Python Format Exercises
Let’s check out some exercises that will help you understand .format() method better.
Exercise 1-a: Python format method of strings
Place a curly bracket "{}" in the appropriate place and fill inside .format()'s parenthesis so that "Hello!, Name" is printed.
Exercise 1-b: Format method to type a number
Using a string and .format() method print the number: 1 only.
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
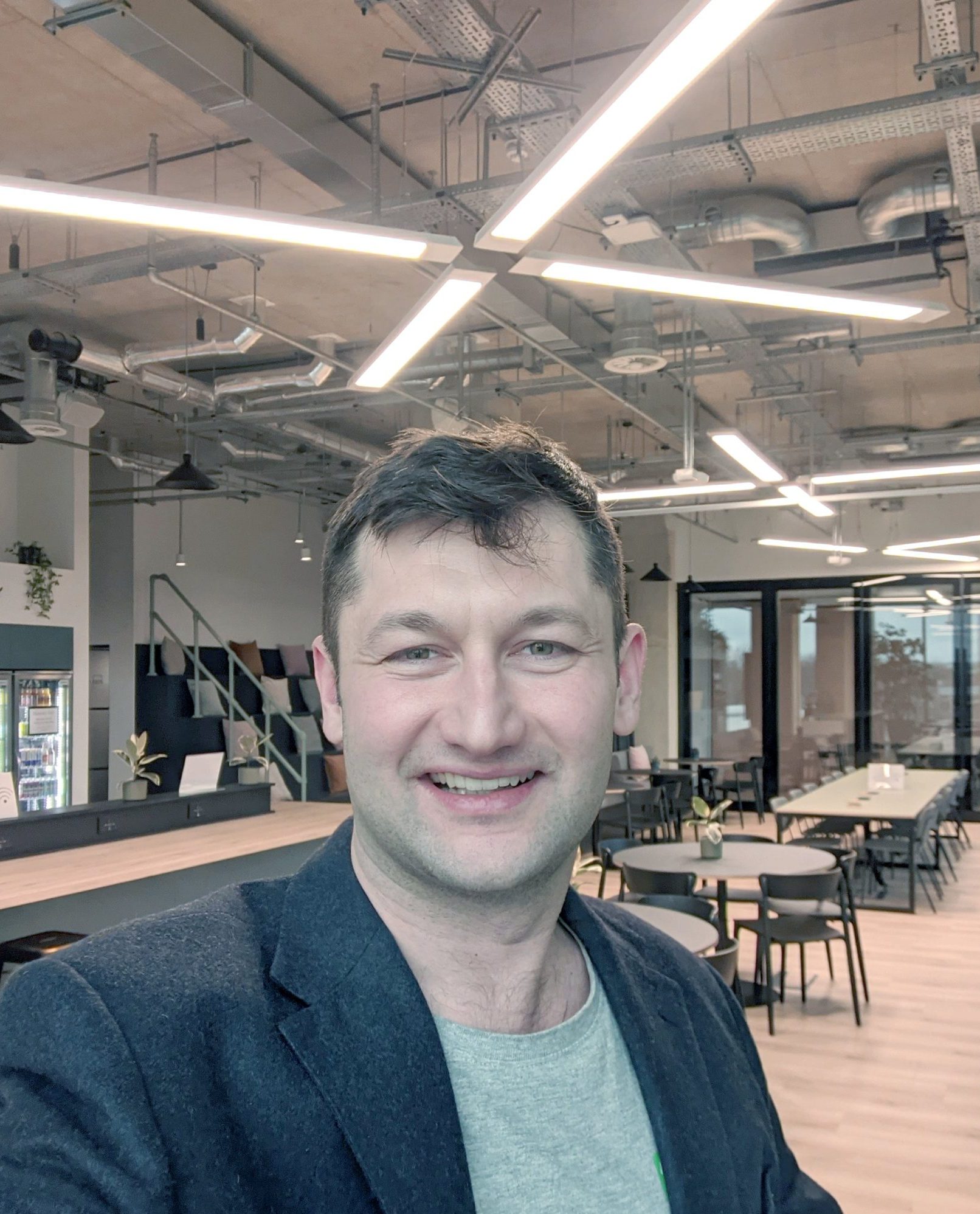
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython