Python Operator Exercises
Let’s check out some exercises that will help understand Python Operators better.
Exercise 18-a: Assignment Operator =
Let's start with the most basic . Assign a list of colors to the variable: ["yellow", "white", "blue"]
Exercise 18-b: Division Operator /
Assign division of a to b to the variable.
Exercise 18-c: Addition using +=
Add 100 to the variable using +=.
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
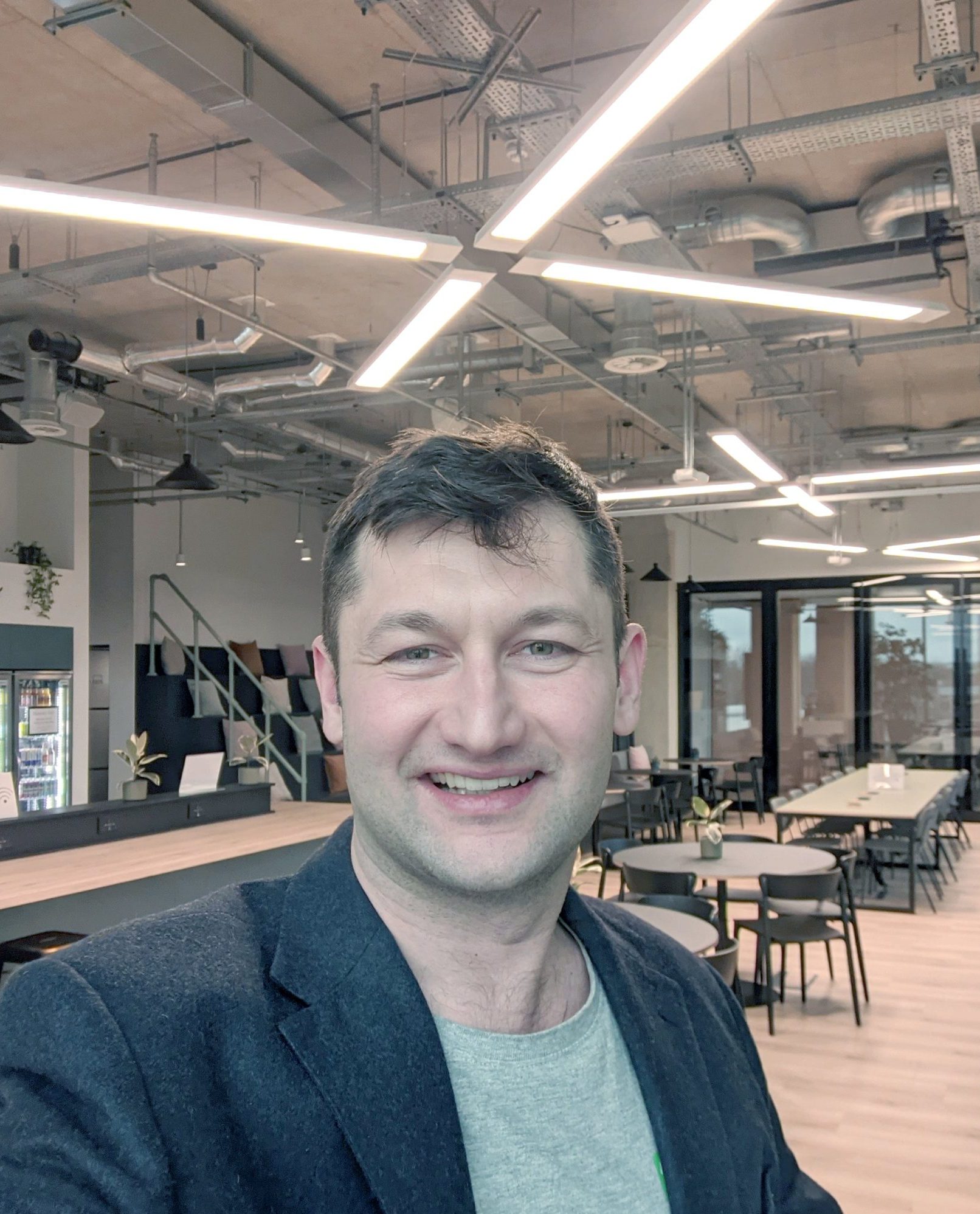
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython