Turtle hideturtle() and showturtle()
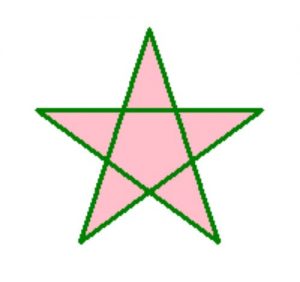
Introduction
.hideturtle()
and .showturtle()
methods can be used to hide and show turtle drawing icon.
It can be particularly helpful during or after the drawing to improve visibility or aesthetics of the turtle drawing.
Default state of turtle is .showturtle() but you can use .hideturtle() to hide the turtle. Drawings will still proceed but you just won’t see the turtle icon that makes the drawing. .hideturtle()
Just add- This method can be used as this Python code sample:
turtle.hideturtle()
.showturtle()
If you hid the turtle you will need .showturtle() to bring it back. Otherwise it will remain visible.
- You can use this code sample to show turtle again.
turtle.showturtle()
Example 1: Flashing Turtle
Hiding and showing turtle is a really straightforward procedure. We want to get a little creative with demonstrating it.
Using a Python for loop, you can make turtle disappear and reappear continuously creating some kind of a flashing effect.
Here is the code:
import turtle
turtle.hideturtle()
turtle.pen(pencolor="green", pensize=3)
turtle.begin_fill()
for i in range(5):
turtle.forward(150)
turtle.right(144)
turtle.fillcolor("pink")
turtle.end_fill()
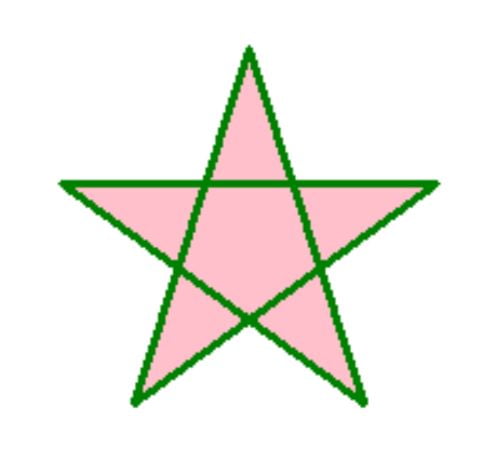
Example 2: Flashing Turtle
Hiding and showing turtle is a really straightforward procedure. We want to get a little creative with demonstrating it.
Using a Python for loop, you can make turtle disappear and reappear continuously creating some kind of a flashing effect.
Here is the code:
import turtle
colors = ["red", "blue", "green", "gray", "orange", "black"]
a = turtle.Turtle()
a.speed(5)
for i in range(130):
a.color(colors[i%6])
if i%2==0:
# a.pendown()
a.forward(i/10)
a.hideturtle()
else:
# a.penup()
a.forward(5+i/10)
a.showturtle()
a.left(40 - i/1.5)
turtle.exitonclick()
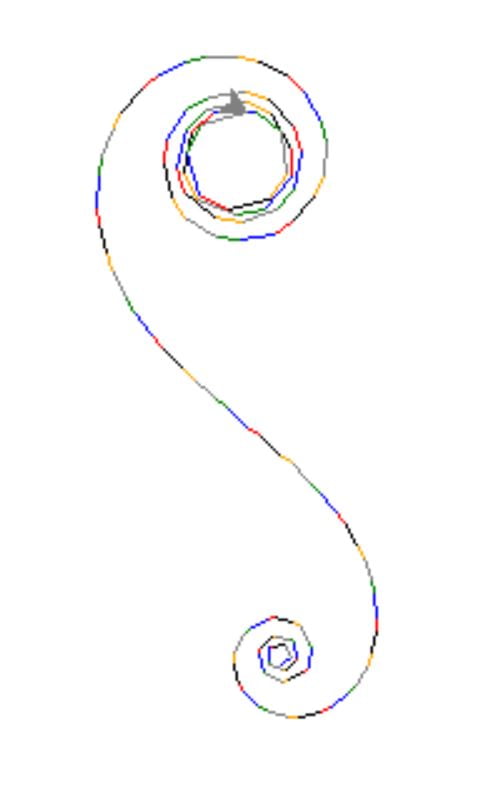
Summary
In this Python Tutorial, we’ve covered turtle hideturtle()
and showturtle()
methods. We’ve learned when these turtle methods can be useful and how to use them.
We have also demonstrated this knowledge with a couple of appropriate Python examples that show usage of hideturtle() and showturtle() in practice.
Thank you for visiting Holypython’s turtle tutorials!