Turtle Stars
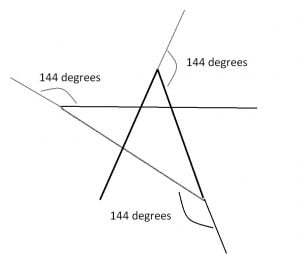
Introduction
Drawing with turtle library of Python can be very educational and fun.
In this tutorial we will focus on how to draw stars with turtle in Python.
We’re going to show you how to draw monocolor stars such as yellow stars as well as how to draw multicolor stars.
Then we will attempt to scatter a number of stars on a dark blue night sky using turtle drawing and Python. Let’s see.
Star Geometry
So how can we draw a perfect star with code in Python? It turns out a star polygon or in this case a star pentagon specifically has 5 sides with external angle of 144 on each side (internal angle of 36 degrees).
- Let’s start with importing turtle library first.
import turtle
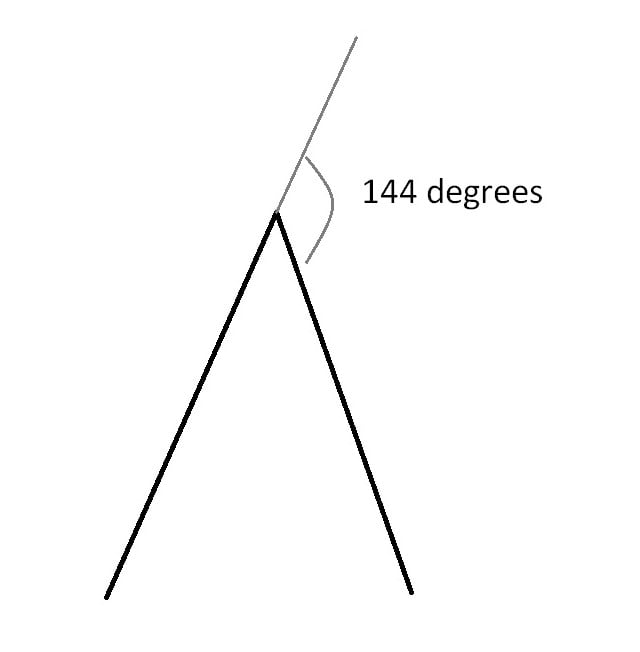
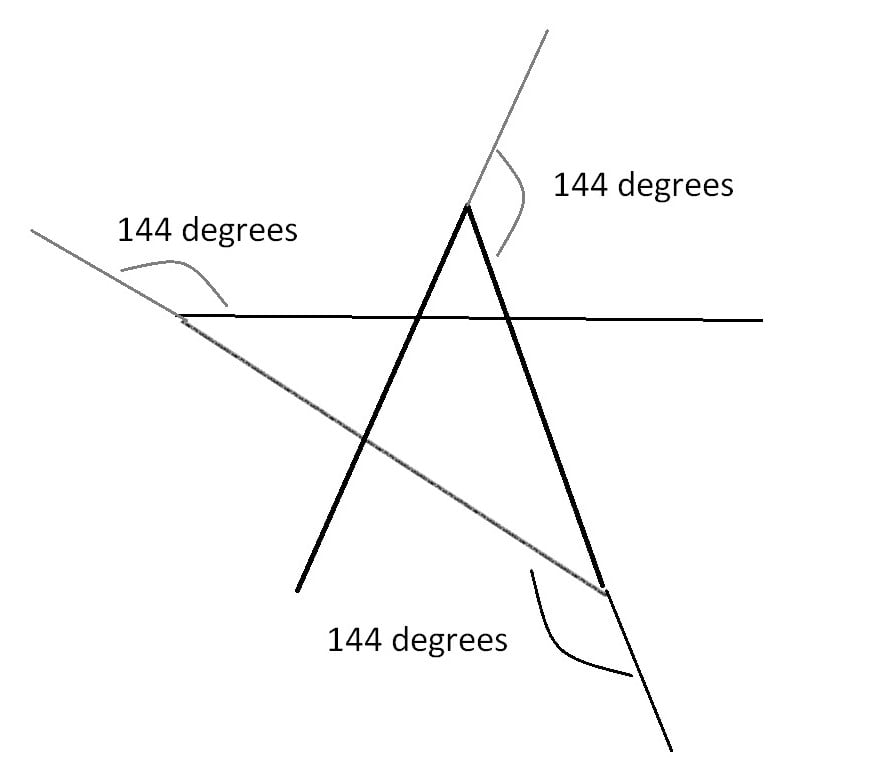
This makes everything so much easier as we love repetition in coding. Only 5 iterations with same turn angles will do.
Example 1: One Simple Star
After opening an image you can use the .resize() method on it to create a new image with a new size.
Please Note: Adding turtle.exitonclick()
can help terminate turtle window once you’re done with your turtle drawing or observations. If you don’t add this little code piece, turtle tends to freeze which requires a kernel restart which can be annoying. So we will add it to the end of each code and you’re also advised to do so.
import turtle
for i in range(5):
turtle.forward(150)
turtle.right(144)
turtle.exitonclick()
Here is turtle in action. We figured out a single star. Yay!
It’s downhill from this point. Now we can add some spice. Maybe some colors. And definitely some more stars so they don’t feel lonely 😉
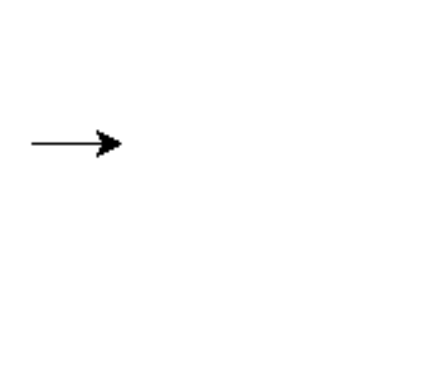
Example 2: Yellow Star
We can simply use .color method to give turtle a color: turtle.color("yellow")
import turtle
turtle.color("yellow")
for i in range(5):
turtle.forward(150)
turtle.right(144)
turtle.exitonclick()
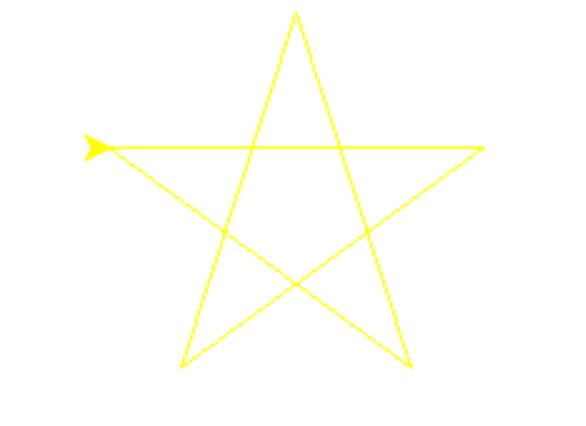
Great now our star has some color even. We can also transform the background to a color like dark blue which will make the star’s yellow pop better.
Example 3: Dark Blue Night Sky
We can use .bgcolor method to change the background color of a turtle drawing window: turtle.bgcolor("darkblue")
import turtle
turtle.color("yellow")
turtle.bgcolor("darkblue")
for i in range(5):
turtle.forward(150)
turtle.right(144)
turtle.exitonclick()
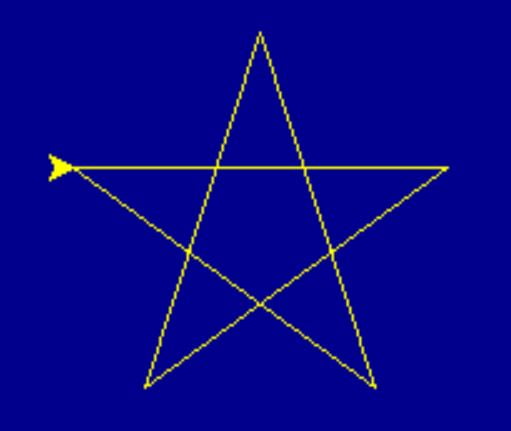
Perfect. On your computer it might look even better actually. On the website it’s being scaled up which makes the resolution appear worse than it is.
Now, let’s find a cure for loneliness.
Example 4: Multiple Stars
turtle’s setpos()
or goto()
methods can be used to make the cursor travel between different coordinates and this way we can draw a different star at each coordinate. We will have to adjust a few things:
- Let’s turn down the star size quite a bit. This can be easily adjusted by passing a smaller value to
.forward()
command which draws each side of the star. - We can implement a random.randint() structure which tells a random x,y coordinate on our pseudosky.
- Also, let’s speed up the turtle so we don’t have to wait too long for each star to be drawn. This can be achieved with
turtle.speed(0)
- 0 : fastest setting while
- 10 : slowest setting
- Finally, I have a feeling that if we put our star drawing algorithm in a user defined function everything will be less messier.
- By the way, let’s also hide the turtle for a smoother example. This can be done with
turtle.hideturtle()
Let’s try. Here is the Python code.
import turtle
turtle.color("yellow")
turtle.bgcolor("darkblue")
turtle.speed(0)
turtle.hideturtle()
def starmaker(step,angle):
for iter in range(5):
turtle.forward(step)
turtle.right(angle)
for i in range(10):
turtle.penup()
turtle.setpos(random.randint(-350, 350), random.randint(100, 300))
turtle.pendown()
starmaker(10,144)
turtle.exitonclick()
And, here is the result.
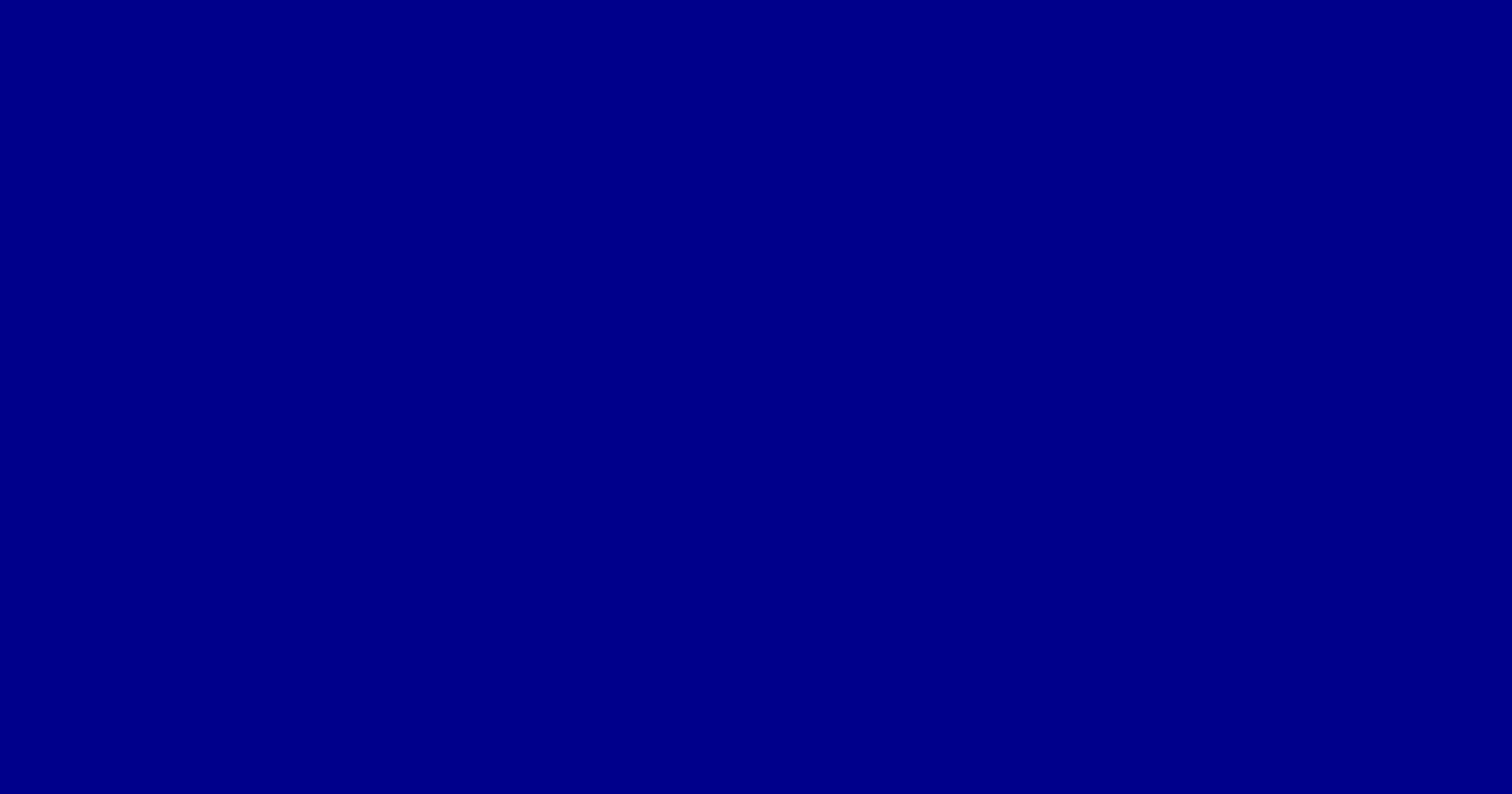
Wow! Now we’re talking. Our turtle drawing is starting to look legit. It might even compete with Van Gogh’s Starry Night, what do you think? Alright, I think that’s an exaggeration too too. But it’s still pretty good for turtle digital art category.
Where can we go from here? Well, rather than a solid yellow, stars kind of flicker . Maybe we can draw stars with multiple colors just for fun?
Example 5: Multi-color Multiple Stars
So, how can we make each side of the star a different random color? Pretty easy actually.
By placing turtle.color()
attribute inside the turtle making function’s for loop, we can make sure turle’s color is redefined at each iteration.
We can also create a list of predefined colors. Then using random library we can pick a random color by randomly accessing the indexes of this color list. Remember that list index starts at 0 in Python so random.randint()
should go to list size-1 at maximum.
import turtle
turtle.bgcolor("darkblue")
turtle.speed(0)
turtle.hideturtle()
color_lst=["red", "orange", "cyan", "yellow", "gray", "lightgreen"]
def starmaker(step,angle):
for iter in range(5):
turtle.color(color_lst[random.randint(0,5)])
turtle.forward(step)
turtle.right(angle)
for i in range(15):
turtle.penup()
turtle.setpos(random.randint(-350, 350), random.randint(100, 300))
turtle.pendown()
starmaker(10,144)
turtle.exitonclick()
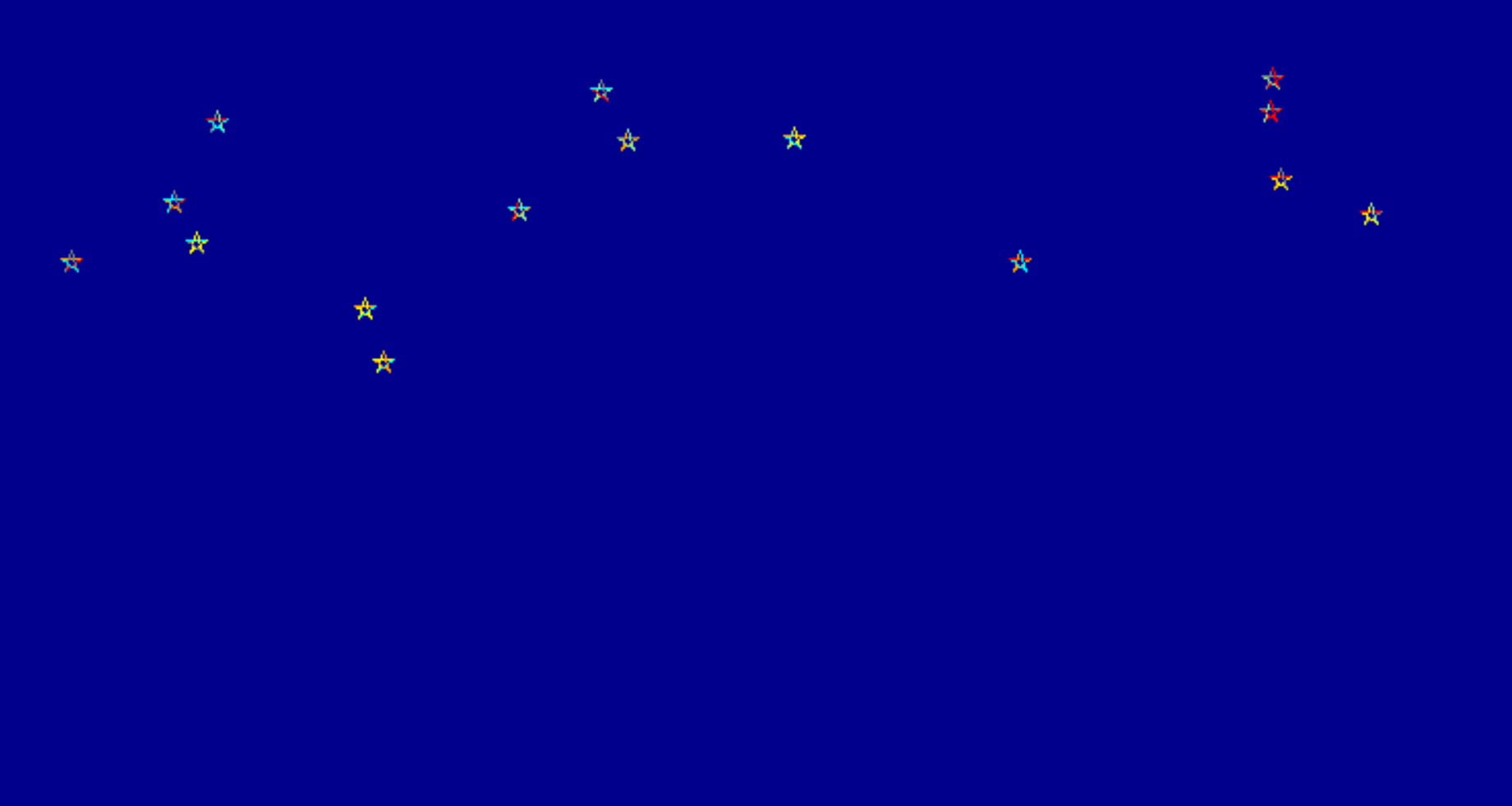
Here we go we started with a single black & white star and discovered all the way up to multiple little stars with multiple colors on a dark blue night sky.
Resources
You can check out other turtle tutorials we have for more examples and turtle knowledge.
Summary
In this Python Tutorial, we have learned how to draw stars with turtle.
We have also advanced the simple idea of drawing a star and played with it a litte. We ended up drawing multiple good looking stars on a night sky.
This tutorial can be a great practice to demonstrate basic Python concepts. Feel free to freely use and share at your school or work, attributions or source credit are very welcome.
Thank you for your support!