Python Tuple Exercises
Let’s check out some exercises that will help understand tuples better.
Exercise 7-a
Assign the first element of the tuple to answer_1 on line 2
Exercise 7-b
This time print the third element of the tuple.
Exercise 7-c
Print the second from the last element of the tuple.
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
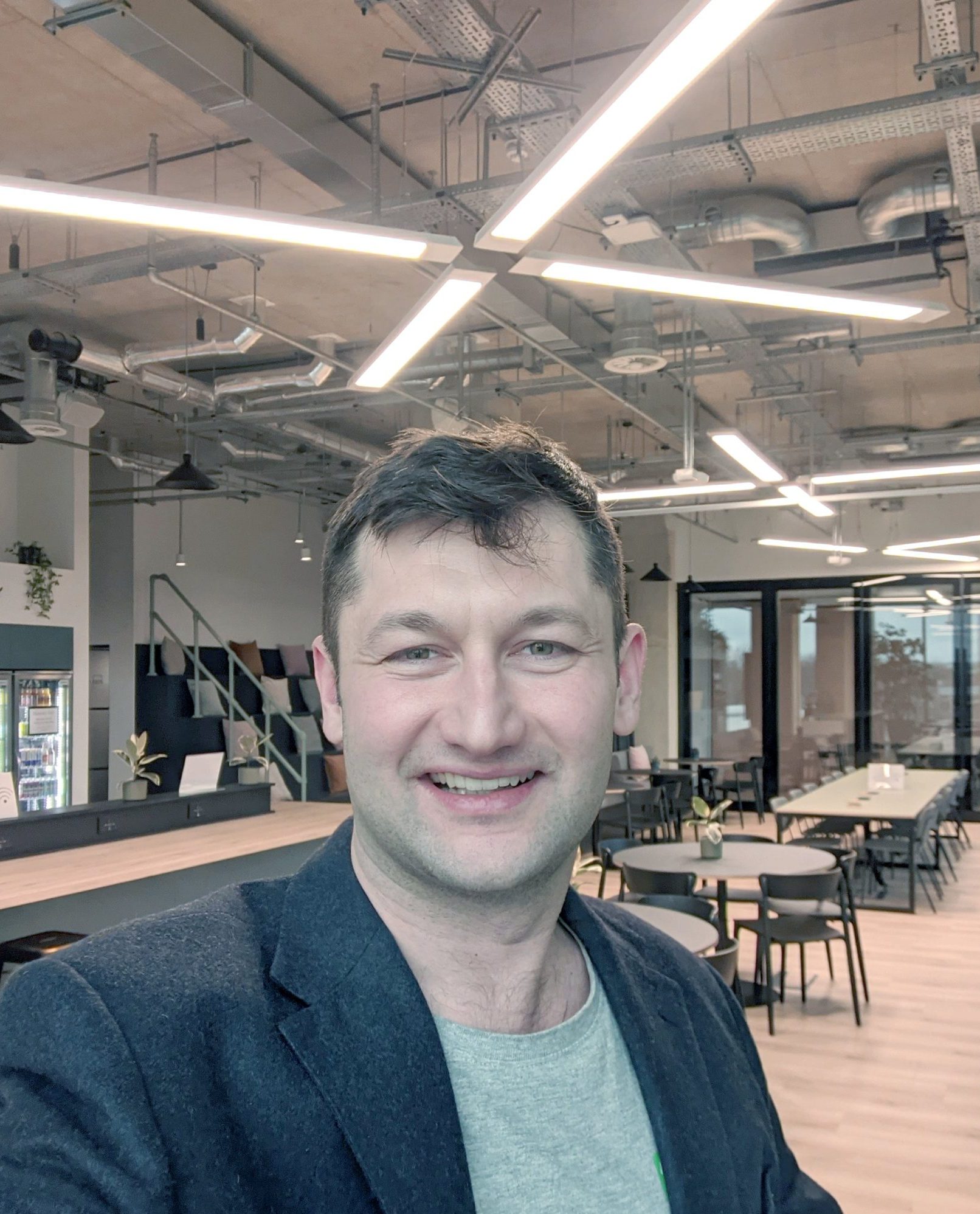
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython