Lesson 1: .format()
- ESTIMATED TIME: 7 mins.
- DIFFICULTY: Intermediate
- FUNCTIONS: .format()
- 6 EXERCISES
Format method can be very practical to make data, numbers, strings, floats more representable. And sometimes it can be used to place values in a string in a very convenient way.
It makes value formatting a breeze in Python. It can be used for decimal formatting or it can be used with place holders represented with curly brackets {}. This allows one to create more complicated strings and place independent variables inside the string very conveniently.
Format method can be learned best through examples and exercises. Don’t worry. We got you covered!
Used Where?
- Coveniently placing values or variables in string
- Formatting numbers.
Syntax do(s)
1) One use of format function is using it after strings while including curly brackets: {} inside the string. i.e.:"{} {}".format("Hello ", "World!")
Syntax don't(s)
1) Don't forget that format works in different ways but it's always applied to a string object even when working with numbers."{:,.2f}".format(123.123)
For example this is a common way to reduce float to 2 decimal float. Do you realize how it is always a string method?
Function 1: .format()
.format()
function is a built-in Python method that can be very useful to create formatted versions of values such as strings and numbers.
Example 1: Simple format example
>>> my_message = “Jess and Jack will marry on {}”
>>> wed_date = “May 20”
>>> print(my_message.format(wed_date))
[“Jess and Jack will marry on May 20”]
Value inside format’s parenthesis replaces the curly brackets in the string it’s applied on.
Tips
* There are different ways to use format method in Python
“{:,.2%}”.format() can be used to achieve nice percentage presentation with 2 decimals like: “95.04”
** Similarly, you can increase the decimal value as you’d like. For example, in foreign currency markets and economics it’s common practice to represent numbers with 4 decimals:
“{:,.4f}”.format(12.908374) will return “12.9083”
Example 2: format with multiple values
.format()
method can be even more useful with multiple values that needs to be placed in the string.
>>> user_message = “Baseball weighs {}, American football weighs {} and Basketball weighs {}”
>>> print(user_message).format(“5 oz.”, “15 oz.”, “22 oz.”)
“Baseball weighs 5 oz., American football weighs 15 oz. and Basketball weighs 22 oz.”
Advanced Concepts (Optional)
1- You can combine format method with other functions such as map so that it will be applied to all the elements of an iterable.
See map lesson for more Python examples with map and format.
Example 3: format with map
There is a specific way to apply format method and it can be very useful.
{:,.2f}.format()
will apply 2 decimal float format to your data.
>> lst = [3.6434, 0.9403, 5.37544, 9.00021234]
>>> new_lst = map(“{:,.2f}”.format, lst)
>>> print(list(new_lst))
[‘3.64’, ‘0.94’, ‘5.38’, ‘9.00’]
Example 4: scientific format
If you are a scientist and working with very big numbers (or very small) you might want to use scientific representation. Just use :e
{:e}.format()
will apply 2 decimal float format to your data.
>>> a = “{:e}”.format(9.00021234)
>>> print(a)
9.000212e+00
Example 5: format as thousand separator
And if you are a billionaire format will help you read through zeros in a more convenient way.
{:_}.format()
will apply an underscore between every three zeros.
>>> a = “{:_}”.format(65883900160)
>>> print(a)
65_883_900_160
What is this number for god’s sake? 🧐
Oh, thank you so much young man! 😁
Next Lesson: .join() method
“Have you installed Python yet?”
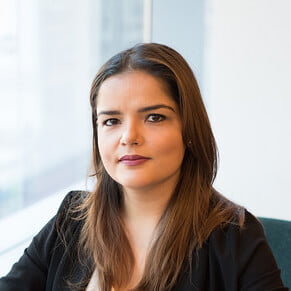