Python Filter Exercises
Let’s check out some exercises that will help understand filter() function better.
Exercise 14-a
Using filter() function filter the list so that only negative numbers are left.
filter(f, list) can be useful. Make sure your function is a logical statement to facilitate the filtering process.
Since filter() function will return an iterator, you can use list() function to convert it to a proper Python list.
lst2 = list(filter(lambda x: x<0, lst1))
print(lst2)
Exercise 14-b
Using filter function, filter the even numbers so that only odd numbers are passed to the new list.
Exercise 14-c
Using filter() and list() functions and .lower() method filter all the vowels in a given string.
You can use filter(f, list). Make sure your function is a logical statement to facilitate the filtering process.
Since filter() function will return an iterator, you can use list() function to convert it to a proper Python list.
To create a lambda function with logical expression you can implement something like this:
lambda x: True if x in ….else False
lst = list(filter(lambda x: True if x.lower() in "aeiou" else False, str1))
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
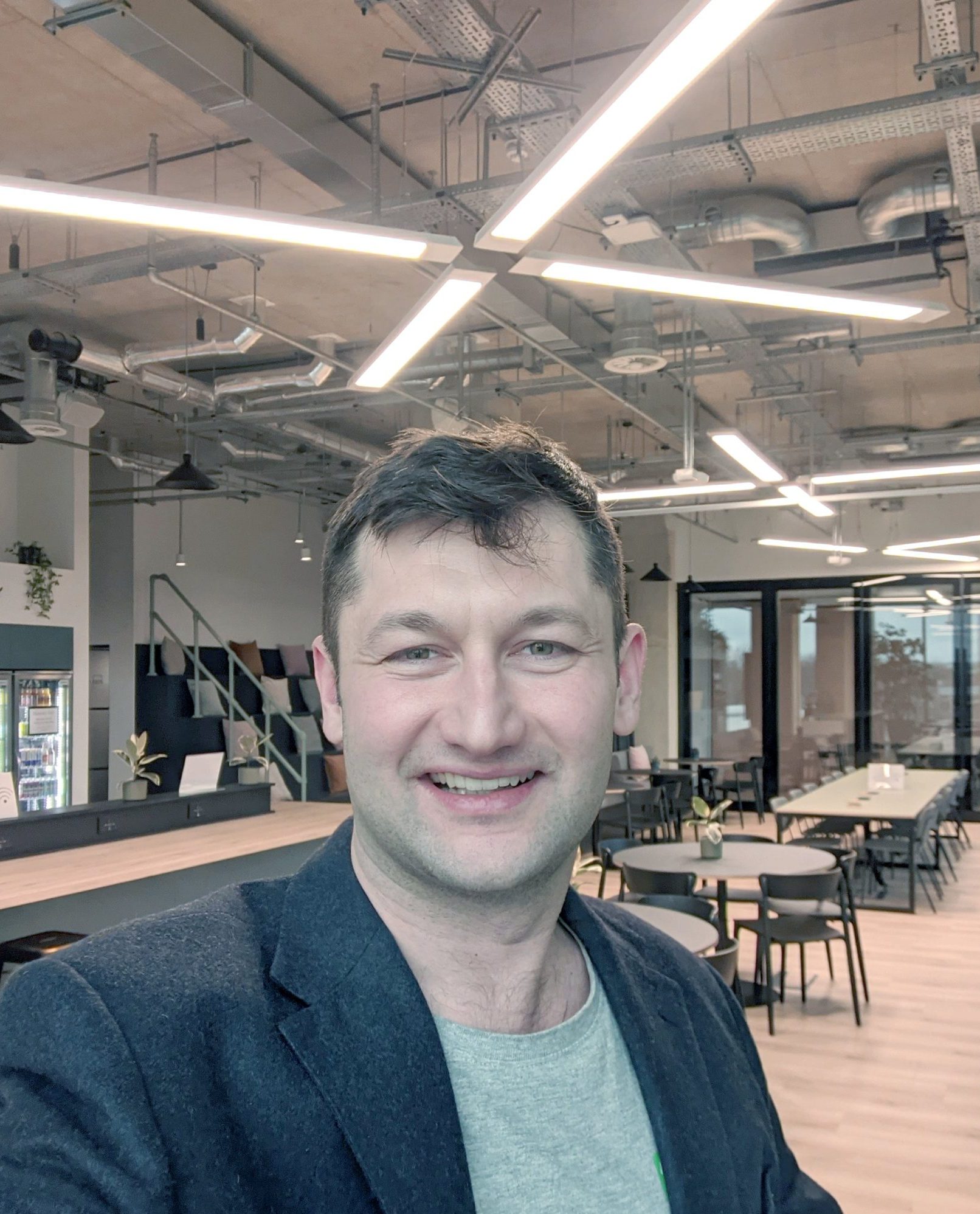
Founder of HolyPython