Python If, Elif, Else Exercises
Conditional Statement is a very important concept in computer programming as well as in Python.
Let’s check out some exercises that will help understand Conditional Statements (if-elif-else) better.
Exercise 7-a
Write an if statement that asks for the user's name via input() function. If the name is "Bond" make it print "Welcome on board 007." Otherwise make it print "Good morning NAME". (Replace Name with user's name)
input() function will return the input from the user.
name = input(“Please enter your name.”)
An if – else statement will achieve what you need.
if name == xx:
statement1
else:
statement2
Make sure to note logical operators can be slightly different than mathematical operators in Python (i.e.: “==” instead of “=”)
name = input("Please enter your name.")
if name == "Bond":
print("Welcome on board 007.")
else:
print("Good morning " + name)
Exercise 7-b
Do the same thing as exercise 7-a this time making sure if the name is bond with lower case b it still prints "Welcome on board 007."
input() function will return the input from the user.
name = input(“Please enter your name.”)
An if – else statement will achieve what you need.
if name == xx:
statement1
else:
statement2
Make sure to note logical operators can be slightly different than mathematical operators in Python (i.e.: “==” instead of “=”)
if you construct your logical statement with name.lower() it will work either way.
name = input("Please enter your name.")
if name.lower() == "bond":
print("Welcome on board 007")
else:
print("Good morning " + name)
Exercise 7-c
Write a function named "evens" which returns True if a number is even and otherwise returns False.
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
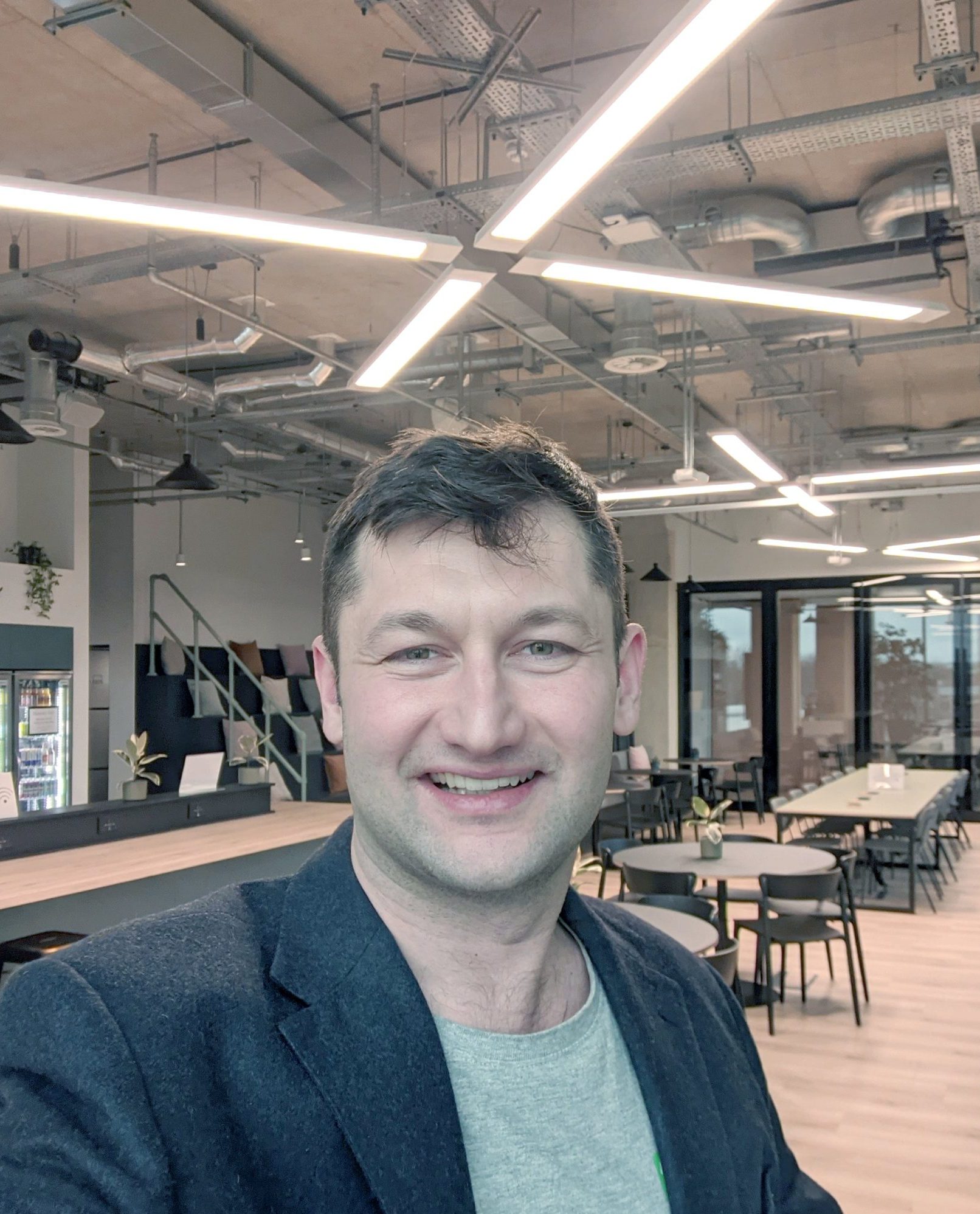
Founder of HolyPython