Lesson 16: Defining Functions
- ESTIMATED TIME: 25 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: n/a
- 9 EXERCISES
So far we have seen many different built-in Python functions. To give some examples: len, print, int, sum, max, min, str, list are all built-in Python functions that can be called and they do what they are supposed to. But these functions also have a source code very similar to the structure that will be outlined in this Python lesson.
These are nice and useful functions that are already defined in Python. They are very practical to use. You don’t need to do anything other than taking them and using them and they will do the job.
However, it’s also very useful to know how to define your own functions.
Defining a function is pretty simple and straightforward in Python but there are a few points you should keep in mind. We will explain all of those clearly here in this lesson.
Take a look. This is what gets executed when we run a code with input function. It makes use of class structure as well as function definition. As you can see input is a function with one parameter: prompt, which is assigned to an empty string by default.
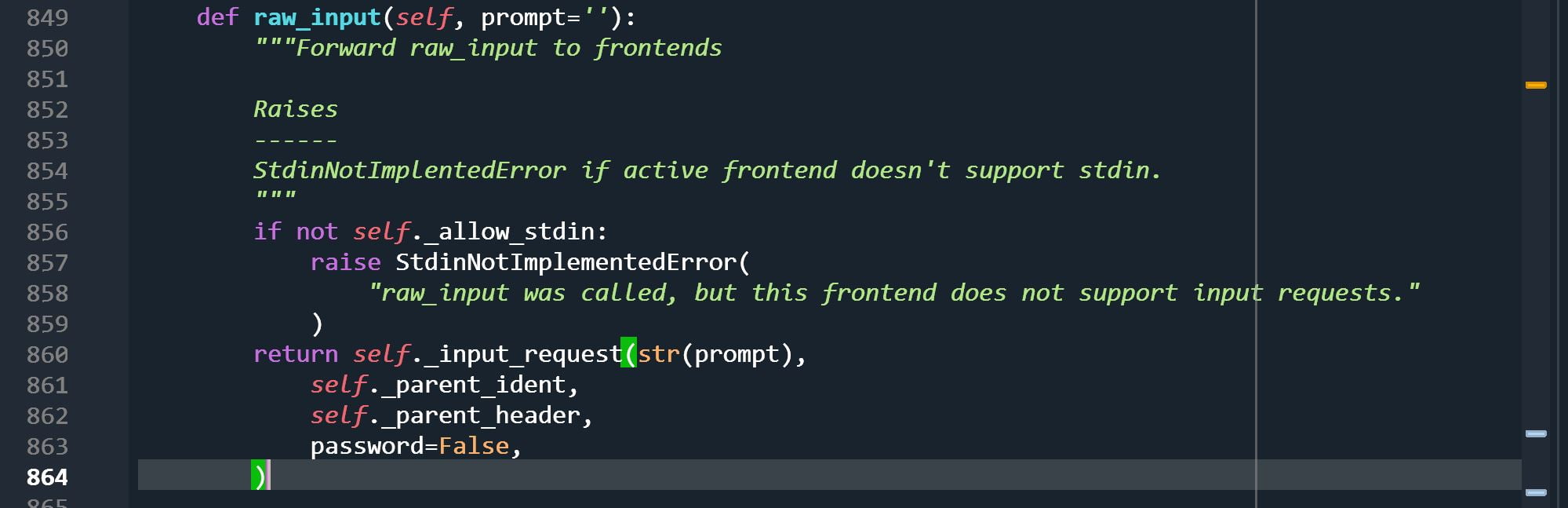
It’s beyond the scope of this lesson but input function calls another function named: _input_request and this is a great example that you can also call other functions (built-in as well as user-defined) from inside your user defined function.
Let’s start with baby steps and demystify user defined functions in Python so you can also create your own functions.
Used Where?
Coders usually have a practical mindset and don’t like to repeat similar tasks. By defining your own functions, you can automate a similar task instead of typing the whole function again and again.
After you define your function you can just call its name and execute it over and over for different applications and parameters. In programming functions are used everywhere. Here are some example use cases:
- In data science to eliminate repetitive tasks. (opening/reading/cleaning data)
- In finance to create custom functions which execute specific tasks. (Accessing data or sending reports)
- In GUI applications to execute specific user interface inputs such as pressing a button, cursor click, pinching, swapping etc.
- In programming languages (such as Python, Rust, Go, JavaScript, R etc.) to create built-in functions from most repetitive and anticipated programming language tasks.
- In development environment to tidy up the code and make it more readable and more accessible.
- In libraries to create class structures which can consist of multiple functions.
- To call other functions from inside a function.
Syntax do(s)
1) to define a function
- start with def,
- choose a wise name for your function,
- make parenthesis,
- and inside your parenthesis include any parameter you might need (optional),
- then use a colon at the end of the line,
- in the next lines define your function, make sure they are indented (using tab key)For example: def no_adder(x): print("No ", x)
Syntax don't(s)
1) Don't confuse the use of print() function with return statement. (This will be explained in great detail in this lesson.)
2) Don't forget to use a colon (:) at the end of your first line that starts with def
3) After your first line that starts with def, don't forget to have and indentation
We will see some Python examples that can help understand user-defined functions in Python and get you warmed up for user-defined function exercises in Python.
Function : print
We’ll use built-in print function inside a very simple user defined Python function to start with a very simple example.
Example 1: Creating a simple user function in Python
Just take a look at the function we define below, you can quickly observe some of the points we previously mentioned.
def helloworld():
print("Hello World!")
helloworld()
“Hello World!”
Python User defined function in 5 simple steps:
- Our function defining starts with def
- Then an appropriate name for the function that’s not reserved for Python keywords (print, int, None, def, len, lower etc. would not be good names as they are examples to Python keywords)
- Then parenthesis (parameters inside optionally)
- Then colon (:)
- In the line below, indentation and our function.
Example 2a: Calling a function with parameter values.
Let’s define another function that divides any number by 100.
- Percent is a risky name, it might be a keyword, so I will be creative.
- Also I will use a parameter this time as I want to use this parameter in my function so that it can work for different values of the parameter.
- Then I will call it for 5000 and see if it works.
def per_hundred(x)
print(x/100)
per_hundred(5000)
50
Perfect! Our function is working well.
Do you see the difference between first example and this one? First example didn’t have any parameters. Its output is independent from any input (through parameters). In fact it doesn’t require any inputs.
In this example though, you will have to specify the parameter since its value is unknown otherwise function can’t be called properly.
Also, instead of x you could call it any other variable name such as desired_value or whatever.
Example 2b: Calling a function and assigning it to a variable with return statement
Now, let’s see what doesn’t work here first.
I want to assign the result to a variable so I can use it later.
def per_hundred(x)
print(x/100)
my_answer = per_hundred(5000)
print (my_answer)
None
It didn’t work. The reason is simple but can be a great source of confusion for beginners. When we use the print() function, our function is programmed to just print and end without returning any result. Returning a value and printing are seemingly similar but very different things. In the next session you can learn how to properly use the return statement instead of print() function (or with the print() function as well.)
Solution to this problem is return statement.
Return Statement
Unless you use return in your function it will not return any value. What does this exactly mean?
Often times, you want your function to do more than just printing on the display for the user, if you’re not using the return command your function will run and end, but it will not be equal to any value.
i.e.:
def plus_two(x):
print(x+2)
Above function might print the value you intended to the display. but it runs and disappears. For instance:
When you try to assign it to a variable it won’t return any value.
my_result = plus_two(2)
Normally, you might expect my_result to equal 4. But it will return None object due to lack of return statement.
You might think, why do I need to return a value? Why can’t I just print the result and be done?
The answer to that is simple, you might not want your results to disappear and you might to assign your results to variables and use them elsewhere in your program.
For instance:
You might want to assign the same function with 3 different parameters to 3 different variables as below:
x = plus_two(2)
y = plus_two(3)
z = plus_two(4)
With correct use of return statement, your variables x,y,z will be assigned to 4, 5 and 6.
If you forget to use return statement in your function, or just use the print() function, your variables will be assigned to: None, None and None.
Example 3: User defined function (syntax error)
Now, let’s see another very common mistakes while defining functions:
def per_hundred(x)
return x/100
my_answer = per_hundred(5000)
print (my_answer)
Syntax Error: invalid syntax
As the first line that starts with def is missing a colon(:) in the end, compiler throws a syntax error.
- colon is where it should be
- second line is indented properly
- return statement is used properly
Example 4: User defined function
And finally a proper version of our example that works.
def per_hundred(x):
return x/100
my_answer = per_hundred(5000)
print (my_answer)
50
- colon is where it should be
- second line is indented properly
- return statement is used properly
Tips
1- At this stage, understanding print() vs return is very important.
Although it is straightforward this new concept can be confusing to newcomers.
If you include a print() function this might trick you to believe that it is the value your function returns, however this is not the case. When you use print function in a function, it simply prints something in the console and does nothing more than that.
If you want your function to return a value when it is called later (for it to equal to something after it runs and ends) you need to use return statement.
print() will only print a message but this is not the same thing as returning a value. Print function’s action shows an output to the screen and vanishes in air. So, it doesn’t mean your function will equal to the value you are printing after it runs. For that you need to use return statement.
It will be more clear after a few examples:
def plus_two(x):
print (x+2)
a = plus_two(2)
print(a)
None
As you can see above your program will return None, because we didn’t use the return statement.
Let’s see another example where function is properly defined.
Example 5: Function with return statement
- You can define functions with optional parameters as well. An optional parameter is a parameter that you don’t have to use because it would already have a pre-assigned value.
def plus_two(x):
print (x+2)
return (x+2)
func = plus_two(2)
print(func)
4
Here we go, our function is properly defined. And when it’s tested with number 2 and assigned to the variable func, it functions properly. There are some important lessons here:
- You could exclude the print(x+2) line. It is only for showing and it doesn’t affect the actual function result.
- return is the final line in a function. As soon as return is executed function will be terminated so technically, you can’t write another line after return as the function finalizes at the return line.
Example 6: User defined function with optional parameters
You can also assign multiple variables to multiple function outputs in one line as below:
def plus_two(x):
print (x+2)
return (x+2)
a, b = plus_two(2), plus_two(6)
print(a, b)
4, 8
- As long as assignee variable amount matches the returned values of functions one-line assignment work without any issues.
Advanced Concepts (Optional)
- While defining your function, you can specify your parameters so that they are optional. This can be done by passing a value to the function’s parameter during definition.
- Optional parameter can be overwritten by specifying it again later while calling the function.
- You can also call other functions from inside your function. This brings incredible flexibility and structure advantages to the code. Check out these examples:
Example 7: User defined function with optional parameters
- You can define functions with optional parameters as well. An optional parameter is a parameter that you don’t have to use because it would already have a pre-assigned value.
def fave_color(name, color="azure"):
msg = name+"'s favorite color is: "+color
return msg
print(fave_color("Pete"))
Pete’s favorite color is: azure
- In this case color is an optional parameter. It means even if you don’t specify color parameter function will execute properly as long as you assign an appropriate value to the default parameter: name.
Example 8: User defined function with optional parameters
- You can still overwrite an optional parameter with a suitable value. In this case another string.
def fave_color(name, color="azure"):
msg = name+"'s favorite color is: "+color
return msg
print(fave_color(name="Olivia", color="violet"))
Olivia’s favorite color is: violet
Example 9: Calling another function from your function (Optional)
- Suppose we invented a new regenerative medicine that more doubles humans’ life expectancy and brings new averages to 200 years.
- But there is a caveat if candidates are smokers efficiency is reduced by half and life expectancy is actually 150. Let’s see some simple demonstration of using multiple user-defined functions in harmony with simple calculations.
- This example also is a great opportunity to practice multiple important Python concepts we have covered so far namely: string methods, data type conversion to string, data structures, bool and string data types in Python, variable assignments, even Python Operators and gently introduces a very fundamental topic ahead of the intermediate Python lessons: conditional statements (if, elif, else).
- At this point, If you feel competent enough to continue with the example by without checking (or quickly checking) conditional statements then great. Otherwise you can always revisit this example after you gain more knowledge about conditional statements in Python.
#Function life expectancy calculation
def new_life (name, age):
if smoker(name) == True:
life_exp = 150
else:
life_exp = 200
life_remaining = life_exp - age
msg = "Hi "+name+"! Your new expected remaining life is: "+str(life_remaining)+" years."
return msg
#Function for smoker database check
def smoker (name='', smoke=False):
if name.capitalize() in ["Jane", "Zack", "Melissa"]:
smoke = True
return smoke
#Execution
print(new_life("jane", 30))
Hi jane! Your new expected remaining life is: 120 years.
- Not bad for a smoker!
- Message output doesn’t capitalize user’s name. Can you fix it?
So, we can wrap up our Python User Defined Functions lesson. I hope you enjoyed it and found it useful. I think defining functions is a milestone in Python and programming in general. It gives you more room for creative applications and more professional implementations.
Also, it can be a bit confusing as it’s a leap from using simple built-in functions. At this point, you are becoming a real deal creator. Of course, intellectual pursuits never end, but still.
If you need a break or repeat some concepts up to this point, take your time, give yourself necessary time to digest these very important programming concepts. If you already feel you got it all then pick up the pace and continue practicing / exploring new lessons. It will be different for everyone’s individual case.
If you’re enjoying our Python tutorials please share our pages with friends and followers! Thank you very much.
Next Lesson: Python Slicing Notation
“Have you installed Python yet?”
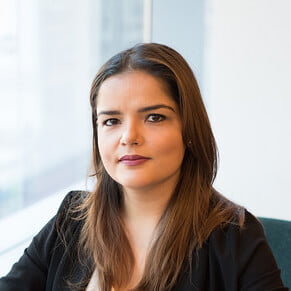