Lesson 14: Python range() function
- ESTIMATED TIME: 8 mins.
- DIFFICULTY: Beginner
- FUNCTIONS: range()
- 5 EXERCISES
There are different ways of using range() function.
range() function can accept 3 parameters as below:
range(start, stop, step)
However, if you type only one number inside range() function, it will smartly assume start is 0 (zero), step is 1 and stop is the number you’ve specified. So,
- You can specify only one number inside range’s parenthesis:
- i.e.: range(5)
- In this case, it will return a range of numbers from 0 to 5 (5 exclusive) increasing by 1 as below:
- 0, 1, 2, 3, 4
- You can specify two numbers inside range’s parenthesis:
- i.e.: range(2, 8)
- In this case, function will return a range of numbers from 2 to 8 (8 exclusive) increasing by 1 as below:
- 2, 3, 4, 5, 6, 7</n>
- You can specify all three numbers inside range’s parenthesis:
- i.e.: range(2, 13, 3)
- In this case, function will return a range of numbers from 2 to 13 (13 exclusive) increasing by 3 at each step as below:
- 2, 5, 8, 11
Used Where?
- Such a range can be used in iteration loops or any case where you might need a sequence of numbers.
- As a bonus you can also create a decremental sequence very easily with a negative step number in range() function. We will take a look at all of these points in this lesson.
Syntax do(s)
1) Just pass range start, range end and step parameters to the function.
Syntax don't(s)
1) Don't forget to construct a list or tuple with your range object if you'd like it to be readable by humans. Otherwise range object is more computation friendly.
Function 1: range()
range() function creates a sequence of numbers in a specified range.
Example 1
>>> rng = range(5)
>>> print(rng)
range(0,5,1)
Range above is consisted of 0,1,2,3,4
Example 2
>>> my_rng = range(0,5,2)
>>> print(my_rng)
range(0,5,2)
Range above is consisted of 0,2,4
Tips
1- Range function will return a range type. This is just another data type in Python and no need to be confused about it. However, it can slightly affect the way you work with range function.
So, in Python, when you use the range function instead of getting a list of numbers in that range you simply get the parameters for the range instead.
2- Here is a good tip, if you’d like to make a list of a range. you can use the list() function we learned before and use the range function inside it and you will have a list of numbers.
Here are some examples.
Example 3
First let’s look at an example where we identify the type of a variable assigned to range function.
>>> a = range(0, 15, 3)
>>> print(type(a))
<class ‘range’>
Example 4
Now let’s try to print the same variable and see what happens.
>>> a = range(0, 15, 3)
>>> print(a)
range(0,15,3)
As you can see above instead of getting a list of numbers we would get a range object.
Example 5
Now let’s overcome this situation with the list() function we’ve learned before.
>>> a = range(0, 15, 3)
>>> b = list(a)
>>> print(b)
[0,3,6,9,12]
Advanced Concepts (Optional)
1- Range function can also be used in descending order. All you have to do is use a negative step parameter. Let’s see an example.
Example 6
Let’s use a negative step to create a decremental (just a fancy way of saying decreasing) range, convert it to a list and print it practically in 2 lines of code.
>>> a = range(5, -5, -3)
>>> print(list(a))
[5, 2, -1, -4]
Next Lesson: Error Handling in Python
“Have you installed Python yet?”
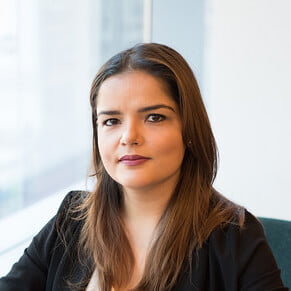
SANDRA FILES