list consisting of different types of elements. It’s a perfect example to demonstrate different nuances of the lists. Let’s see.
Example 1: List with different types of data
>>> p_data = [“Soho”, “Gramercy”, 23, 51, False, “False”, “22”]
>>> print(p_data)
“Soho”, “Gramercy”, 23, 51, False, “False”, “22”
1) First of all we can see there are different types of data inside the list: p_datatypes. These are string, integer, boolean, then string again.
2) Last two elements of the list are actually strings although they might look like bool and integer type since they are inside quotation marks they are registered as strings in Python.
3) Finally when we look at the indexing of the list, index 0 is the first element “Soho” and index 6 element is “22”, both strings. Although there are actually 7 elements in the list, index goes up to 6 since it starts with 0.
Accessing Elements: List elements can be accessed with their index number in brackets.
p_datatypes = [“Soho”, “Gramercy”, 23, 51, False, “False”]
for instance to access the first element “Soho” the correct syntax would be:
p_datatypes[0]
and to reach 23:
p_datatypes[2]
Let’s look at some examples.
Last element of the below list is a boolean with the value: False. We will access it inside the print function below.
Example 2: Accessing Python Lists via Index
>>> p_datatypes = [“Soho”, “Gramercy”, 23, False]
>>> print(p_datatypes[3])
False
And accessing the first element of the list.
Example 3: Python List and Index 0
>>> p_datatypes = [“Soho”, “Gramercy”, 23, False]
>>> print(p_datatypes[0])
“Soho”
Function 1: .append()
append() is probably one of the most commonly used list method. It’s used for adding elements to lists.
Now let’s see an example where .append() method is used to add a new element to the end of the list.
Example 4
>>> p_datatypes = [“Soho”, “Gramercy”]
>>> p_datatypes.append(“Tribeca”)
>>> print(p_datatypes)
p_datatypes = [“Soho”, “Gramercy”, “Tribeca”]
Tips
1- Reverse Indexing: Elements in the list can also be called starting from the end which is usually called reverse indexing. Unlike regular indexing reverse indexing doesn’t start with 0 and starts with -1.
So to access the last element of p_datatypes:
p_datatypes[-1] can be used and it would give you the last element of the list.
2- Similarly, p_datatypes[-2] would give you the second element from the end and so on.
This approach can offer great benefits in appropriate situations. It can be more practical and efficient to use since you don’t have to count the number of elements inside the list to access the last item or other items from the end. Another advantage would be evident if the size of the list is changing, again you wouldn’t have to bother with counting from the beginning to reach the last elements.
Let’s look at the examples.
Example 5
>>> p_datatypes = [“Soho”, “Gramercy”, 23, False]
>>> print(p_datatypes[-1])
False
Function 2: .insert()
.insert() is another useful method for lists. It can be used for inserting an element to the list by indicating index number.
Let’s see an example.
.insert() method takes two arguments inside its parenthesis: first, the index that the new element is desired to be inserted at, second, the new element.
Example 6
>>> p_datatypes = [“Soho”, “Gramercy”]
>>> p_datatypes.insert(0, “Tribeca”)
>>> print(p_datatypes)
p_datatypes = [“Tribeca”, “Soho”, “Gramercy”]
Example 7
>>> p_datatypes = [“Soho”, “Gramercy”]
>>> p_datatypes.insert(1, “Tribeca”)
>>> print(p_datatypes)
p_datatypes = [“Soho”, “Tribeca”, “Gramercy”]
Function 3: .index()
.index() helps identify the index number of an element.
Let’s see an example.
And here is a similar example for an empty dictionary assignment.
Example 8
>>> lst = [1, 33, 5, 55, 1001]
>>> a = lst.index(55)
>>> print(a)
3
Function 4: .clear()
.clear() method will clear all the elements of a list.
Example 9
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> lucky_numbers.clear()
>>> print(lucky_numbers)
[]
Function 5: .remove()
.remove() method will help you remove a specific element from a list.
Example 10
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> lucky_numbers.remove(101)
>>> print(lucky_numbers)
[5, 55, 4, 3, 42]
Function 6: .reverse()
.reverse() method will reverse the order of elements in a list.
Example 11
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> lucky_numbers.reverse()
>>> print(lucky_numbers)
[42, 101, 3, 4, 55, 5]
Function 7: .count()
.count() method is useful to find out how many occurrences there are in a list for a specific element.
In the example below, you can see that integer 5 is counted inside the list lucky_numbers. When we print, we get the output 1, meaning 5 occurs once in the list.
Example 12
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(lucky_numbers.count(5))
1
1 however, is not even in the list, so the output is 0.
Example 13
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(lucky_numbers.count(1))
0
Function 8: sum()
.sum function will give you the total sum of a list numbers.
Example 14
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(sum(lucky_numbers))
210
Function 9: min()
min() function will show you the minimum value of a list.
Let’s see an example.
Example 15
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(min(lucky_numbers))
5
Function 10: max()
max() function will show you the maximum value of a list.
Let’s see an example.
Example 16
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(max(lucky_numbers))
101
Advanced Concepts (Optional)
There are some other methods and Python functions we will see in the upcoming lessons. Some of them deserve their own lesson for the sake of keeping this lesson at a reasonable length.
These methods and functions can have a small twist in the way they work or cover a more broad functional perspective.
Let’s summarize them here ahead of the next lessons. Some of these built-in Python functions can be used with other types of data as well.
- .sort() method
- len() function (shared by many other data types)
- zip() function
- map() function
- filter() function
- List Comprehension (a super practical and useful approach to constructing lists that we will cover later.)
How to mix Python Lists
Shuffle Method
You can shuffle your lists in place thanks to random library.
random library is a default library that comes with Python and it has great features that everyone can benefit from (randrange and randint are some popular ones.)
But it also has a fantastic lesser known method called shuffle.
Using shuffle you can shuffle your lists in place. All you have to do is import random library and then use random.shuffle(sequence) syntax.
Check out this Python example:
import random
lst = [1,2,3,4,5,6,7,8,9,10]
random.shuffle(lst)
print(lst)
Output:
[7,3,5,2,6,8,4,1,9]
So, that’s about it. Lists are very handy and highly utilized in Python programming. So, congratulations on covering this fundamental topic.
We have some great exercises you can check out. As you code around and play with the concepts you will have a much deeper understanding of main Python concepts and programming in general which is usually the path to building amazing things.
In next lesson we will explore Python Tuples.
Next Lesson: Python Tuples
“Have you installed Python yet?”
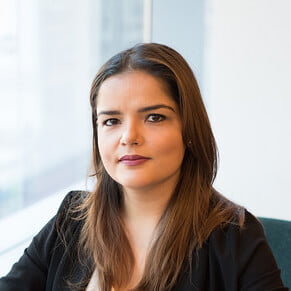