Lesson 9: Python Strings
- ESTIMATED TIME: 25 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: .replace(), .lower(), .upper(), .startswith(), .endswith(), .capitalize(), .index(), .find(), .count(), type()
- 14 EXERCISES
- Strings are always represented in quotes in Python. They can be consisted of letters and numbers as well as symbols.
- Strings have similar characteristics to text and you can not do arithmetic operations with them.
- They make up with a variety of string methods what’s lacking in arithmetic operations.
Function 1: replace()
.replace() is a string method that can be used to change a sub-string in a string.
Used Where?
Strings are at the heart of data science and lots of other programming disciplines among other data types.
Often in programming process, we get long texts consisted of letters and numbers and other characters that we have to work with. These texts in string formats can come from web crawling, email data, financial reports, social media, books, documents, user input, sales reports, economic reports and many other sources.
It’s important for your to be comfortable dealing with strings so you can efficiently apply your coding skills on your data without getting confused or wasting too much energy. In this lesson we will explain lots of useful methods regarding strings.
- Python String Methods:
- .replace(): used to change substrings in a string
- .lower(): used to change a string’s characters to lower characters
- .upper(): used to change a string’s characters to capital letters
- .capitalize(): used to convert first character of the string to capital letter.
- .startswith(): used to check if the string starts with a specified letter
- .endswith(): used to check if the string ends with a specified letter
- .join(): a very useful string method used to join a specified string to the string
- .split(): another very useful method used to split a string based on the specified character(s)
- .strip(): used to strip a string of specified characters including empty spaces
- .index(): used to identify a character’s index in a string
- .find(): also used to find a character’s index in a string. find and index are very similar with a small difference which we will explain.
- .count(): used to count a sub-string in a string
- type(): function used to identify type of the data, can be used on strings.
Syntax do(s)
1) Print works with parenthesis.
correct usage is: print()
2) If you want to print a text, it will have to be in quotes: print("Hello World")
3) But if you want to print a variable this will have to be without brackets: print(mytext), more on this later.
Syntax don't(s)
1) print without parenthesis.
print "Hello, World!"
Although this will work with Python 2, it won't work with Python 3 and you'll get an error.
Example 1
You can use [] notation to call an element of a string.
>>> msg = “A giant leap for mankind”
>>> print(msg[0])
A
Example 2
.replace will take 2 parameters. First the character you’re searching, second, replacement character.
>>> msg = “A giant leap for mankind”
>>> msg = msg.replace(” “, “+”)
>>> print(msg)
‘A+giant+leap+for+mankind’
” ” represents the space character.
Example 3
Let’s try to replace a whole word.
>>> msg = “A giant leap for mankind”
>>> msg = msg.replace(“leap”, “step”)
>>> print(msg)
‘A giant step for mankind’
” ” represents space character.
Function 2: .lower()
.lower() string method makes all the characters in a string lowercase.
Example 4
>>> msg = “A giant leap for mankind”
>>> msg = msg.lower()
>>> print(msg)
‘a giant step for mankind’
Function 3: .upper()
.upper() string method makes all the characters in a string uppercase.
Example 5
>>> msg = “A giant leap for mankind”
>>> msg = msg.upper()
>>> print(msg)
‘A GIANT LEAP FOR MANKIND’
Function 4: .capitalize()
.capitalize() is a smart method that will make the first letter of a string uppercase (capital letter).
Example 6
>>> msg = “a giant leap for mankind”
>>> msg = msg.capitalize()
>>> print(msg)
“A giant leap for mankind”
Function 5: startswith()
.startswith() returns a bool type value: True or False. It questions whether the first character of a string is the one that’s being looked up.
Example 7
>>> msg = “a giant leap for mankind”
>>> a = msg.startswith(“A”)
>>> print(a)
False
Example 8
>>> msg = “a giant leap for mankind”
>>> a = msg.startswith(“a”)
>>> print(a)
True
Function 6: endswith()
Similar to .startswith() method, .endswith() method looks up if a value matches the last character of a string.
Example 9
>>> msg = “a giant leap for mankind”
>>> a = msg.endswith(“d”)
>>> print(a)
True
Example 10
>>> msg = “a giant leap for mankind”
>>> a = msg.endswith(“.”)
>>> print(a)
False
Function 7: .index()
Just like lists and tuples, strings also have index orders.
.index() method is useful to find out the index of first matching substring.
Example 11
>>> msg = “a giant leap for mankind”
>>> a = msg.index(“a”)
>>> print(a)
0
Example 12
>>> msg = “a giant leap for mankind”
>>> a = msg.index(“d”)
>>> print(a)
23
Function 8: .find()
.find() method does the exact same thing .index() method does except one difference.
When .find() method can’t find a value it returns, -1, but when .index() method can’t find the value it raises a ValueError.
It can be handy if your program needs to avoid this error.
Example 13
>>> msg = “a giant leap for mankind”
>>> a = msg.find(“d”)
>>> print(a)
23
Example 14
>>> msg = “a giant leap for mankind”
>>> a = msg.find(“X”)
>>> print(a)
-1
Function 8: .count()
.count() method can be used to identify how many times a value occurs in a string.
Example 15
>>> msg = “a giant leap for mankind”
>>> a = msg.count(“i”)
>>> print(a)
2
Function 9: type()
type() function is useful to tell the type of your data including strings among many others.
Example 16
>>> msg = “a giant leap for mankind”
>>> a = type(msg)
>>> print(a)
<class ‘str’>
Tips
1-
Function 10: len()
len() function will return the length of your string.
Example 17
>>> msg = “a giant leap for mankind”
>>> a = len(msg)
>>> print(a)
24
Advanced Concepts (Optional)
1- .split(), .strip() and .join() are incredibly powerful string methods that we will focus individually in the upcoming lessons.
They are very commonly utilized and can be very handy in many text related tasks.
.split() splits a string based on a character and returns a list of pieces that are split.
.join() joins a list of elements to a string and it can use a specified character for the joining process.
.strip strips a string of specified characters. It is particularly useful for stripping whitespace from the start and end of a string.
Example 18
>>> msg = “a giant leap for mankind”
>>> a = msg.split(” “)
>>> print(a)
[‘a’, ‘giant’, ‘leap’, ‘for’, ‘mankind’]
Example 19
>>> msg = ” a giant leap for mankind “
>>> a = msg.strip()
>>> print(a)
“a giant leap for mankind”
Example 20
If you notice, .join() is slightly different than other methods that character that will be used for joining comes in front of it and the list name comes inside its parenthesis. This can be confused if you don’t pay attention.
>>> lst = [‘a’, ‘giant’, ‘leap’, ‘for’, ‘mankind’]
>>> msg = “!!!”.join(lst)
>>> print(msg)
‘a!!!giant!!!leap!!!for!!!mankind’
Next Lesson: Python len() Function
“Have you installed Python yet?”
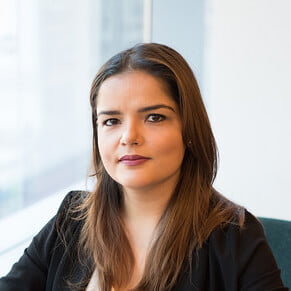