Creating your alarm in Python can be anything between fun, educating, useful and even liberating.
For example, Windows alarm application is pretty but sometimes it’s too tedious to adjust each parameter with the mouse and you may wish to just type the values (date, hour, minute, second, millisecond etc.).
With Python you can create an alarm that works exactly the way you intend it to do and it can be a great learning project as well.
So, we have prepared numerous different methods that can count as an alarm and hopefully you will find some of them cool.
Featured image credit: Laura Chouette
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
Creating Creative Alarms (w/ Python)
These ideas will construct the core programming of a Python alarm application. A combination of:
- Task Scheduling with Python method
- Plus one of the Python alarm methods mentioned here
will give you an OS level DIY alarm. If you won’t turn off your system or if you don’t mind leaving the alarm script or your programming IDE running in the background you can avoid using the Task Scheduler extension. In that case your alarm will probably be set for shorter time periods such as a few minutes to hours.
Here are 5 Alarm Ideas using Python and some of its default libraries!
1- Using Webbrowser Library to Create a Python Alarm
Let’s start with the web browser method. You can easily open any page on your default web browser using webbrowser library.
import webbrowser
webbrowser.open("https://www.youtube.com/watch?v=x0mmajhLKh8")
So, just find a video you’d like to set as an alarm. It can be your favorite music or something inspirational on Youtube or another streaming/video site.
Maybe check out “New Day Video Clip” from 50 Cent, Alicia Keys and Dr. Dre for ideas.
Alternatively you can set the link to
- your favorite movie clips,
- upcoming movie trailers,
- your favorite Youtube Vlogger,
- nature sounds (ocean, forest, wind, rain etc.)
- bird sounds
- cute animals
- puppy videos
- funny videos etc.
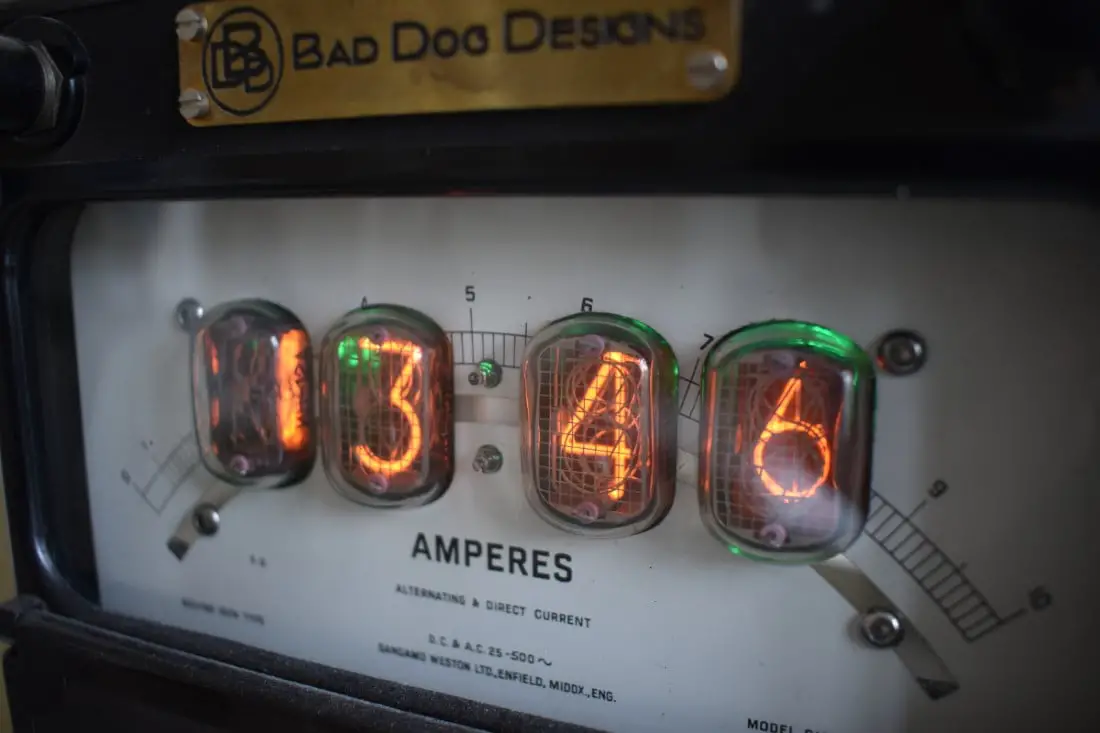
2- Python Alarm Using Print Function
This one is my absolute favorite. Its beauty is its simplicity.
Apparently if try to print “\a”, this triggers a Windows notification sound (I think it’s called warning sound. Whatever its called its a sound we’ve all heard thanks to Windows Operating System and it’s somewhat alarming indeed. )
So all you have to do is to execute this code: print ("\a")
For a more sophisticated code you’d wanna put it in a loop or it will be too short to act as an alarm. But once in a loop it actually works surprisingly well. Very elegant and creative in my opinion.
import time
for i in range(5):
time.sleep(1)
print("\a")
Also, we’re using Python’s convenient time library to create a little bit of delay between each chime.
This will create 5 Windows sounds with approximately 1 second breaks. Feel free to adjust for loop parameters according to your taste and heaviness of your snooze maybe.
You can also create a nested loop to produce multiple blocks of these with a longer break in between. Check out this code:
import time
for i in range(10):
for i in range(4):
time.sleep(0.5)
print("\a")
time.sleep(3)
It will produce 10 blocks of alarm tunes which will consist of 4 chimes. Between blocks there will be a 3 second break. I don’t know if it’s just me but it’s mind blowing to me to be able to create something like this from print function and a couple of Python loops. Well, there is also the time library but I think you know what I mean.
3- Win32api's Beep Method for a Proper Frequency Implementation
Now, this is also simply a beep but it’s a more proper and complete method than the print method. Using win32api library you can actually make a precision on the frequency of the sound and adjust its length to the milisecond.
This opens the door for lots of sound creativity. Check out this alarm tune creation method which will produce unique sequence of sound every time it’s run because of the implementation of random library’s randint method:
Note: Frequency range is limited to approximately something like 37 to 32000.
Lower frequencies will create a very deep sound while high frequencies produce high pitch sounds.
Also, random library’s ranint simply produces a random integer between the values its given.
import win32api
import random
for i in range(5):
win32api.Beep(random.randint(37,10000), random.randint(750,3000))
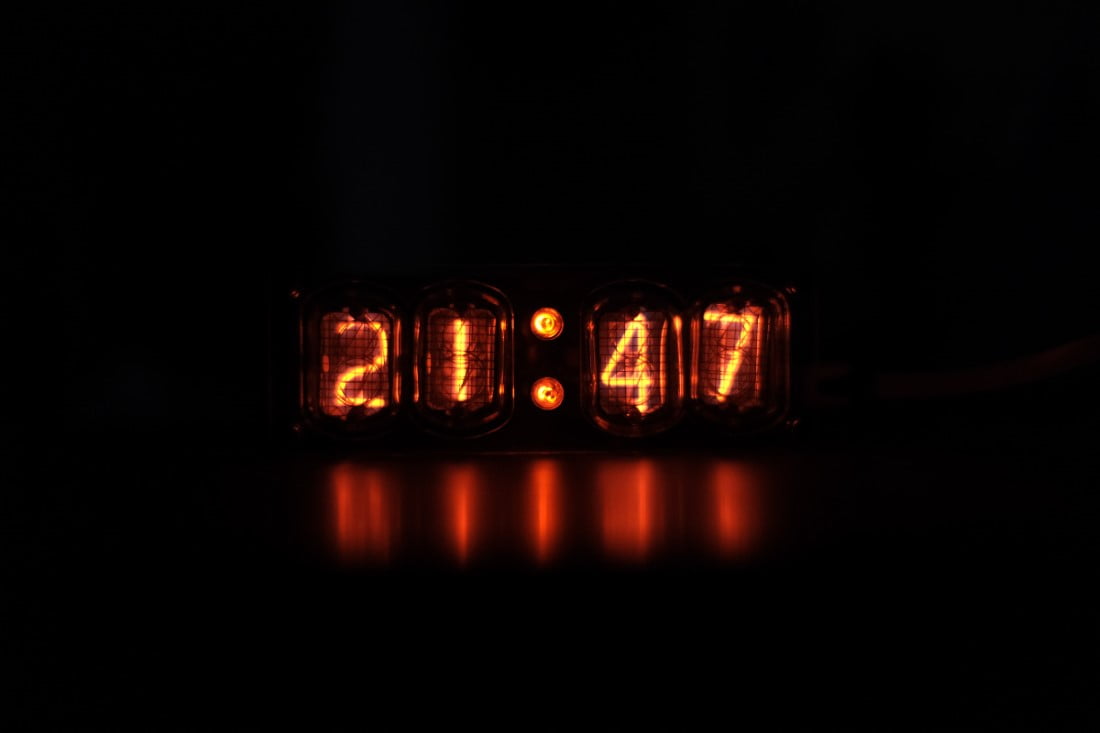
Increasing frequency and decreasing length is another approach to creating an alarm in Python using Beep method. If this paranoia evoking – bunker style alarm won’t wake you up I don’t know what will. But hey, no one said it was gonna be a pleasant alarm. If you’re in the market for a pleasant alarm check out the next idea! 🙂
x=37
y=2500
for i in range(13):
win32api.Beep(x,y)
x+=100
y-=120
4- Playing a Local Audio File Using Python
This might be the most appropriate approach if you’re particular about using an exact sound, chime, tune, song, audio book, speech, podcast or any other audio file for your self-made alarm. In my opinion bird chirping, ocean waves or funny audios make excellent alarm tunes.
It can also be helpful in cases where you are missing an internet connection temporarily such as on a boat or off-grid cabin. Playing a local audio is really easy with Python:
- All you have to do is import os library and make use of its .system method.
import os
f="birds_chirping.mp3"
os.system(f)
If you use a path just make sure to use double backslashes instead of regular slashes. If it still doesn’t work try changing the direction of the slash. There can be conflicts due to operating system’s directory notation. This is usually the case for Windows to recognize the path.
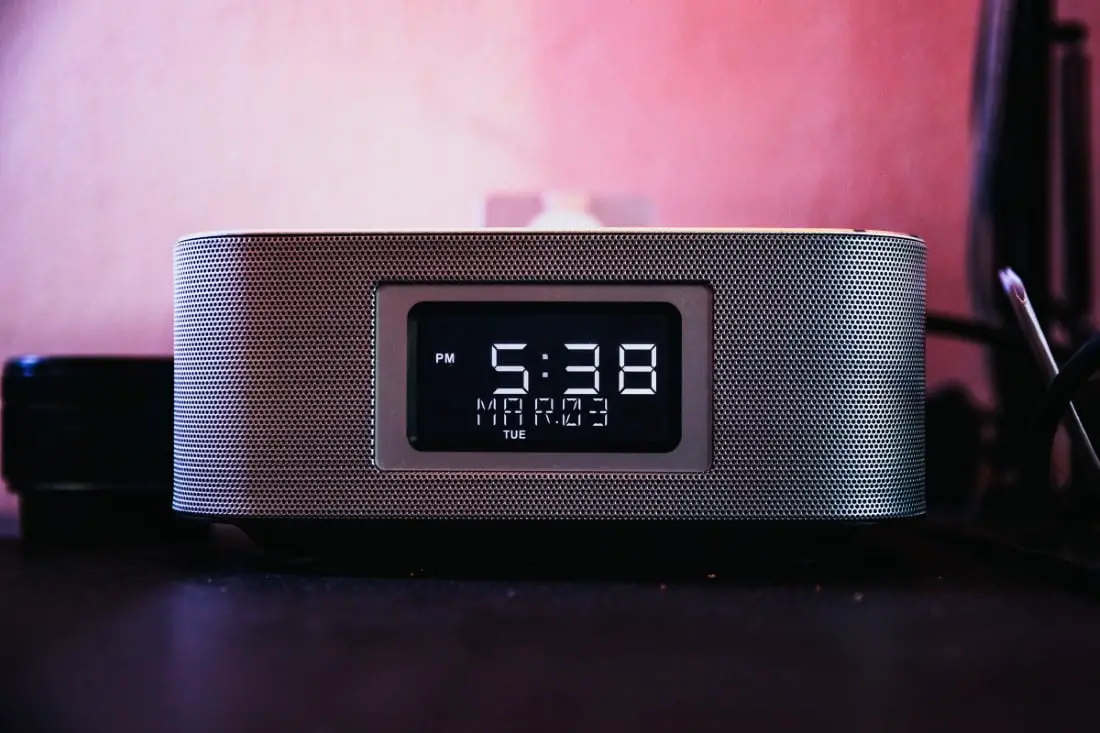
You can also use os.system method to open any other file such as video clips or movies.
5- Win32com.client for Python Alarm with Video Files
You can also use the Run method from win32com.client to run any file in your system locally. You can also use this method as an alternative to webbrowser because if you pass a web link as an argument to Windows Run it will automatically Run it in your default browser.
import win32com.client
shell = win32com.client.Dispatch("WScript.Shell")
shell.Run("Matrix_IV.mp4")
Bonus: - Python Alarm Library on Pypi
If you’re still not satisfied with none of these methods or if you don’t want to deal with the Task Scheduling part (which is a fantastic method to improve your coding skill-set and practice Python) you can also check out this interesting alarm library created for Python here.
That’s all the methods we have. Thanks for reading! I hope you found some of these methods useful and entertaining. Using Python for personal solutions is a great way to immerse yourself in coding and engage with Python learning at a more conscious level.
Please also consider sharing this article with your audience, followers, friends and family so they can also learn or hear a few simple but cool programming tricks.
And also, you can check out some other cool Python scripting ideas from HolyPython.