Python Operators Lesson with Examples
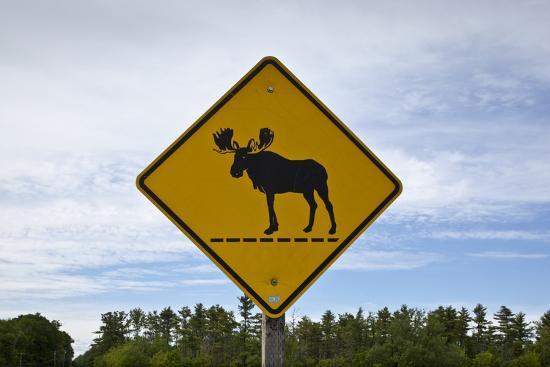
Simply put, Python operators are special signs. They are commonly used in programming languages and are also very important. Just as someone has to learn road signs before getting a driver’s license, a programmer has to learn operators to be able to carry out sophisticated operations in coding.
Just as road signs give meaning to driver-to-driver and driver-to-environment relations, programming operators give meaning to relations between variables and values in coding.
Estimated Time
15 mins
Skill Level
Beginner
Functions
na
Course Provider
Provided by HolyPython.com
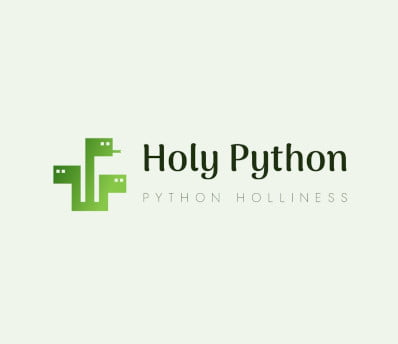
Used Where?
- Assignment operators: While assigning values to variables.
result = "win"
orlst_RE = ["wind", "solar", "wave power", "bioenergy"]
- Arithmetic operators: Mathematics! but really everything we do.
5+5
or100%7
or12//5
- Relational operators: When constructing a conditional statement
if a==5
orif result == "win"
- Membership operators: When checking if a group of values contains a sub-value (very convenient)
5 in lst
or"Jay" in sentence
or"a" not in wrd
Operators are very important signs used in programming. In python, there are different groups of operators that work based on similar logic but specific goals.
Although very fundamental and logical, operators might not be familiar to people who are not coming from a STEM background or our younger friends.
Since people from all walks of life whether they are entrepreneurs, economists, artists, doctors, nurses, musicians, students or retirees are starting to join programming literacy, and especially since more and more people choose to start with Python, we will try to explain operators with 2 logical common language analogies rather than technical lingo.
This tutorial will aim to help you learn Python Operators with examples and exercises and provide a referential guide to all the operators that are used in Python.
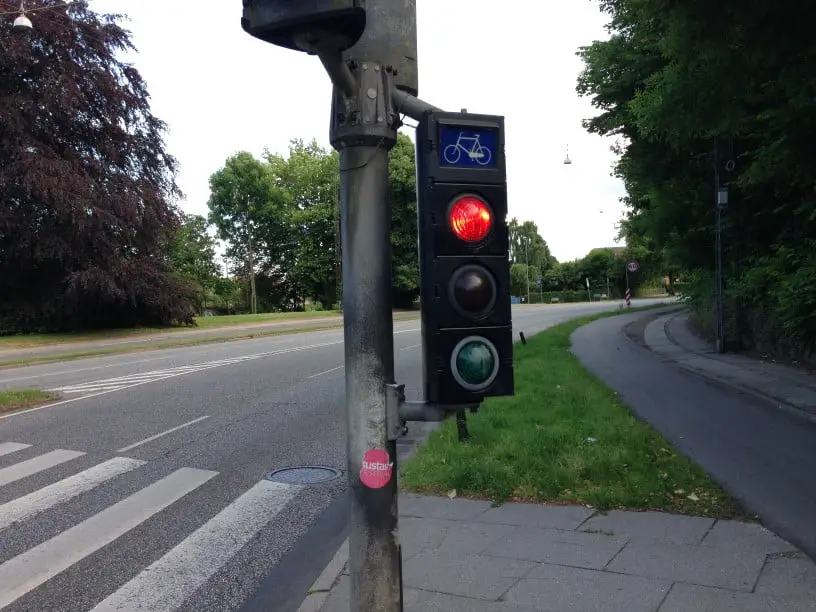
Blueprint of a Typical Programming Journey with Python
As a beginner coder someone learns these fundamental concepts:
- Variables (They contain value)
- Data Types (Self explanatory: integer, list, dictionary, string etc.)
- Syntax (Language specific, i.e.: Python uses quotes, spaces and indentation while some uses curly braces or star signs etc.)
- Operators (Topic of this article)
- Accessing Data, Altering Data (via different ways such as: slicing, functions, reassigning)
- Conditional Statements (if, elif, else)
- Loops
After that coder usually starts learning cool Python tricks:
- List Comprehension
- Dict Comprehension
- Lambda
- zip, map, filter, sorted, range, break & continue, try & except
Libraries and specialization:
Somewhere at this point or before, coder starts to mingle with libraries, domains and specialized tasks:
- games,
- finance,
- data science,
- visualization,
- machine learning,
- scripting,
- os,
- web requests,
- web scraping,
- APIs,
- turtle etc.
Python software:
Then there are also indirect, environment related topics like:
- IDEs,
- Notebooks,
- Text editors,
- Vim,
- Anaconda,
- Spyder,
- environment,
- Pycharm,
- Atom,
- Sublime,
- debugging tools,
- dependencies etc.
We recommend free and open source Anaconda Individual solution to all beginners as it makes everything much simpler with an all-in-one approach.
Now, we have a nice analogy, we summed up the Python learning process, let’s dive right into Python operators. They are probably best learned by examples and exercises anyway. So, if you skim this text and give the exercises a try you should have no problem learning Python operators.
----MOST IMPORTANT OPERATORS----
This is the most important section as we lay out the backbone of the Python operators. Most beginner and intermediate programmers can survive with these operators.
Assignment Operators (Used for variable assignments)
Let’s start with assignment operators since they are one of the first group of operators that are encountered by programming learners.
=
Variable goes on the left side of the operator (equal sign) and the value being assigned goes to the right of the equal sign.
Arithmetic Operators (Used for most basic mathematical operations)
+
Addition: adds values a + b
-
Subtraction: subtracts values a – b
*
Multiplication: multiplies values a * b
/
Division (float): divides the first value by the second value and returns value with decimals (float) a / b
//
Division (floor): same as above but return integers without decimals, rounds the result a // b
%
Modulus: gives the remainder of a division process a % b
**
Power : Raises the first value to the power of second value a ** b
Logical Operators (Used for logical statements)
and
Logical AND: Will returns True when both operands are True
or
Logical OR: Will return True if one of the operands is True
not
Logical NOT: Will return True if operand(s) is False
Relational or Comparison (Used for relational and logical statements)
>
Greater than: returns True if left side is greater than the right side x > y
<
Less than: returns True if left side is less than the right side x < y
==
Equal: returns True if left side is equal to the right side x == y
!=
Not equal: – returns True if left side is not equal to right side x != y
>=
Greater than or equal to: returns True if left side is greater than or equal to the right side x >= y
<=
Less than or equal to: returns True if left side is less than or equal to the right side x <= y
Cool Tricks
Here are some Python operators that are very cool and make coder’s life easier.
+=
This is a useful operator that makes adding values more practical. It supplements the “=” operator.
a+=b gives the same result with: a=a+b, basically it just saves you an extra character but it’s addictive.
-=
Same idea as above but for subtraction.
a-=b gives: a=a-b
Membership Operators (Used for most basic mathematical operations)
Not even sure this one is a trick. Membership Operators make life so much easier and they are very commonly used.
in
Will return True if an object is in another object
not in
Will return True if an object is not in another object
Advanced Details (Optional)
You can put aside some of the operators not to burn yourself out if you want. You can bookmark this article and refer back to it when you need a clarification about Python operators.
Identity Operators (Used for most basic mathematical operations)
is
Tells if two object are identical
is not
Tells if two object are not identical
More assignment operators (Optional)
+=
and -=
. Just used much less, especially in the beginning. *=
a*=b instead of a=a*b
/=
a/=b instead of a=a/b
%=
a%=b instead of a=a%b
//=
a//=b instead of a=a//b
**=
a**=b instead of a=a**b
&=
a&=b instead of a=a&b
|=
a|=b instead of a=a|b
^=
a^=b instead of a=a^b
>>=
a>>=b instead of a=a>>b
<<=
a <<= b instead of a= a << b
Bitwise Operators (Used for binary - bit operations) (Optional)
&
Bitwise AND a & b
|
Bitwise OR a | b
~
Bitwise NOT ~a
^
Bitwise XOR a ^ b
>>
Bitwise RIGHT SHIFT a>>
<<
Bitwise LEFT SHIFT a<<
Key Takeaway Points:
- Operators are critical to know in coding
- They can make your life easier
- You can leave aside some operators in the beginning of your learning period or as long as you don’t ened them(i.e.:Bitwise)
- Some operators are important but easily confused or forgotten
//
division (floor):**
power:%
modulus:!=
not equal:>=
greater than:<=
lower than:in
in:not in
not in:
- Another confusion is “=” vs “==”. Former is used for assignments while latter is used in conditional statements: i.e.:
If a == 0:
Python Operators Demonstration:
Here are some examples that can help you understand Python Operators and their use cases:
Example 1a: Assignment operator w/ string (=
)
greek_island = "Santorini"
Example 1b: Assignment operator w/ integer & tuple (+=
)
earth_age_bln = 4.4
universe_age_bln = 14
earth_age_bln += 0.1
print (earth_age_bln)
You can see free beginner Python lessons regarding data types, data structures, strings, integers etc. here.
4.5
Example 1c: Assignment operator w/ list (=
)
asia_wishlist = ["Bhutan", "Ha Long", "Laos", "Danxia", "Seoul", "Khao Sok", "Cebu", "Chiang Mai", "Ho Chi Minh"]
You can see a Python tutorial page about Python lists here.
Example 2a: Relational (comparison) operator (==
)
Let’s try to get the words (only with letters) in the text by trying different approaches.
msg = "life is beautiful"
if msg == "I love you":
print ("propose")
else:
print ("wait xP")
[‘wait xP’]
If you’d like to visit a free lesson about Conditional Statements if, elif, else
you can check out this page:
Example 2b: Relational (comparison) operator (>=
)
Let’s try to get the words (only with letters) in the text by trying different approaches.
"net_earnings" = 10.000.000
if net_earnings >= 100.000.000
print ("Large Cap")
else:
print ("Small Cap")
[‘Small Cap’]
Example 3a: Membership operator (in
)
lst = ["soccer", "swimming", "running", "skiing"]
if "rock climbing" not in lst:
print ("boo")
[‘boo’]
Example 3b: Membership operator (not in
)
web_data = ["techresearch and computervision"]
if "@" in web_data:
print ("e-mail address")
elif "0123456789" in web_data:
print ("phone number")
else:
print (not e-mail nor phone number)
[‘not e-mail nor phone number‘]
Example 4: Arithmetic operator (+, -, *, /, //, **, %
)
a = 10+20
b = 100 - 1
c = 50 / 7
d = 50 // 7
e = 10 % 8
f = 5 ** 2
print (a,b,c,d,e,f, sep="\n ")
30
99
7.142857142857143
7
4
25
If you’d like to see a Python lesson about print function and sep parameter used above, you can refer to the advanced tips section of this Python print lesson.
Good luck with the Intermediate Python Lessons!
Congratulations!
If you have followed Beginner Lessons in order, this is the last lesson of the beginner course. Congratulations for your courage, curiosity, effort and consistency. You can continue with the Intermediate Python Lessons.