Lesson 4: Type Conversion
- ESTIMATED TIME: 12 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: type(), int(), float(), str()
- 8 EXERCISES
type()
function returns the type of your object. It’s a straightforward function and the examples below can help you have a better understanding of how it works.
We will also look at some other functions that can help you convert the type of a data. These are: int()
, float()
and str()
.
Function 1: type()
type()
is another basic cool function in Python that helps you learn the type of a variable. Now that we know about variables and print
function. By learning type()
function we can print the type of a variable.
Used Where?
type()
is used to identify the type of a variable.- Type information can be critical for your programming. And it can be helpful in so many ways.
- For instance, your program might collect data and you might want to know the type of that data.
- You might also want to make specific executions on specific data types. i.e.: arithmetic calculations on integrals or floats. Letter or word searching on strings etc.
- Also, there can be conditional situations where you’d like to treat certain data depending on their types or take action based on this information.
- There will be many other cases where
type()
function can offer value to you as a programmer.
Syntax do(s)
1) You can convert int
or float
types into str
type as str
can have any letter, number or symbols in them.
2) Str
type is always represented in quotes while int
, float
and bool
types don't have quotes.
Syntax don't(s)
1) You can't convert a string consisting of letters into integer or float type.
Example 1
>>> game_count = 21
>>> print(type(game_count))
<class ‘int’>
Tips
1- In next lessons we will learn about more complex data types such as lists, tuples and dictionaries.
These are usually referred to as composite data types as well because they can be consisted of multiple data types and values. type()
function can also be used on identifying these data structures.
Example 2
>>> person1_weight = 121.25
>>> print(type(person1_weight))
<class ‘float’>
Function 2: int()
int()
function you can try to convert from another type of data to int type. Below you can see on the first line a number in quotes gets assigned to variable inc_count.
Example 3
>>> inc_count = “2256”
>>> print(type(inc_count))
>>> print(inc_count)
>>> inc_count = int(inc_count)
>>> print(type(inc_count))
>>> print(inc_count)
<class ‘str’>
2256
<class ‘int’>
2256
Because of quotes, variable registers this data as a string. Following commands of print, print the initial type and value of the variable on the screen and then you can see int()
function converting the variable to int type.
After which print function will show that the type of the variable now is int.
You can note that before and after the int conversion data appears similarly on the output screen suggesting it would be hard to distinguish the types without type()
function.
Function 3: float()
float()
is a function that can be used to convert data to float type from other types.
Example 4
>>> inc_count = “2256”
>>> print(type(inc_count))
>>> print(inc_count)
>>> inc_count = float(inc_count)
>>> print(type(inc_count))
>>> print(inc_count)
<class ‘str’>
2256
<class ‘float’>
2256.0
Function 4: str()
int()
and float()
, str()
helps you convert a data to str from another type. Example 5
>>> inc_count = 2256
>>> print(type(inc_count))
>>> print(inc_count)
>>> inc_count = str(inc_count)
>>> print(type(inc_count))
>>> print(inc_count)
<class ‘int’>
2256
<class ‘str’>
2256
Advanced Concepts (Optional)
1- Not any type of data can be converted to any other type of data. For instance if a string is consisted of letters you will get an error when you try to use int()
function on it simply because letters can’t be converted to integer type.
However, if a string is consisted of numbers, it will be a successful operation.
2- You can convert pretty much any type of letter, number or character to string type. This will be the equivelant of typing your numbers in quotes and this representation stands for string type in Python.
Example 6
>>> my_data = “Anything”
>>> my_data = int(my_data)
ValueError: invalid literal for int() with base 10: ‘any’
As you can see in this example, since the variable is consisted of letters, Python is having a hard time executing the int()
function and throws an error.
We will look into these errors in detail in the upcoming lessons as it can be important to understand what they mean and what might be causing them.
Example 7
>>> main_check = True
>>> print (type(main_check))
class ‘bool’
When we check the type of True we see that it’s a boolean. This should be no surprise since True is not inside quotes.
Example 8: Boolean to String
Just for fun let’s see if we can convert bool type to str type in Python.
>>> main_check = True
>>> main_check = str(main_check)
>>> print(main_check)
>>> print (type(main_check))
‘True’
class ‘str’
Great! Looks like we can also convert boolean to a string. While boolean has one element as a whole which is True boolean, string ‘True’ actually has 4 elements. These are characters: ‘T’, ‘r’, ‘u’ and ‘e’
Example 9: String to Boolean
Let’s see if we can also reverse it going from a string ‘True’ to bool this time.
>>> main_check = ‘True’
>>> main_check = bool(main_check)
>>> print (type(main_check))
class ‘bool’
Perfect. That also worked.
In last two Python examples, aside of data types and type conversion we are also practicing some other fundamental Python concepts we have seen in previous classes. These are: Python variables and print function. If something is not clear at this point you should really revise those lessons and maybe check out our online Python exercises.
Going forward we will build on the topics so far and your coding knowledge will gradually advance.
Python type conversion finishing thoughts
In this Python lesson we have elaborated data type concept and looked closely to some of the most common data types in Python. These types are namely: int, str, float and bool. All very fundamental concepts.
We have seen how to transition between some of these Python data types. This only works when type conversion makes sense. We have also seen that, for instance, a string like “Hello World!” can’t be converted to integer while a string like “99” can be. This is understandable given the logic of numbers.
With this knowledge we are getting ready to work with all kinds of data that exist in the world. Audio data, signal data, text data, number data, price data, digital image data in the end everything is data in digital world. But our knowledge would be missing without data structures to store data in most convenient ways. That will be our next Python lesson. And later on after some Intermediate Python Lessons you will start to understand the possibilities and to feel digitally liberated.
Next Lesson: Data Structures
“Have you installed Python yet?”
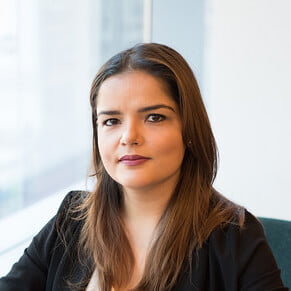