Turtle penup() and pendown()
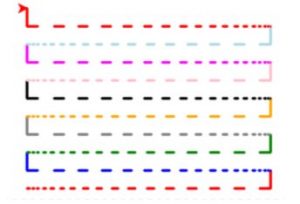
Introduction
In this tutorial we will look at turtle’s .penup() and .pendown() methods. Turtle operates with pendown state by default.
But, if you use penup() it will stop drawing when you move the turtle. To start drawing again, you will need to use pendown() to go back to previous drawing state.
Just as in physical drawing (for art or technical drawing), it can be useful lift the pen off the paper or canvas. Normally not all shapes are 100% connected in a drawing and sometimes you need to go from point to point without drawing and then start drawing from there again.
This is pretty much the main purpose of penup() and pendow().
.penup() method
.penup() will lift the turtle off the “digital canvas” and if you move the turtle in penup state it won’t draw.
- Moving the turtle without drawing
- This is useful for repositioning the turtle
- making breaks in the drawing
-
from PIL import Image, ImageFilter
.pendown() method
.pendown() is the default state of turtle. It will ensure the turtle draws when it’s moving with your commands such as forward() or setpos(). Since pendown is the default state normally when you move turtle with a command like .forward() it draws by default.
- .pendown() is mostly useful to reestablish pendown state after using .penup().
- If you use penup() and then not switch back to pendown() turtle will continue moving but won’t draw.
Example 1: Simple .penup() example
Here is a rather simple iteration. Forward, penup, forward, pendown, and so on.
import turtle
turtle.color("green")
turtle.hideturtle()
turtle.speed(1)
turtle.left(90)
for i in range(4):
turtle.forward(30)
turtle.penup()
turtle.forward(30)
turtle.pendown()
turtle.exitonclick()
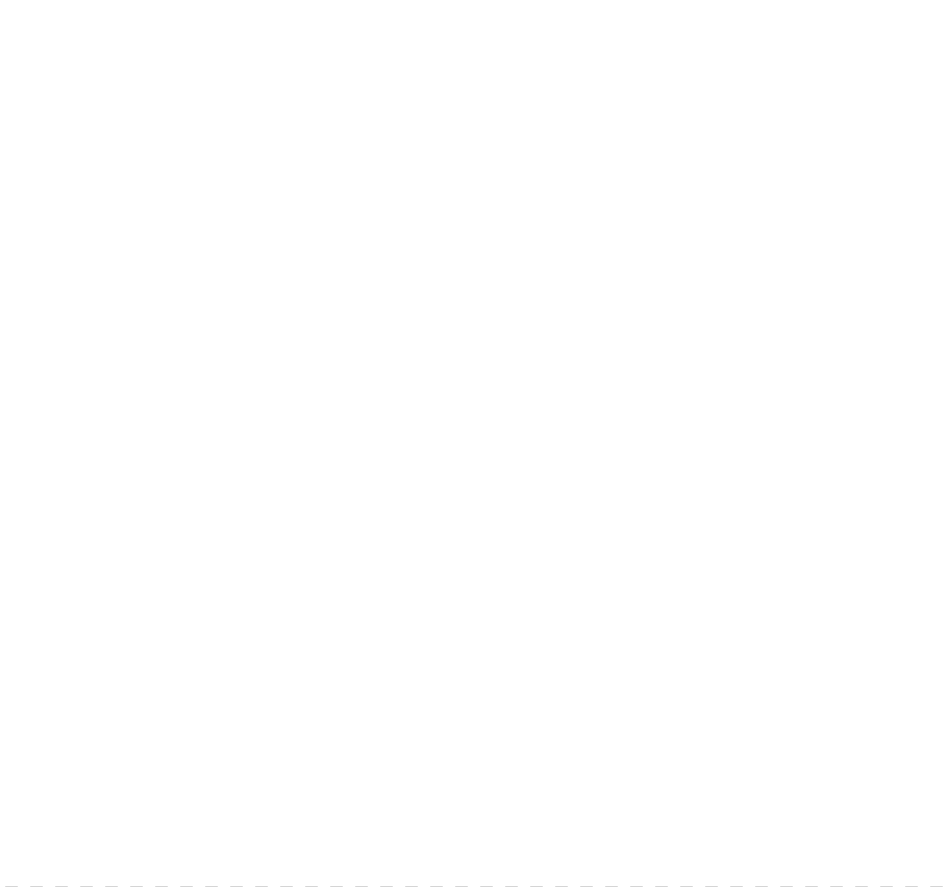
Example 2: Pacmanesque Colorful Dashes
Here is a more sophisticated code that builds colorful dashes horizontally and vertically using .penup() and .pendown()
import turtle
a = turtle.Turtle()
a.penup()
a.setpos(-350,-300)
a.pendown()
a.speed(0)
a.pensize(3)
colors = ["red", "blue", "green", "gray", "orange", "black", "pink", "magenta", "lightblue"]
def onecycle():
for i in range(37):
if i%2==0:
a.pendown()
a.forward(i//3)
else:
a.penup()
a.forward(i/2)
for i in range(10):
a.color(colors[i%9])
onecycle()
if i%2==0:
a.left(90)
a.fd(20)
a.left(90)
else:
a.right(90)
a.fd(20)
a.right(90)
turtle.exitonclick()
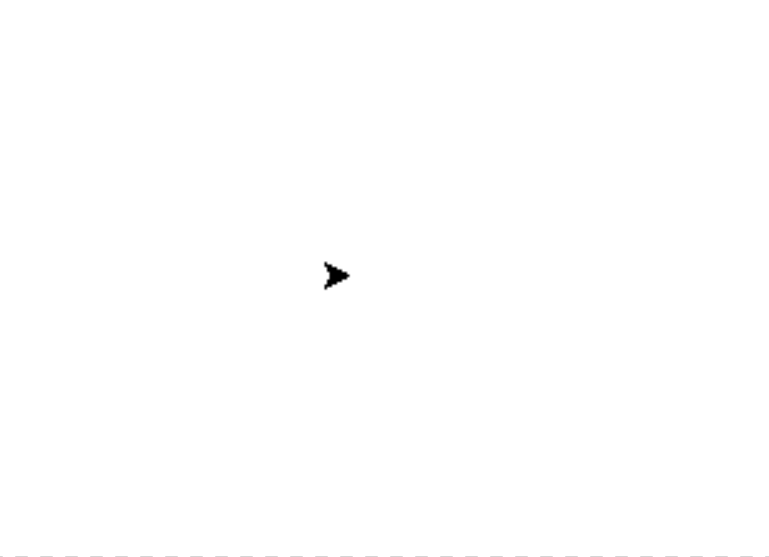
Example 3: Mors Code with Turtle
Mors code is a communication method to use signals as letter encoding to share a message.
Recently if you’ve watched the South Korean masterpiece Parasite you will remember the scenes where some of the characters use Mors code unnoticed.
Since all you need to utilize Mors code is a signal of some sort and two different durations (short and long or dot and line), it makes it very handy in some emergency situations where you don’t have other communication means or in situations where you need to be more subtle about your message.
You can technically produce message via Mors using any signal. These can be:
- sound
- light (flashlight, projector, light bulb)
- sticks
- pen
- smoke etc.
- and in this case, Python turtle!
Full disclosure by the way, Holy Python Team members are safe and sound and this is only a demonstration! 🙂
Here is how it looks in Python. You can find the code below.
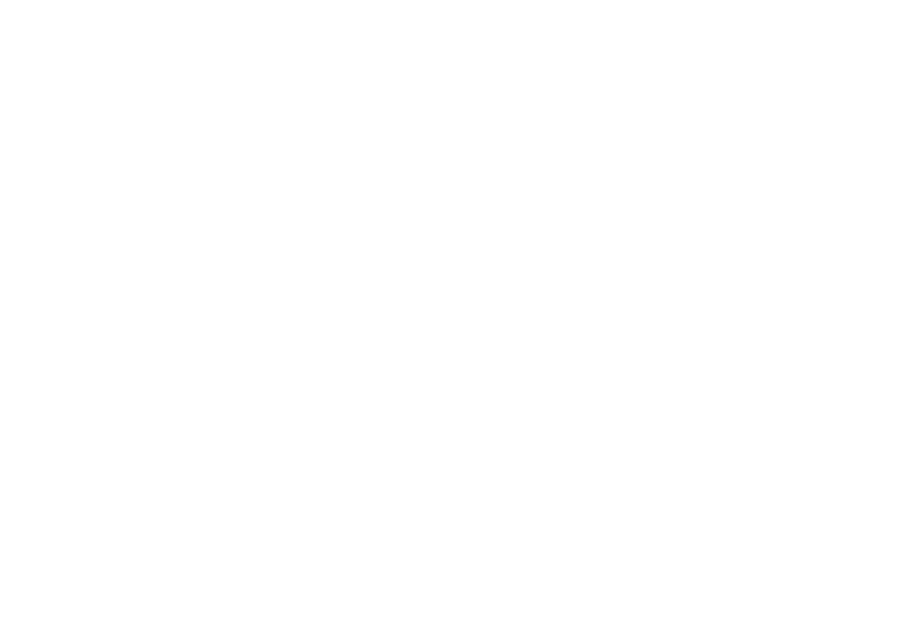
Although For Loops are used in the functions for letters “H” and “P”, same result can be achieved without loops if you’d like to construct a more basic demonstration for teaching.
import turtle
a = turtle.Turtle()
a.hideturtle()
a.penup()
x, y = -350, 300
a.setpos(x,y)
a.pendown()
a.speed(0)
a.pensize(3)
def mors_H():
for i in range(8):
if i%2==0:
a.pendown()
a.forward(2)
else:
a.penup()
a.forward(7)
def mors_E():
a.pendown()
a.forward(2)
a.penup()
a.forward(7)
def mors_L():
a.pendown()
a.forward(2)
a.penup()
a.forward(7)
a.pendown()
a.forward(12)
a.penup()
a.forward(7)
a.pendown()
a.forward(2)
a.penup()
a.forward(7)
a.pendown()
a.forward(2)
a.penup()
def mors_P():
a.pendown()
a.forward(2)
a.penup()
a.forward(5)
for i in range(4):
if i%2==0:
a.pendown()
a.forward(10)
else:
a.penup()
a.forward(7)
a.pendown()
a.forward(2)
a.penup()
a.forward(5)
def nextline(margin):
a.setpos(x,y-margin)
mors_H()
nextline(50)
mors_E()
nextline(100)
mors_L()
nextline(150)
mors_P()
turtle.exitonclick()
Summary
In this Python Tutorial, we have learned .penup() and pendown() methods of Python’s turtle.
Additionally, we have seen a couple of interesting examples that demonstrate the usage and potential of these methods.
We have many more interesting Python tutorials. Feel free to explore other categories we have prepared for you. And feel free to share with your friends, students and social media audience!
Thank you.