Lesson 8: Python Dictionaries
- ESTIMATED TIME: 23 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: .keys(), .items(), .get(), .clear(), .copy(), len(), type(), min(), max()
- 11 EXERCISES
- Structure: A dictionary consists of key-value pairs that are separated from other key-value pairs by commas. Inside the key-value pair, key and value is separated by a colon (:). First few examples below will clearly demonstrate this.
- Composite Nature: List is a very useful composite data structure meaning it can hold multiple types of data. You can also call it a data sequence.
- No order: Unlike lists and tuples there is no order or indexing inside a dictionary. You can think of key-value pairs inside a dictionary as if they are mixed in a bag. Although this can be a little confusing or restricting sometimes it is actually straightforward. There is no first or second key in a dictionary, they just randomly exist. Python’s dictionary structure is optimized for performance in a way that a value is accessed through its key.
Used Where?
Dictionarie are another very commonly used data sequences in Python. They can be used with pretty much anything that’s related to data.
- .keys(): used to show the keys in a dictionary
- .items(): used to create a key-value tuple (more on this later)
- .get(): used to return the value of a key
- .clear(): clears the entire dictionary
- .copy(): used to copy a dictionary
- len(): used to get the length of a dictionary
- type(): used to tell the type
- min(): used to identify the key with the lowest value
- max(): used to identify the key with the highest value
Syntax do(s)
1) Dictionaries are constructed using curly brackets: {}
2) Key-value pairs inside your dictionary should be separated with commas.
3) Keys and values should be separated with a colon (:) between them.
Syntax don't(s)
1) Keys inside a dictionary can't be other than strings, integers and floats.
But values of keys can be pretty much any data type.
2) Don't forget to use quotation marks if your key is a string.
Function 1: .keys()
.keys() is a useful dictionary method that will return a list of dictionary’s keys.
Below is a dictionary example with strings as keys and integers as values.
Example 1
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(p_ages)
{“Tom”: 32, “Jess”: 29, “Pete”: 18}
1) Please note that keys are string type in this dictionary and they are in quotes.
Below is a dictionary example that’s sort of opposite of the 1st Example: integers as keys and strings as values.
Example 2
>>> p_ages = {32: “Tom”, 29: “Jess”, 18: “Pete”}
>>> print(p_ages)
{32: “Tom”, 29: “Jess”, 18: “Pete”}
1) Please note that this time keys are integers and not in quotes and each key-value pair is separated from each other with commas.
Accessing Values: Dictionary values are accessed by their keys.
p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
to access Pete’s value we have to call it as below:
p_ages[“Pete”]
and to get 32:
p_ages[“Tom”]
Let’s look at some examples.
Let’s see an example with .keys() method.
Example 3
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(p_ages.keys())
[Tom, Jess, Pete]
Tips
Starting from Python version 3.9 we have exciting new operators coming to dictionaries that makes merging dictionaries much more practical.
1- Merge Operator ( | ): Merge operator will allow merging 2 dictionaries (dict type) simply by using “|” character.
2- Update Operator ( |= ): Update operator allows updating the first dictionary by another dictionary (dict type).
The difference between Python’s Merge operator and Update operators may seem subtle at first.
Merge (|) creates a new dictionary by merging both sides.
Update (|=) only updates the first dictionary.
Merge (|) operator can be a very practical way to merge dictionaries and make this commonly used data type easier to work with.
Example 4: New Merge Operator
>>> dict1 = {“x”: 1, “y”:2}
>>> dict2 = {“a”:11, “b”:22}
>>> dict3 = dict1 | dict2
>>> print(dict3)
{“x”:1, “y”:2, “a”:11, “b”:22}
Update (|=) operator is a great new way to update dictionaries.
Example 5: New Update Operator
>>> dict1 = {“x”: 1, “y”:2}
>>> dict2 = {“a”:11, “b”:22}
>>> dict2 |= dict1
>>> print(dict2)
{“x”:1, “y”:2, “a”:11, “b”:22}
Note: When there are overlapping keys (a Python dictionary can only have one unique key) key from the second dictionary will remain and first dictionary will be updated accordingly.
Function 2: .items()
.items() will return a list of tuples consisted of key-value pairs in a dictionary as tuples. The usefulness of this dictionary method will be more obvious in a more intermediate stage in your programming journey. Just take a mental note now and it might be a good idea to quickly revisit these functions, methods and lessons every once in a while.
Let’s see an example.
.items() method can be useful if you’re going to use indexing to access your data.
Example 6
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> a = p_ages.items()
>>> print(a)
[(‘Tom’, 32), (‘Jess’, 29), (‘Pete’, 18)]
Function 3: .get()
.get() is a useful method to access the values of a key in a dictionary.
Let’s see an example.
Example 7
>>> my_id = {“name”: “Sam”, “city”: “New York”, “age”: 30}
>>> print(my_id.get(“name”))
Sam
Let’s access the age value now, using .get() method.
Example 8
>>> my_id = {“name”: “Sam”, “city”: “New York”, “age”: 30}
>>> print(my_id.get(“age”))
30
Function 4: .clear()
.clear() method will clear all the elements of a dictionary.
Example 9
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> p_ages.clear()
>>> print(p_ages)
{}
Function 5: .copy()
.remove() method will help you remove a specific element from a list.
Example 10
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> lucky_numbers.remove(101)
>>> print(lucky_numbers)
[5, 55, 4, 3, 42]
Function 6: len()
.reverse() method will reverse the order of elements in a list.
Example 11
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> lucky_numbers.reverse()
>>> print(lucky_numbers)
[42, 101, 3, 4, 55, 5]
Function 7: type()
.count() method is useful to find out how many occurrences there are in a list for a specific element.
In the example below, you can see that integer 5 is counted inside the list lucky_numbers. When we print, we get the output 1, meaning 5 occurs once in the list.
Example 12
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(lucky_numbers.count(5))
1
1 however, is not even in the list, so the output is 0.
Example 13
>>> lucky_numbers = [5, 55, 4, 3, 101, 42]
>>> print(lucky_numbers.count(1))
0
Function 9: min()
min() function will show you the key with the minimum value in a dictionary.
Let’s see an example.
Example 14
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(min(p_ages))
“Pete”
Function 9: max()
max() function will show you the key with the maximum value in a dictionary.
Let’s see an example.
Example 15
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(max(p_ages))
“Tom”
Advanced Concepts (Optional)
1- .get() method is more powerful than simply accessing the value of a key.
If you add a second parameter to the .get() method, it will return that value in case the key you’re looking for doesn’t exist. Let’s see an example.
2- The new union operators explained in the tips: merge (|) and update (|=) can only be used with dict type. If you try to use them with various iterables including dict comprehension you will get a Type Error
Example 16
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(p_ages.get(“Jonah”, “Person not found”))
“Person not found”
Example 17
>>> p_ages = {“Tom”: 32, “Jess”: 29, “Pete”: 18}
>>> print(p_ages.get(“Jess”, “Person not found”))
29
Update: Lesson is updated with content regarding union operators that will be introduced with new Python 3.9 Version. You can also find 2 examples regarding new union operators for merging or updating dictionaries.
Next Lesson: Python Strings
“Have you installed Python yet?”
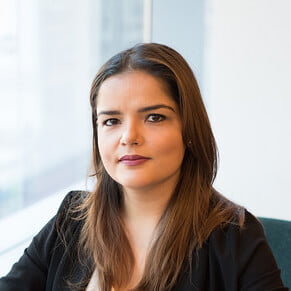