Python Len Exercises
Let’s check out some exercises that will help understand len() function better.
Exercise 10-a
Using len() function find out how many items are in the list.
Exercise 10-b
len() function can also tell the length of a string.
Find out the length of the string given below.
Exercise 10-c
How many keys are there in the dictionary?
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
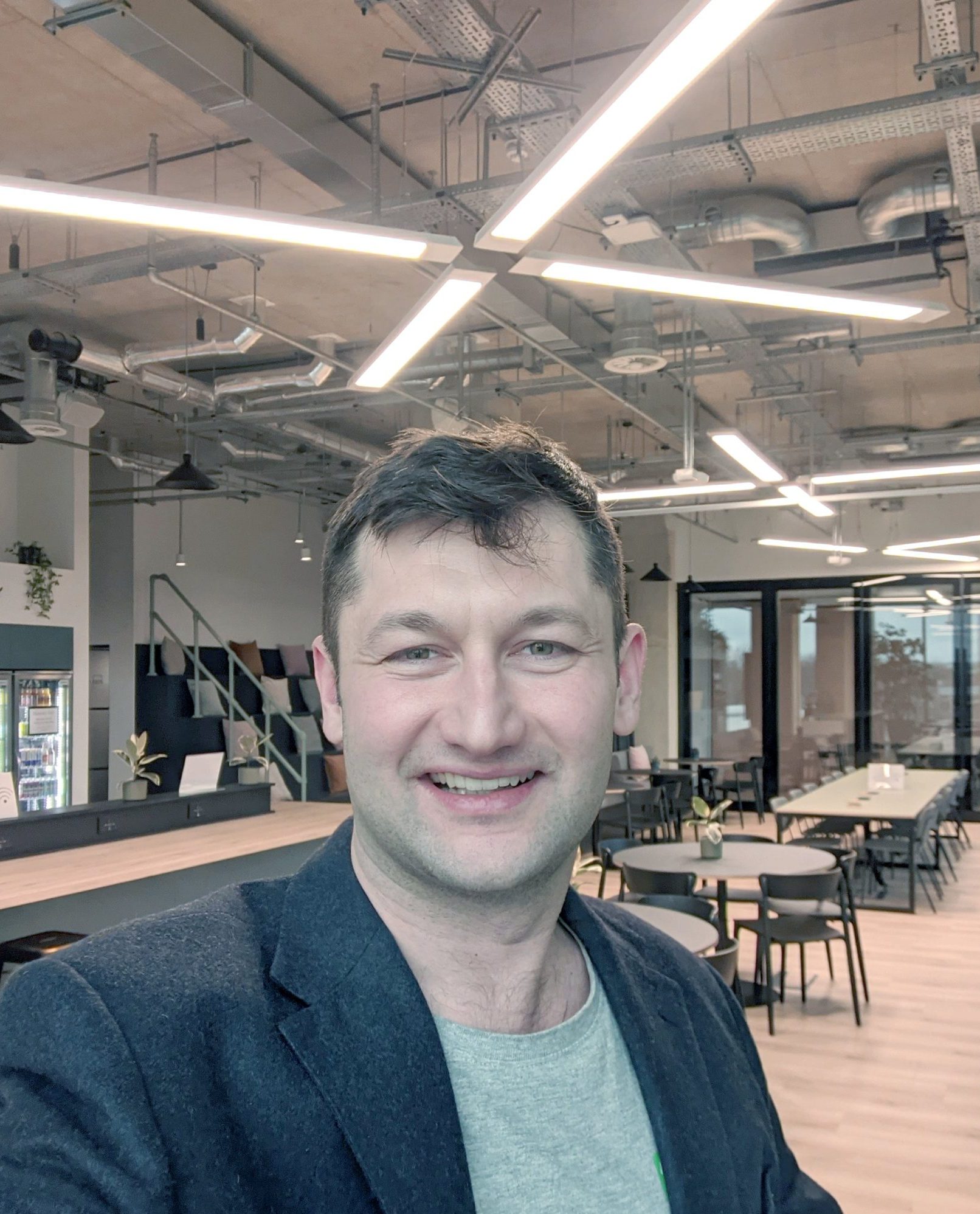
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython