Python User Function Exercises
Let’s check out some exercises that will help understand “Defining Functions” better.
Exercise 16-a
Define a function named f_1 which will print "Hello World!"
Exercise 16-b
Now define the same function f_1 and assign it to variable ans_1. See what happens.
Exercise 16-c
Now define the same f_1 function this time so that it returns a value instead of just printing it..
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
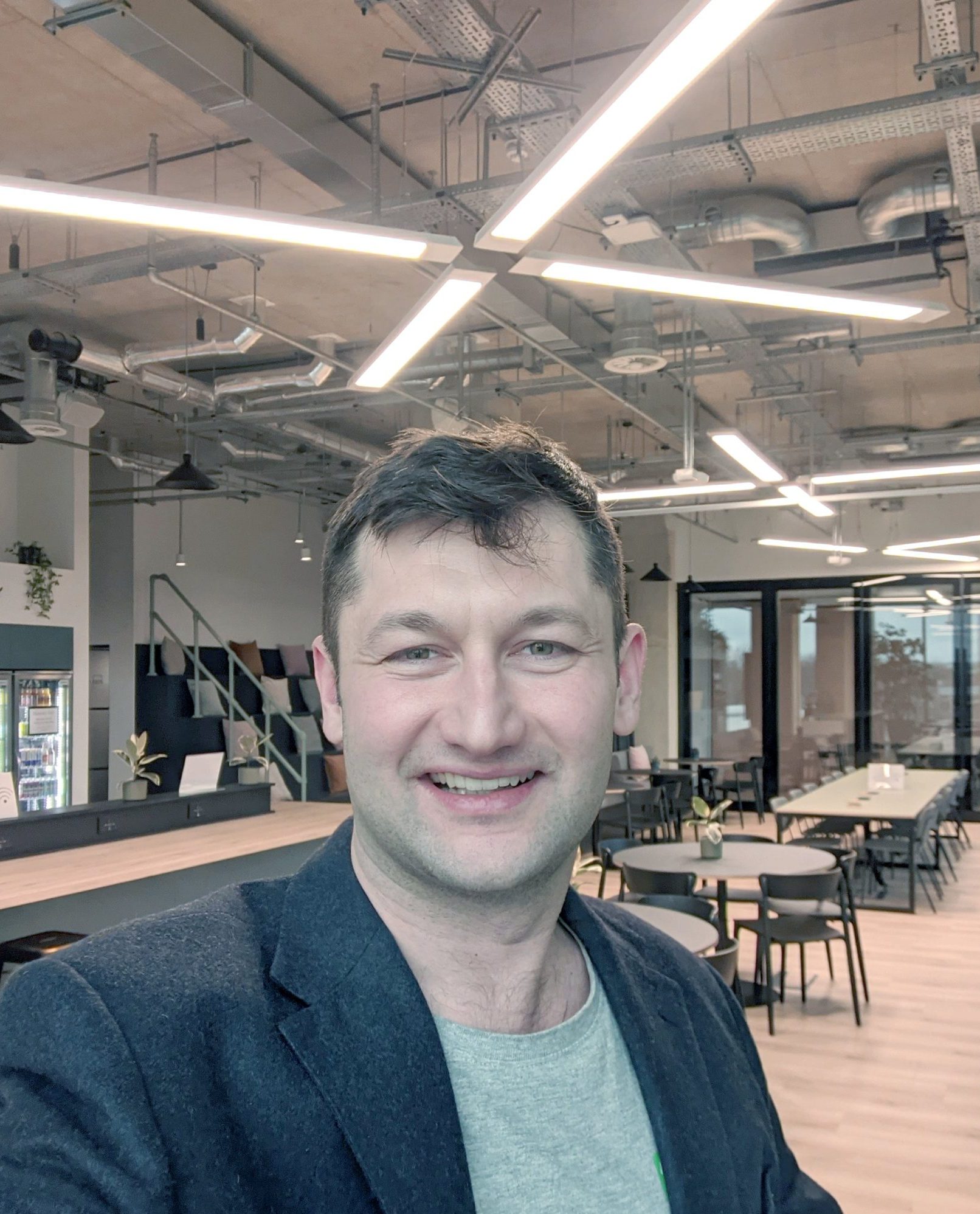
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython