Python map function (Applies function to each item in sequence)
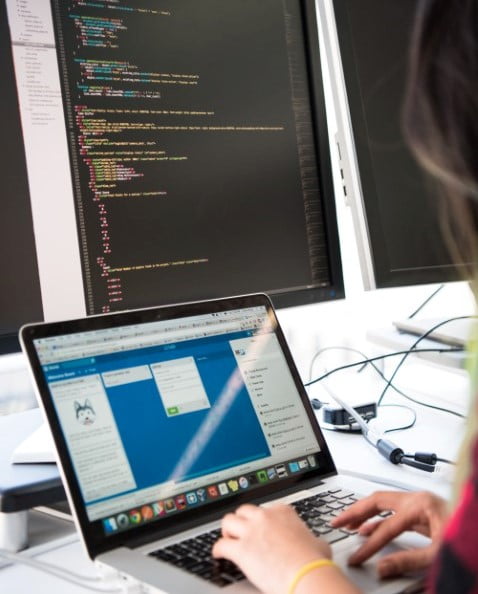
Map function is used to apply a function to each of the items in an iterable.
This is particularly useful when you have one of the sequence objects of Python such as dictionaries, tuples, strings, lists, range object etc.
We also have filter()
function which is like a sister function to map()
By combining the two, you can first filter the items in a sequence (say a Python list) and then apply map to only those that pass the filter. But we’re getting ahead of ourselves. You’ll see the Python examples below.
You can also do it the other way, map a function to each item in a list and then do it inside a filter function so that only results that pass the filter will be shown.
Estimated Time
13 mins
Skill Level
Intermediate
Functions
map ()
Course Provider
Provided by HolyPython.com
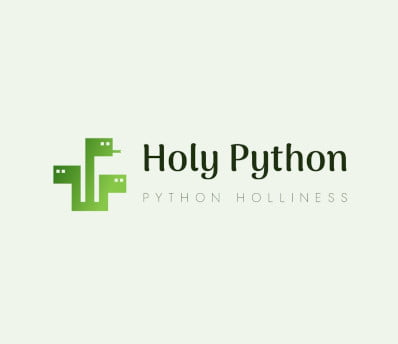
Used Where?
map()
function is used to apply a function to each of their elements.
When you have an iterable data (a list, tuple, string, dictionary etc.),filter()
function,map()
function can be used together and they both become more useful. This gives map more control on which values to apply the function to.- This combination makes both functions more powerful. However, in Python, list comprehension and dict comprehension can sometimes make map and filter functions obselete.
Notes before map examples:
* It’s good to know different Python objects when working with iterables and functions (list comprehension, dict comprehension, map, filter, zip etc.) as these work in harmony.
Usually in programming, there are multiple ways to achieve a result, and particularly so with Python. So, it’s important to have good command of the syntax and functions especially in the beginning of a journey of programming language learning and practice a lot. But there are many concepts so don’t overwhelm yourself either. One step at a time, you will be an amazing Python programmer.
Below you will find some Python map
function examples we have created for you. These Python examples can hopefully make these concepts more clear in a demonstrative way.
** Most examples will make use of list
function for data conversion. This is because map function returns a map object (which may look weird). It’s an efficient memory address representation. list
function will be used to convert it to an actual list in most of the examples.
Syntax do(s)
1) inside map
function's parenthesis type your function followed by a comma
2) then the name of a list, tuple, dictionary or string
i.e.: map(f, iterable)
Syntax don't(s)
1) Don't print map
function directly, you will only get a unique memory address
2) Don't forget to put your map function inside a list()
function if you'd like to create meaningful results that are readable by the user.
Example 1: map() with len
- You can pass any function that makes sense to your
map()
function, it doesn’t have to be in the form of . Here is an example where function is defined conventionally and then passed inmap()
function as an argument.
lst = ["Greenland", "Japan", "Taiwan", "Botswana", "Bhutan", "Tuvalu"]
lst2 = map(len, lst)
print(list(lst2))
[9, 5, 6, 8, 6, 6]
Example 2: map() on integers (and floats)
- Map doesn’t only work with strings though. You can apply any iterable function to any suitable Python object. Let’s see something cool with integers and floats.
- Let’s say you have a dataset of distance variances. Although distances are negative and positive you are interested in total absolute sum to see the total deviation. Check out how easy it is in Python with map function:
lst = [100,-3.5, 5, -0.7, -9, -0.003]
new_lst = list(map(abs, lst))
print(new_lst)
[100, 3.5, 5, 0.7, 9, 0.003]
Example 3: map() for data type conversion
- Here we have a bunch of floats. Imagine it’s a list with 100 values. The CFO is picky, it’s understandable because the guy is so busy all the time. He wants to see these numbers as integers. Can you do it?
lst = [87.9983729183943, 88.27381223193, 88.01283119001, 90.3166247903554, 89.94283229101, 100.00000000001]
new_lst = map(int, lst)
print(list(new_lst))
[87, 88, 88, 90, 89, 100]
Example 4: map() with reversed
- Here is another cool example.
reversed
function can be used to reverse strings in Python, it can also be used as an iterating function combined with map function. But there is a little trick. This Python example is a great demonstration of multiple programming techniques.
lst = ["Greenland", "Japan", "Taiwan", "Botswana", "Bhutan", "Tuvalu"]
new_lst = map(reversed, lst)
print(list(new_lst))
[reversed object at 0x000001C05F984F48,
reversed object at 0x000001C05F984588, reversed object at 0x000001C05F984408,
reversed object at 0x000001C05F984D08, reversed object at 0x000001C05F984E08,
reversed object at 0x000001C05F98F548]
- Oops! So, what is this? No need to panic it’s just a computational way of storing and presenting data. It’s not very presentable to the human eye. But computers are perfectly cool with it.
- Let’s make it so that humans can also read it.
lst = ["Greenland", "Japan", "Taiwan", "Botswana", "Bhutan", "Tuvalu"]
new_lst = map(reversed, lst)
for i in new_lst:
print("".join(i))
dnalneerG
napaJ
nawiaT
anawstoB
natuhB
ulavuT
- Here we go! In this little exercise we have the opportunity to practice many Python concepts like:
Example 5: map() with reversed V2
- Here is a smart solution. Instead of having to deal with Python loops, you can just apply map twice! Double map it like below:
lst = ["Greenland", "Japan", "Taiwan", "Botswana", "Bhutan", "Tuvalu"]
new_lst = map("".join ,map(reversed, lst))
print(new_lst)
dnalneerG
napaJ
nawiaT
anawstoB
natuhB
ulavuT
- This is how Python can get fancy. Quick smart ways to apply programming solutions is most likely what got so many people hooked with Python. It’s fun and useful. Some people call it Pythonic way to do things.
- It comes with time though so as you learn always remember you will get more comfortable with time and don’t stress. Practicing is a guaranteed way to long-term mastery. That’s why we have high quality interactive Python exercises for you.
Example 6: map() with lambda
- Oh no! I told Suzan to write everything in lower case. And the actual list has 10 thousand emails
- Can you convert the list to lower case real quick? You can use
map()
function. - .lower() method will be super handy for this but it’s a string method and not a function by itself. It was about time we start writing our own functions!
lst = ["Suzie@GMAIL.com", "haru@Baidu.com", "LIUWEI@qq.com", "ADAM@Yahoo.COM"]
fnc = lambda x: x.lower()
new_lst = list(map(fnc, lst))
print(new_lst)
[‘suzie@gmail.com’, ‘haru@baidu.com’, ‘liuwei@qq.com’, ‘adam@yahoo.com’]
- Lambda is a very elegant way of creating simple, one line user-defined functions. Quick and dirty? More like quick and beautiful 🙌
- In case you’d like to explore lambda further > Python lesson about Lambda
Tips
* You can combine two maps or a filter and a map for more sophisticated operations.
** Some functions return iterable objects that are hard to read for humans. These structures are extremely efficient and when you print them you just see a memory address like :
Simply convert these objects to suitable Python data structure et voila! It’s readable to humans.
Recommended Reads:
Example 7: filter with map and lambda
- Let’s say you are a quantitative analyst in finance. There are some important price returns in a list but they put the percentage values in directly. Using
map()
function and lambda, you can divide each value with 100.
lst = [2.54, 4.0, 3.0, 9.95, 5.4]
new_lst = map(lambda x: x/100, lst)
print(list(new_lst))
[0.0254, 0.04, 0.03, 0.09949999999999999, 0.054000000000000006]
- Oh! That’s great, but so hard to read. Check out the next example.
Example 8: filter with format
Let’s say you are a quantitative analyst in finance. There are some important price returns in a list but they put the percentage values in there directly. 0.03 instead looks like 3. This will not fly from the execs!
- Using
.format()
is a very cool method to change the presentation format of data in Python. It can be applied to iterables. It looks a bit weird at first but you get used to it. - For example “{:,.2f}”.format will convert numbers to two float decimals.
- Similarly, “{:,.2%}”.format will return percentage format with 2 decimals. You can see more about Python format method in this lesson.
Check out this example.
lst = [2.54, 4.0, 3.0, 9.95, 5.4]
new_lst1 = map("{:,.2f}".format, map(lambda x: x/100, lst))
new_lst2 = map("{:,.2%}".format, map(lambda x: x/100, lst))
print(list(new_lst1))
print(list(new_lst2))
[‘0.03’, ‘0.04’, ‘0.03’, ‘0.10’, ‘0.05’]
[‘2.54%’, ‘4.00%’, ‘3.00%’, ‘9.95%’, ‘5.40%’]
- Lovely!🤗
Advanced Concepts (Optional)
* map()
and filter()
can compliment each other.
If you’d like to filter the elements of your list based on a logical expression, but also map those values to a specific function, you get map()
inside filter()
.
** You can also combine functions from imported Python libraries. We will see some cool examples with numerical Python library Numpy!
Example 9: filter with map and lambda
- Inside
filter()
function, first part tells the filtering criteria (logical expression function), second part is the iterable which is mapped withmap
function.
lst = [1, 4, 9, 11, 400]
a = filter(lambda x: x<100, map(lambda x: x**2,lst))
print(list(a))
[1, 16, 81]
**
operator returns square of a number in Python. If you’d like to refresh your arithmetic operators knowledge in Python > Python Operators Lesson.- When you’re using a
filter
,map
orzip
function as an iterable in a loop or inside another function you don’t have to use thelist()
function to make them look readable and pretty. Computers work with iterables just fine. You can directly iterate on them. - This operation will give same results as
filter()
insidemap()
. You can see filter version of this Python example here.
Example 10: map() numpy operations (sqrt)
- Numpy library has a function called sqrt. This function returns square root of a number. Let’s apply it to a whole list.
import numpy as np
lst = [1, 4, 9, 11, 400, 10000]
root_lst = map(np.sqrt, lst)
print(list(root_lst))
[1.0, 2.0, 3.0, 3.3166247903554, 20.0, 100.0]
Example 11: map() numpy operations (square)
- Let’s reverse that action. Numpy has a function called square which will square numbers. If we you use this we can eliminate the necessity of using multiple steps (** operator with lambda) to square a list of numbers.
import numpy as np
lst = [1, 2, 3, 3.3166247903554, 20, 100]
sq_lst = map(np.square, lst)
print(list(sq_lst))
[1, 4, 9, 11.0, 400, 10000]