Python Variable Exercises
Let’s check out some exercises that will help you understand Variables in Python better.
Exercise 2-a
Below is a good example of mixing numbers and text inside the print() function
Assign: 3 to variable glass_of_water.
Exercise 2-b
Let's try to see what happens after assigning a new value to our variable. Note that program gets executed line by line.
Place the variable: glass_of_water inside the print function and observe what happens.
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
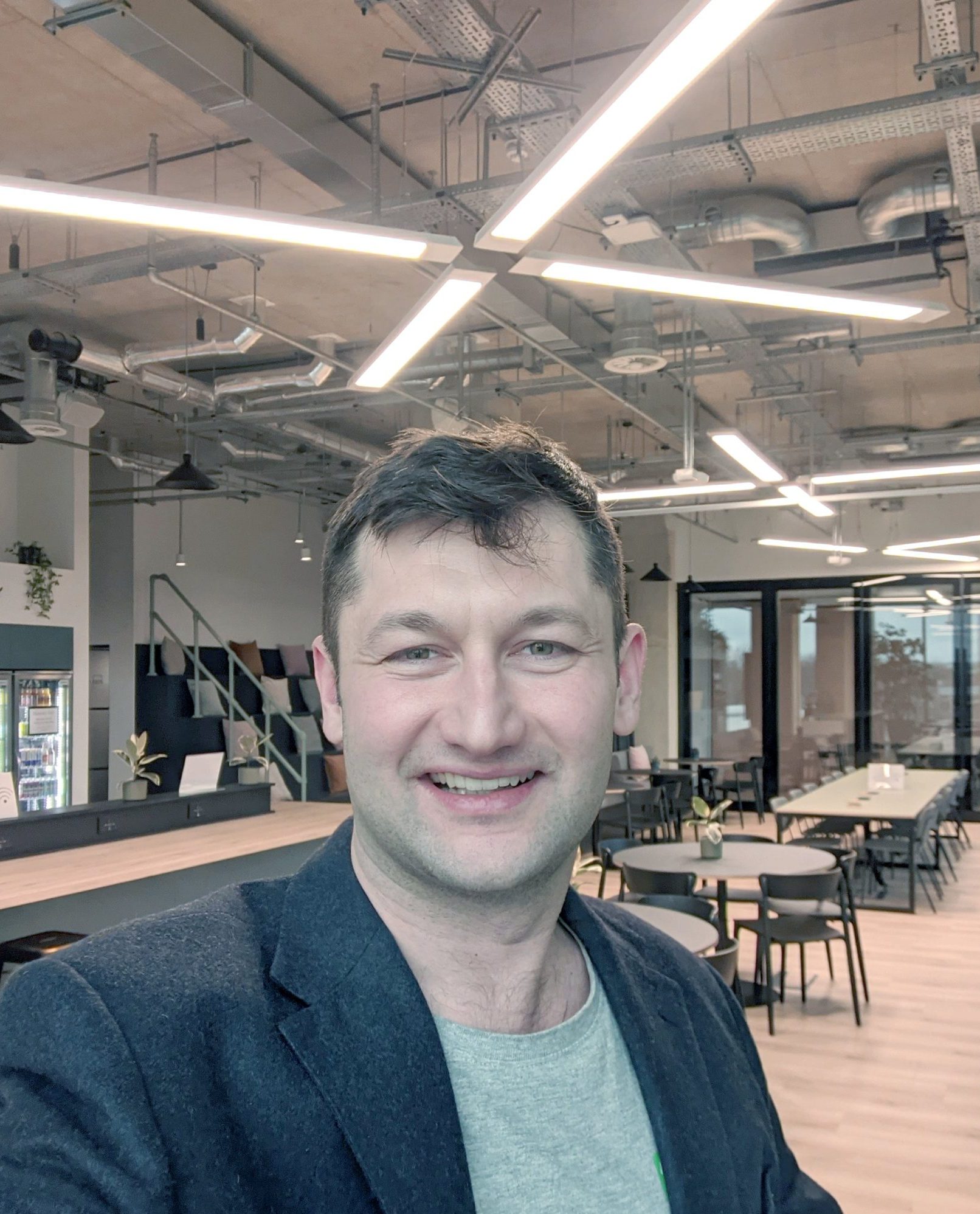
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython