Python Slicing Exercises
Let’s check out some exercises that will help you understand and master how Python’s Slice Notions, Slicing and Advanced Slicing works.
Exercise 17-a
Slice the word until first "a". (Tosc)
Exercise 17-b
Slice the word so that you get "cana".
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
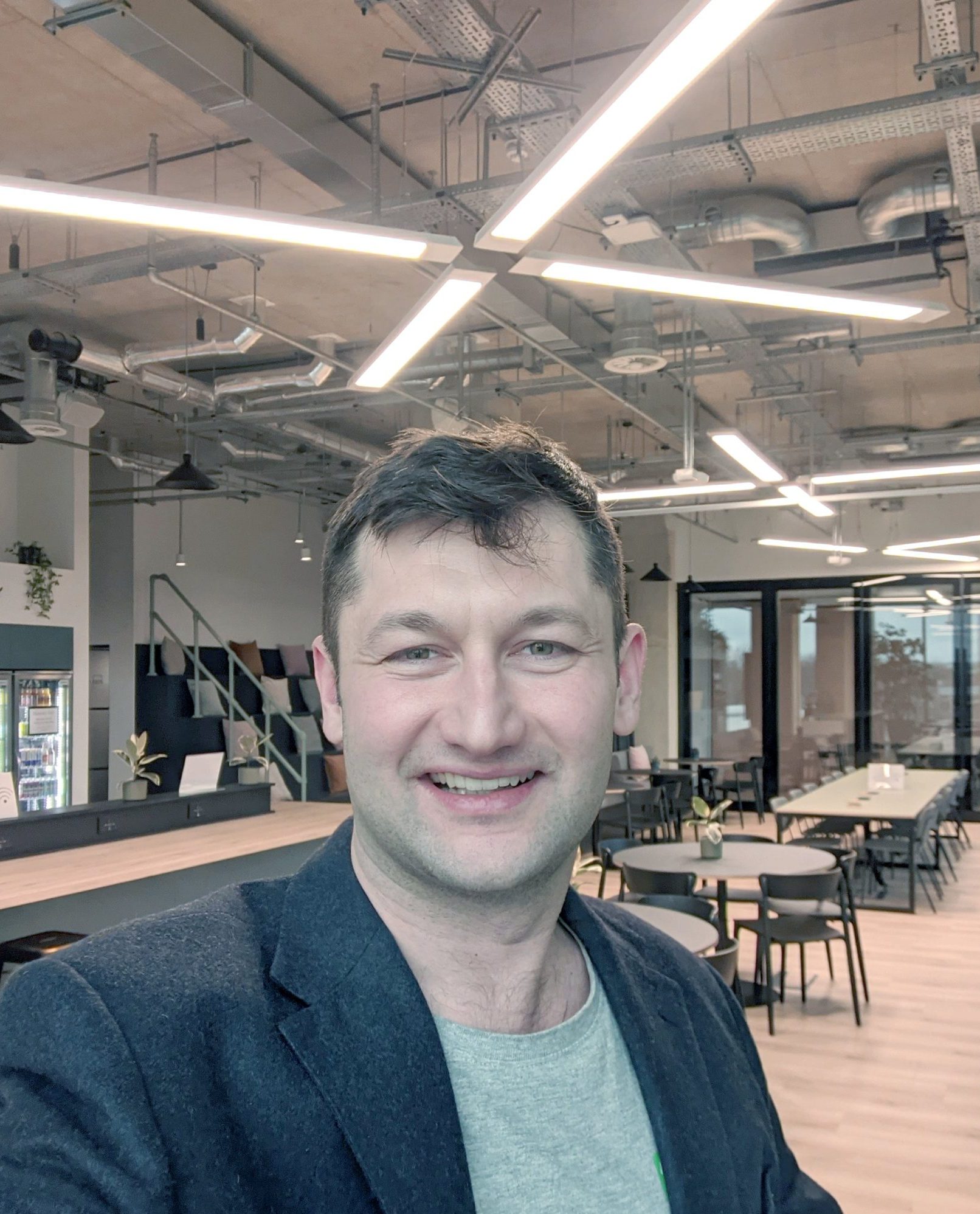
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython