Python While Loop Exercises
Let’s check out some exercises that will help understand While Loops better.
Exercise 9-a
Write a while loop that adds all the numbers up to 100 (inclusive).
you can start with while counter < 100:
One of the key aspect of writing while loops is watching your counters. For instance, when you type while counter < 100:
If you don’t increase counter in every loop it will create an infinite loop as counter will stay the same and always below 100. Eventually you’ll get a runtime error.
So defining i before the loop:
counter = 0
And increasing i inside the loop:
counter = counter+1
are key points in most while loops.
This can be confusing for absolutely new programmers but you also need another counter which adds up the numbers during the iteration.
With some repetition and pondering you will definitely get a grip of while loops, it’s normal to struggle with them slightly more than for loops which usually doesn’t have to bother with counters for the loop to function properly.
So,
total = 0
before the loop;
and,
total = total+counter
inside the loop will ensure that total adds each number to the sum along the way during the iteration.
while counter <= 100:
total= total+counter
counter= counter+1
Exercise 9-b
Using a while
loop, len
function, an if
statement and the str()
function; iterate through the given list and if there is a 100, assign a notification message to the variable my_message
with the index of 100.
i.e.: "There is a 100 at index no: 5"
You can start with defining a counter:
i = 0
To make sure you go through the list you can construct your while loop as:
while i < len(lst)
Most important thing to remember, so that you don’t get an error is to increase i in each iteration as:
i = i+1
Inside your while loop, you can use an if statement as such:
if lst[i] == 100:
…
Besides being the counter i is being used as the index throughout the list and lst[i] is the value of elements in the list at index: i
message = "" i = 0 while i < len(lst): if lst[i] == 100: my_message = "There is a 100 at index no: " + str(i) i = i+1
Exercise 9-c
Using a while
loop and an if
statement write a function named name_adder which appends all the elements in a list to a new list unless the element is an empty string: "".
You can start with defining a counter and a new empty list which will be used for appending.:
i = 0
new_list = []
and inside the loop i = i+1
Again you can iterate during the length of the list.
Inside the loop you can make an if statement that
if list[i] != “”:
…
which means if list[i] is not equal an empty string.
Make sure your function returns the new list too.
def name_adder(list): i = 0 new_list = [] while i < len(list): if list[i] != "": new_list.append(list[i]) i = i+1 return new_list
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
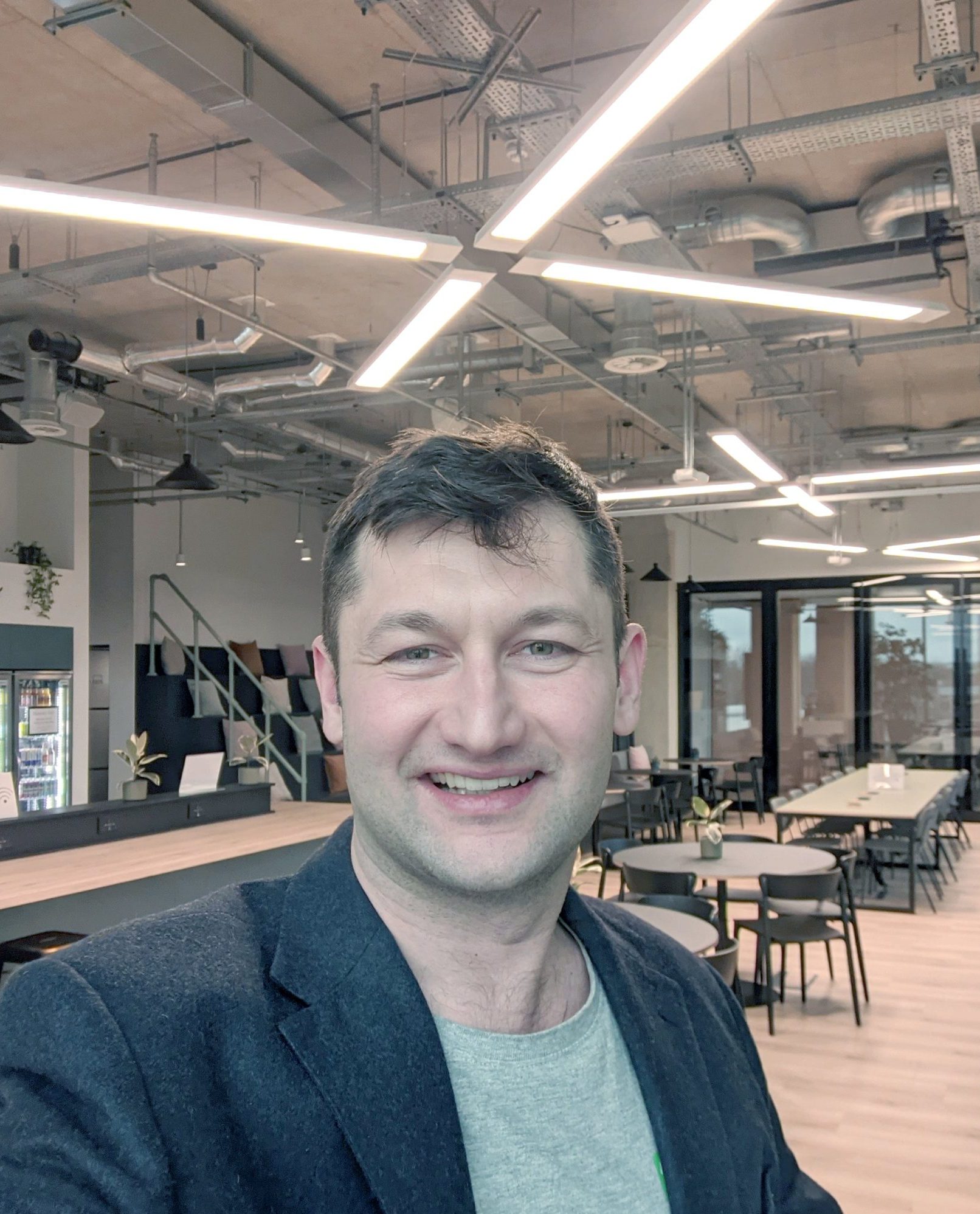
Founder of HolyPython