Python String Exercises
Let’s check out some exercises that will help you understand Python strings better.
Exercise 9-a
Assign the string below to the variable in the exercise.
"It's always darkest before dawn."
Exercise 9-b
By using first, second and last characters of the string, create a new string.
Exercise 9-c
Replace the (.) with (!)
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
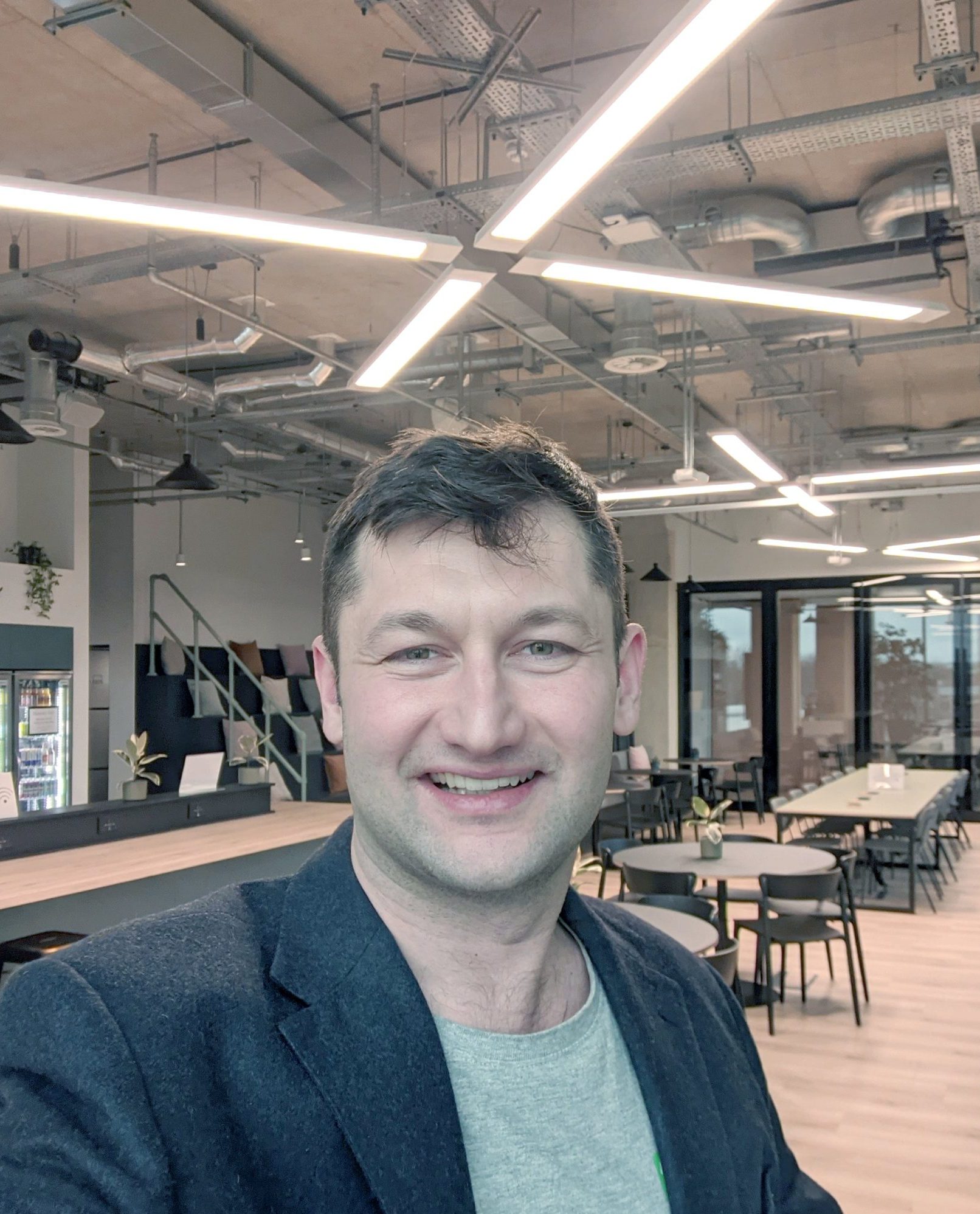
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython