Python filter function (Filters each item in a sequence)
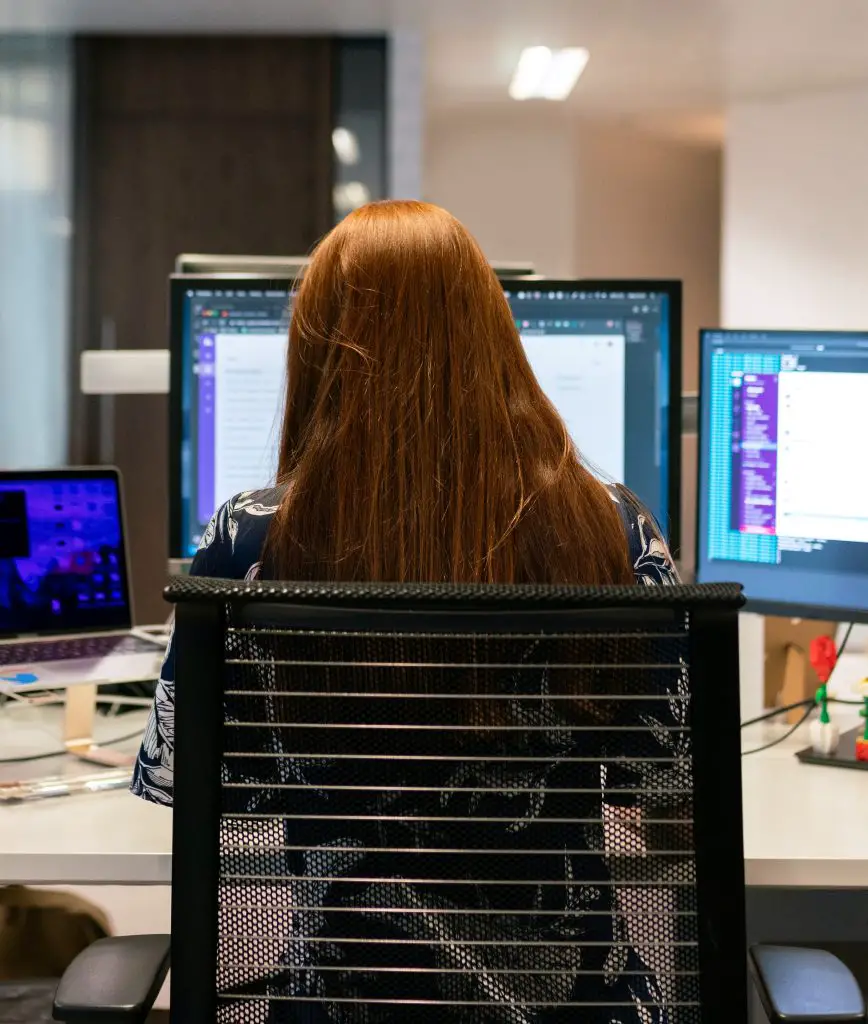
filter
function filters each element of an iterable (such as lists or strings) based on a function passed as an argument to the filter
function.
It resembles map function very much and can be combined with it. But what’s different than map function’s usage is filter function applies a conditional statement as a filter to the elements of an iterable.
Estimated Time
12 mins
Skill Level
Intermediate
Functions
filter()
Course Provider
Provided by HolyPython.com
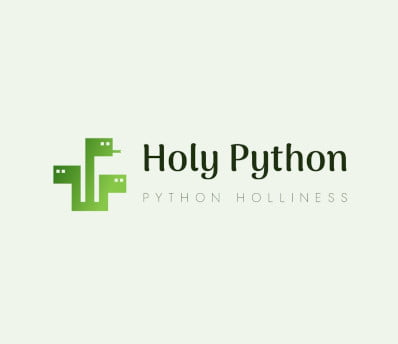
Using filter() function
, you can take a list and apply a filtering statement (such as x < 100).
In this case, you will end up with a filtered list of value below 100. This concept can be elaborated and used in many different ways.
In plain English here is the difference between map and filter functions:
If you have a function such as:
- multiply by 100 (x*100)
- divide by 10 (x/10)
- convert to integers (int(x))
- convert to lower characters (lower(x))
- Filter below 100 ( x<100 )
- Filter words only if first letter is capital ( upper(x[0]) == True )
- Filter if length is over 10 ( len(x) > 10 )
- Filter if value is positive ( x > 0 )
Used Where?
filter
function allows filtering items based on a criteria passed as argument. It often comes very handy.
The fact that you can pass any logical function as an argument creates a high freedom of applications.
You might want to filter your elements based on, but not limited to, below criteria:
- length of the items
- Numerical value
- above zero
- below zero
- odd number
- even number
- division remainder equals zero
- absolute value
- etc.
- includes certain letter or not
- alphabetic order
- reverse alphabetic order
Syntax do(s)
1) inside filter
function's parenthesis type your function followed by a comma
2) then the name of a list, tuple, dictionary or string i.e.: filter(f, iterable)
Syntax don't(s)
1) Don't print filter
function directly, you will only get a unique memory address
2) Don't forget to put your filter
function inside a list()
function if you'd like to create meaningful results that are readable by the user.
Example 1: Positive numbers
>>> a = lambda x: x>=0
>>> lst = [-3, 4, 71, -555, -0.02, 0]
>>> lst_filtered = filter(a, lst)
>>> print(list(lst_filtered))
[4, 71, 0]
- We created a function with
lambda
and logical expression: greater or equal (<=
) and assigned to letter a. - We passed our function to the
filter()
function - Using
list()
function we converted our result to a readable list.
Example 2:
>>> a = lambda x: print(x*3)
>>> print(a(3))
9
Example 3: Even numbers
Let’s create a function that checks for even numbers and then use it in filter()
function.
>>> a = lambda x: x%2==0
>>> lst = [75, 4, 55, 65, 22]
>>> lst = filter(a, lst)
>>> print(list(lst))
[4, 22]
This time we assigned the filtered result to the original list named <lst>
Example 4: Odd numbers
Let’s create a function that checks for odd numbers this time and then apply it to filter()
function.
>>> a = lambda x: x%2==1
>>> lst = [75, 4, 55, 65, 22]
>>> lst = filter(a, lst)
>>> print(list(lst))
[75, 55, 65]
Printing the function object
You might at some point encounter a strange output when you’re learning Python. This happens when you try to print function objects directly without without calling it with any value.
What you get will be the id of your function as a memory address rather than any meaningful value to the user.
Example 5: Any vowels?
>>> t = “Winter Olympics”
>>> a = lambda x: True if x in “a,e,i,o,u,A,E,I,O,U” else False
>>> t_filtered = list(filter(a, t))
>>> print(t_filtered)
[‘i’, ‘e’, ‘O’, ‘i’]
You can filter all the vowels in a text.
Example 6: Capital letters
>>> t = “Winter Olympics”
>>> a = lambda x: True if x.lower() in “a,e,i,o,u” else False
>>> t_filtered = list(filter(a, t))
>>> print(t_filtered)
[‘i’, ‘e’, ‘O’, ‘i’]
.lower()
method for strings previously. By adding that to your variable you can check if lowercase versions of all the letters are in the list and filter them based on that. Tips
1- Sometimes it can be confusing to understand functions with logical expressions vs functions that return a numerical value.
A function with logical expression as below, will return a True or False value based on the criteria and this is what the filter()
function needs to filter its iterable’s (list or other) elements.:
a = lambda x: x>=0
a(5)
True
a(-5)
False
Versus when we use the map() function, we generate functions that actually change the value of the given variable instead, for instance:
a = lambda x: x^2
input: a(5)
output: 25
Example 7:
.sort()
is a list method that sorts the list’s elements directly.
>>> lst = [1, 5, 66, 7]
>>> lst.sort(key=lambda x: x)
print(lst)
[]
if you’d like to use a function as an argument in .sort()
you need to use it with “key=
” keyword.
Filter Map Combinations
map()
you can apply functions to each item in a sequence based on a filtering criteria.I.e.: Imagine a Python dictionary of countries and their population. You want to return a list with each value increased by 20% but you only want to return countries above 10 million population.
By combining map and filter you can end up with a dictionary which multiplies these values by 1.2 only if the population is above 10,000,000. Half of this operation is achieved by filter function while the other half is achieved by map function.
We have plenty of filter function exercises as well as map function exercises to make this concept super clear and help you understand Python better.
Advanced Concepts (Optional)
1- filter()
and map()
can have a completing relation.
If you’d like to map the elements of your list to specific values, but also only do this to the elements that pass the filter, you get filter()
inside map()
.
This operation will give same results as map()
inside filter()
. We also have that version of this example at the end of map()
function’s lesson here.
filter()
function will produce your filtered iterable insidemap()
function as below:map(function, iterable)
- where function will be the map object
- where iterable will be:
filter(function, iterable)
- where function will be logical expression criteria
- and iterable will be the actual iterable list or other.
Let’s see an example.
Example 8: map with filter
map()
function, first part tells what function to map values to, second part is the iterable which is filtered with filter function. >>> lst = [1, 4, 9, 11, 400]
>>> a = map(lambda x: x**2, filter(lambda x: x<10, lst))
>>> print(list(a))
[1, 16, 81]
- When you’re using a
filter
,map
orzip
function as an iterable in a loop or another function you don’t have to use thelist()
function to make them look readable and pretty. You can directly iterate on them.