Python Data Structure Exercises
Let’s check out some exercises that will help you understand structured data types in Python better, also usually referred to as composite data types as well.
Exercise 5-a
Let's create an empty list and print its type.
list() is a function that creates an empty list. You don’t need to put anything inside list() function to achieve this. The same thing can be achieved by typing brackets: []
type() function will tell you the class type of your variable.
gift_list = []
answer_1 = type(gift_list)
Exercise 5-b
Now, we will create an empty dictionary and print its type.
dict() is a function that creates an empty list. You don’t need to put anything inside dict() function to achieve this.
The same thing can be achieved by typing curly brackets: {}
type() function will tell you the class type of your variable.
grocery_items = {}
answer_2 = type(grocery_items)
Exercise 5-c
Then we will create an empty tuple and print its type.
Now let’s see some more exercises about converting data types.
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
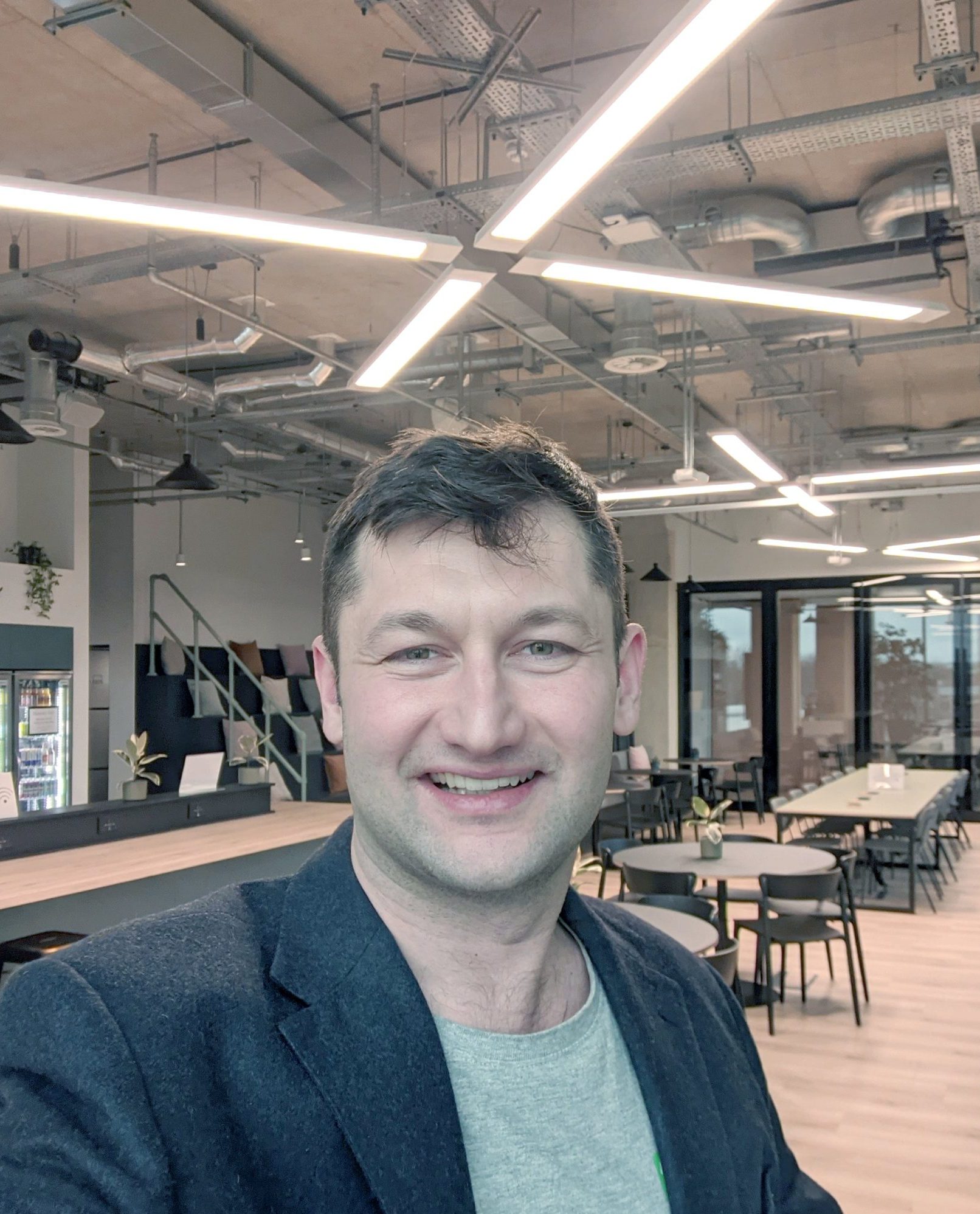
Founder of HolyPython