Lesson 1: print()
- ESTIMATED TIME: 4 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: print()
- EXERCISES: 3
Printing “Hello World!” is probably the most common programming ritual in all languages, so let’s kick start the first lesson with that.
Function 1: Print()
Print is like the first letter of the programming alphabet. Not to be confused with paper printing. print in Python displays data to the user.
Used Where?
print()
is used to display information to the user or programmer. It doesn’t change or affect values, variables, functions in any way and simply displays what you tell it to display.
- It can be very useful for the programmer to check certain values, make reminders or show messages to the user at different stages of the coding process.
Syntax do(s)
1) Print works with parenthesis.
correct usage is: print()
2) If you want to print a text, it will have to be in quotes:
print("Hello World")
3) #
symbol is used to put comments in your code. These comments don't get executed or printed they are simply notes intended to appear for other people looking at the code.
Syntax don't(s)
1) Don't print without parenthesis.print "Hello, World!"
Although this will work with Python 2, it won't work with Python 3 and you'll get an error.
2) You can't use quotes inside your print function when you're printing a variable. Quotation marks are only needed when dealing with text strings.
Example 1
>>> print(“Hello, World!”)
Hello, World!
Tips
"\a"
is a character that triggers a Warning chime in Windows environments. This creates a unique and fun situation where if youprint (\a)
in Python in a local Windows environment (such as with Anaconda, Spyder, Python in Command Console or PyCharm) you will actually produce this sound.
Although it’s jumping ahead, you can check out this simple alarm application in Python (#2).- As you can see, text inside the print function needs to be in quotes. Whenever you are printing a piece of text, this is the syntax you need to follow.
- If you are printing a variable, then you can’t use quotes and you need to type the variable name only. Such as in Example 2.
Example 2
>>> mymessage = “How about this?”
>>> print(mymessage)
How about this?
Example 3
>>> print(“Hello” , “my name is” , “John”)
Hello my name is John
Advanced Concepts (Optional)
1- Use of comma: You can print multiple values by separating them with commas.print("Hello" , "My name is" , "John")
2- sep parameter: On top of that you can use sep parameter inside your print function and this will separate each value with the separator you provided. print("Model S" , "Model 3" , sep="--")
3- end parameter: Also by default print() function enters a line value after each execution. You can avoid this if you’d like so by using end parameter. You can also assign it to be any character you’d like.
print("Model S" , "Model 3" , end="|")
print("100" , "200" , end="|")
print("USA" , "France" , end="|")
Example 4
>>># This is a comment. It can be useful to indicate something or share notes with the coder. But this line doesn’t get executed.
>>> print(“Hello” , “my name is” , “John”)
Hello my name is John
You can see the code includes a comment line, because the line starts with a #
sign. This line has no significance for the program and it is totally ignored by to program. You can think of it as a note mark on the code.
Next Lesson: Variables
“Have you installed Python yet?”
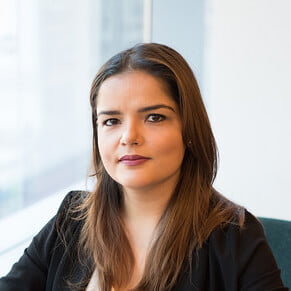