Python Sort Exercises
Let’s check out some exercises that will help understand Python’s .sort() method better.
Exercise 11-a
Sort the list in ascending order with .sort() method.
Exercise 11-b
This time sort the countries in alphabetic order.
Exercise 11-c
Now sort the list in descending order with .sort() method.
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
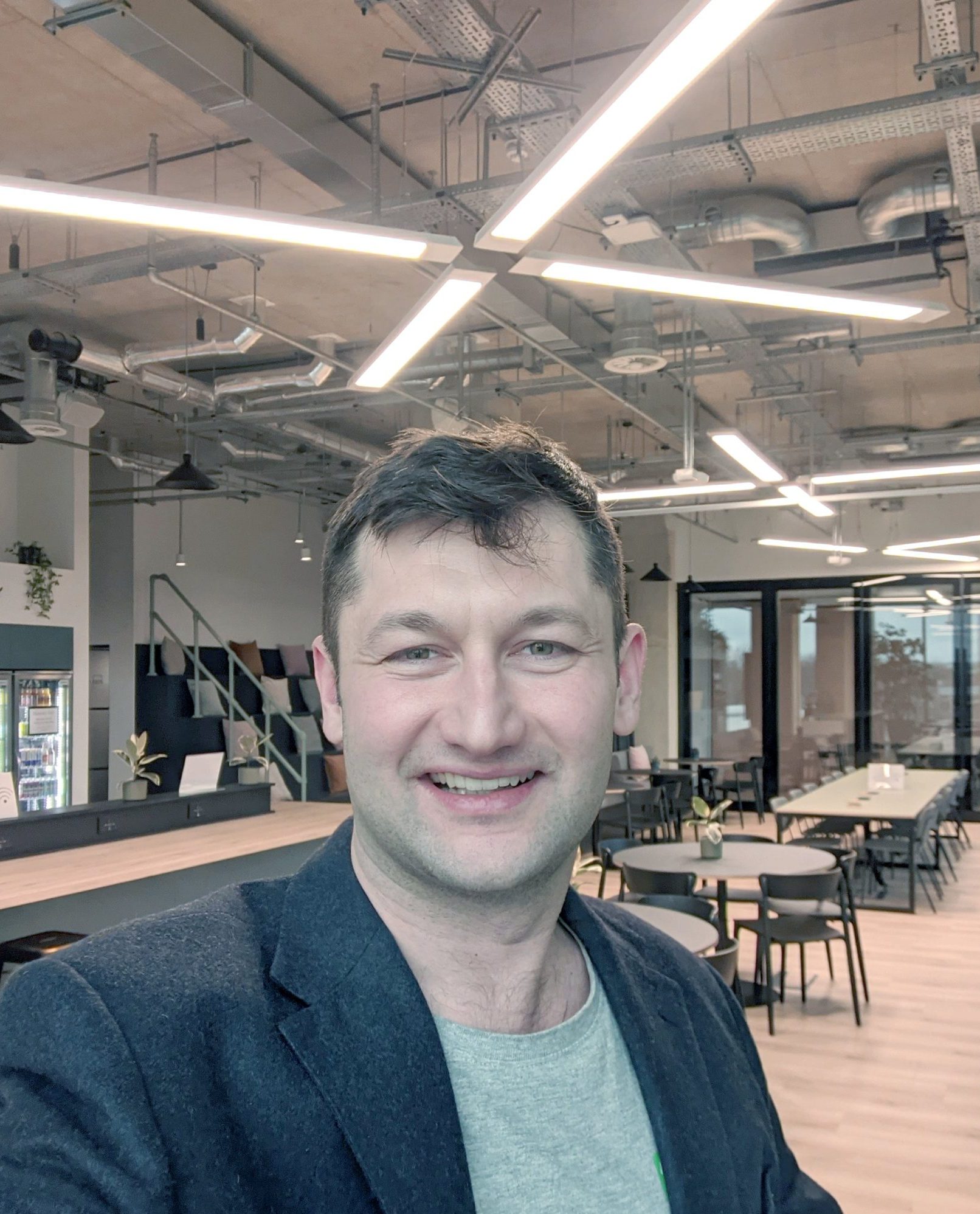
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython