Python Type Conversion Exercises
Let’s check out some exercises that will help you really understand the type() function and type conversions in Python.
Exercise 4-a
Using type() function assign the type of the variable to answer_1, then print it.
type() function shows the type of a variable.
You can directly type the type() function inside the print() function or another elegant way of doing this is assigning another variable (such as answer_1) to the type of the first variable and printing that variable inside the print() function.
answer_1 = type(men_stepped_on_the_moon)
Exercise 4-b
Using type() function assign the type of the variable to answer_2, then print it.
type() function shows the type of a variable.
You can directly type the type() function inside the print() function or another elegant way of doing this is assigning another variable (such as answer_2) to the type of the first variable and printing that variable inside the print() function.
answer_2 = type(my_reason_for_coding)
Now let’s see some more exercises about converting data types.
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
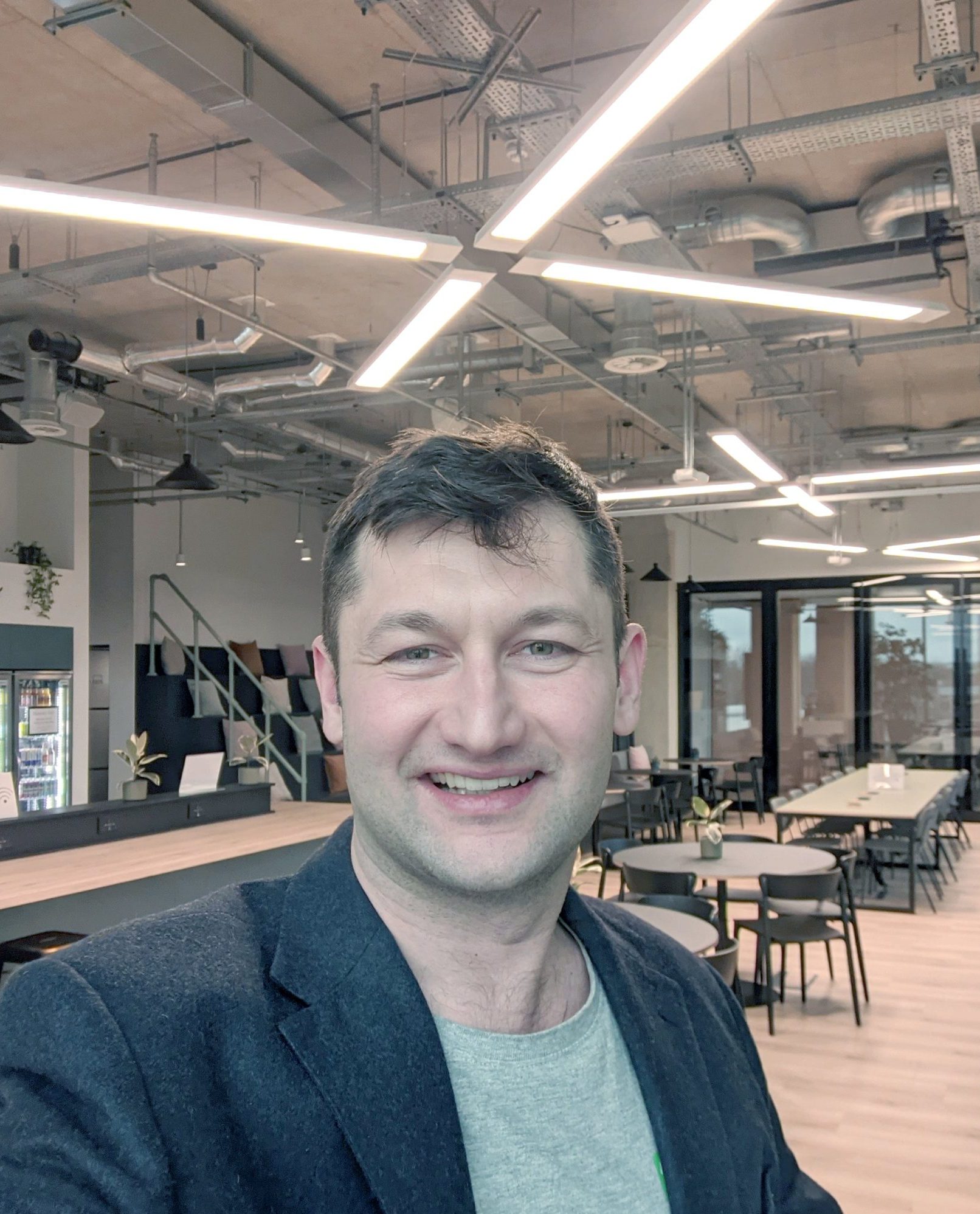
Founder of HolyPython