Lesson 15: Error Handling
- ESTIMATED TIME: 9 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: n/a
- 7 EXERCISES
PART I: In part one we will look at Built-in Python errors that you may encounter. Getting familiar with these can help you understand the issue fast and feel more confident. Here are some of the most common ones which we will look closely:
- NameError
- SyntaxError
- TypeError
- IndexError
- KeyError
- AttributeError
PART II: In part two, we will look at the errors in a more general programming sense. It can help your programming skills to understand that not all the errors are caught by the compiler all the time and some of them have to do with results you’d like to achieve.
- Syntax Error
- Semantic Error
- Runtime Error
Function: N/A
No new functions will be introduced in this lesson.
Used Where?
Syntax do(s)
1)
Syntax don't(s)
1)
Part I
NameError
You’ll get a NameError when an object can’t be found in Python.
Example 1
>>> str = “Hello World!”
>>> print(monkey)
NameError:…
SyntaxError
You’ll get a SyntaxError when you make a syntax error in Python. It can be a missing quote or parenthesis.
Example 2
>>> str = “Hello World!
>>> print(str)
SyntaxError:…
*(Missing quote)
TypeError
You’ll get a TypeError when you apply an operation or function to the wrong type of data, such as applying arithmetic operations to strings.
Example 3
>>> str = “Hello World!”
>>> print(str+5)
TypeError:…
IndexError
You’ll get an IndexError when you try to reach an index outside the limits of your data.
Example 4
>>> str = “Hello World!”
>>> print(str[25])
IndexError:…
KeyError
KeyError is like IndexError for dictionaries. If you try to reach a key that’s not included in your dictionary, you’ll get a KeyError
Example 5
>>> dict = {“Apple”: 5, “Mango”: 7}
>>> print (dict[“Lemon”])
KeyError:…
AttributeError
Attribute error occurs when you try to use an attribute or method that doesn’t apply to the specific data you’re working on. For instance, trying to apply .reverse() method on a string.
Example 6
>>> data = 11.79011
>>> data.capitalize()
AttributeError:…
ValueError
Value error occurs when you apply a function to a data type correctly but the content is not suitable for that operation. For example, you can apply int() to a string of numbers such as:
int(“9999”)
but you can’t convert letters to integers so following won’t work:
int(“kittycat”)
Example 7
>>> str = “11A”
>>> int(str)
ValueError:…
Tips
1- If you try to intentionally cause Python Builtin Errors a few times you won’t easily confuse them nor forget them in the future. Exercises 15 are specifically design in that way for this lesson.
2- Part II will be regarding a general programming discussion rather than a Python specific lesson.
Part II
Syntax Error
- When you get a Syntax Error this means you are making a grammer mistake in Python. And Python can’t understand and can’t compile your code. Usually it’s easy to fix as Syntax is definitive and you will just need to fix your mistake in the related line with the mistake.
- For instance:
- you’re typing an extra character in a wrong way,
- you’re typing a Python keyword wrong,
- parenthesis mistakes are also very common,
- you might be forgetting or mistyping quotation marks,
- your line might need indentation (usually in if statements),
- you might be forgetting a colon (common with if statements, defining functions, building classes etc.)
- sometimes an extra space will cause a syntax error.
As you can see, a tiny mistake can cause your program to throw out a syntax error and this can be very frustrating for beginner programmers.
It gets better as you code as there are limited amount of syntax errors and they usually resemble each other.
Initially keep your cool, you’re probably not seeing a mistake because your eyes are not trained yet.
Unlike normal language writing, programming languages are less forgiving in this sense and one tiny mistake will cause your whole program to malfunction.
The good thing is syntax error tells you the line of the error and you will get used to the syntax of a language rather quickly.
Example 8
>>> lst = [1,2,3,4
SyntaxError
*(Missing bracket)
Semantic Error
Also called Logical Errors, semantic errors are an issue of meaning. It’s not a grammar issue, or in programming lingo, syntax is fine and program compiles but the results are not what we intended to have.
- Your program will run successfully but the result will be anywhere between slightly off to completely wrong.
- For instance:
- Forgetting to divide a percentage result with 100
- Wrong result caused by wrong order of arithmetic operators
Example 9
>>> population = 23777000
>>> live_births = 570000
>>> birth_rate_per_thousand = live_births / population
>>> print(birth_rate_per_thousand )
0.0239
Although program runs successfully, we forgot to multiply the result by 1000 since the rate is per thousand. So the answer is wrong and confusing.
Runtime Error
Finally, runtime errors are errors that happen during the program’s runtime. It means, program compiled and started executing without any problems, meaning there is no syntax error. However, during the runtime something is not quite right.
For instance:
- It can be caused by infinite loops (As we will see during if statements later.)
- Trying to divide a number by zero (another infinity issue.
Example 10
>>> population = 23777000
>>> space_incident = 0
>>> space_incident_rate = population /
Your program is likely to freeze or throw a runtime error due to division by zero.
Advanced Concepts (Optional)
1- Here is a full list of Python’s built-in Errors.
AssertionError: | Raised when the assert statement fails. |
EOFError: | Raised when the input() function hits the end-of-file condition. |
FloatingPointError: | Raised when a floating point operation fails. |
GeneratorExit: | Raised when a generator’s close() method is called. |
ImportError: | Raised when the imported module is not found. |
KeyboardInterrupt: | Raised when the user hits the interrupt key (Ctrl+c or delete). |
MemoryError: | Raised when an operation runs out of memory. |
NotImplementedError: | Raised by abstract methods. |
OSError: | Raised when a system operation causes a system-related error. |
OverflowError: | Raised when the result of an arithmetic operation is too large to be represented. |
ReferenceError: | Raised when a weak reference proxy is used to access a garbage collected referent. |
RuntimeError: | Raised when an error does not fall under any other category. |
StopIteration: | Raised by the next() function to indicate that there is no further item to be returned by the iterator. |
IndentationError: | Raised when there is an incorrect indentation. |
TabError: | Raised when the indentation consists of inconsistent tabs and spaces. |
SystemError: | Raised when the interpreter detects internal error. |
SystemExit: | Raised by the sys.exit() function. |
UnboundLocalError: | Raised when a reference is made to a local variable in a function or method, but no value has been bound to that variable. |
UnicodeError: | Raised when a Unicode-related encoding or decoding error occurs. |
UnicodeEncodeError | Raised when a Unicode-related error occurs during encoding. |
UnicodeDecodeError | Raised when a Unicode-related error occurs during decoding. |
UnicodeTranslateError | Raised when a Unicode-related error occurs during translation. |
ZeroDivisionError | Raised when the second operand of a division or module operation is zero. |
“Have you installed Python yet?”
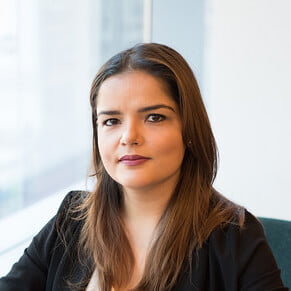