Turtle Fill
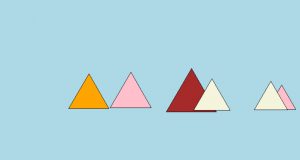
Introduction
In some cases you might need more than just drawing.
Filling is a big part of drawing and Python’s turtle has very handy Python methods that can be used to fill shapes and patterns with different colors.
In this tutorial we’re going to show you how to use .fillcolor()
, .begin_fill()
and .end_fill()
methods.
How to fill turtle drawings with color?
Filling a drawing in turtle is pretty simple.
You just need to place your drawing between begin_fill and end_fill commands.
- A typical fill operation in turtle can be like this:
- Define a filling color using
turtle.fillcolor()
- Start the filling operation with this command:
turtle.begin_fill()
- Perform your turtle drawing in the middle
- End the filling operation with this command:
turtle.end_fill()
- Define a filling color using
If you’ve done it right turtle will fill the drawing with your choice of color once the drawing ends.
.fillcolor()
Filling a drawing in turtle is pretty simple.
You just need to place your drawing between begin_fill() and end_fill() commands.
- A typical fill operation in turtle can be like this:
- Define a filling color using
turtle.fillcolor()
- End the filling operation with this command:
turtle.end_fill()
- Define a filling color using
If you’ve done it right turtle will fill the drawing with your choice of color once the drawing ends.
.begin_fill()
This method activates filling operation in turtle. It should be placed before the drawing of a pattern.
- It also makes sense to include a
turtle.fillcolor()
object before theturtle.begin_fill()
object.- Define a filling color using
turtle.fillcolor()
- End the filling operation with this command:
turtle.end_fill()
- Define a filling color using
If you’ve done it right turtle will fill the drawing with your choice of color once the drawing ends.
.end_fill()
Filling a drawing in turtle is pretty simple.
You just need to place your drawing between begin_fill() and end_fill() commands.
- A typical fill operation in turtle can be like this:
- Define a filling color using
turtle.fillcolor()
- End the filling operation with this command:
turtle.end_fill()
- Define a filling color using
If you’ve done it right turtle will fill the drawing with your choice of color once the drawing ends.
Example 1: Filling A Star
We have a fun Python tutorial on how to draw stars. Now, let’s try to draw a star and fill it with color.
- Don’t forget to pass the new size in tuples:
img.resize((x, y))
- Also. don’t forget that you will have to assign the new image with new size to a new variable or you can also reassign it to the same variable and overwrite it.
Shortly, a pentagon star can be drawn with 5 lines. Making a turn with 144 degrees of external angle each time will result in a star. So, we need:
- 5 iterations (can be achieved easily with a Python for loop)
- 144 degree turns
import turtle
turtle.begin_fill()
for i in range(5):
turtle.forward(150)
turtle.left(144)
turtle.end_fill()
turtle.exitonclick()
Now let’s apply begin_fill() using turtle, so that our star is filled with a color.
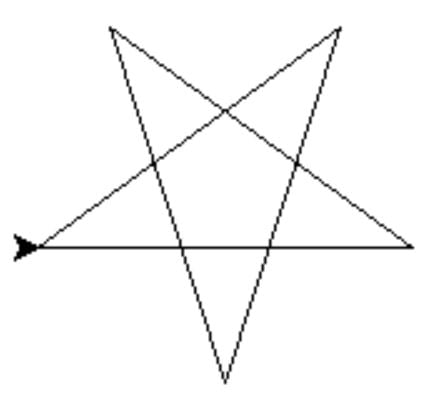
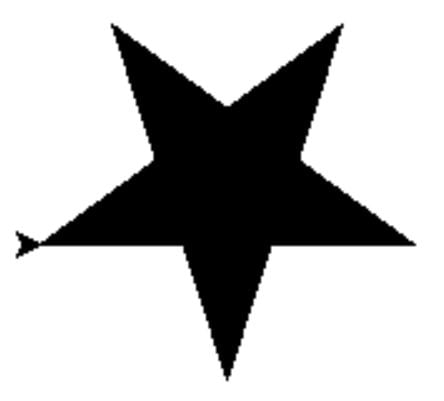
And another example, this time using .fillcolor() to pass a filling color value to turtle.
import turtle
turtle.fillcolor("orange")
turtle.begin_fill()
for i in range(5):
turtle.forward(150)
turtle.left(144)
turtle.end_fill()
turtle.exitonclick()
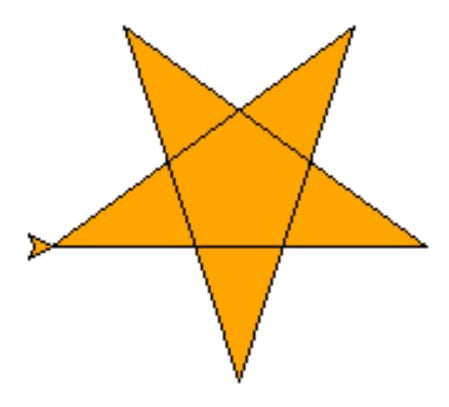
Example 2: Filling Triangles
Let’s try something different. By only adjusting the angle and iteration number we can get a perfect triangle with the same Python loop.
- We will adjust the angle to 120 (This is the external angle of an equilateral triangle since all three internal angles are 60 degrees.)
- We also need
range(3)
only so that it makes 3 iterations since a triangle has 3 sides.
import turtle
turtle.fillcolor("pink")
turtle.begin_fill()
for i in range(3):
turtle.forward(150)
turtle.left(120)
turtle.end_fill()
turtle.exitonclick()
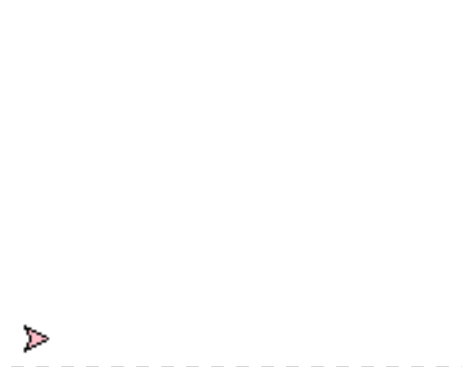
Example 3: Blurring an image
Great. Drawing and filling shapes with turtle is so much fun!
Let’s try something a little more advanced. We can spread a few of these triangles around the horizon using random library making them look like colorful tents.
You can also make them look like mountains by choosing more earthy colors.
To achieve this we will use:
turtle.speed(0)
to speed up the drawing.turtle.bgcolor("lightblue")
to create a pleasant background- For loop to draw triangles.
- A user defined function to put the first for loop (triangle maker) in it.
- We’ll call it with different random values to create different sizes of triangles.
- Another for loop to spread multiple tents at different locations
- Here we will also use:
random.randint()
method to randomize tent positions around an imaginary horizon.
- Here we will also use:
- Also before and after we will place the necessary fillcolor(), begin_fill() and end_fill() methods.
- We will use a list of predefined colors and random.randint() again to fill each tent with different colors.
It’s not that complicated but I wanted to explain as much as possible in case this Python tutorial is being used to teach an audience.
Let’s see the code and turtle drawing.
import turtle
import random
turtle.bgcolor("lightblue")
turtle.speed(0)
turtle.hideturtle()
def triangle_maker(i,j):
lst=["red","green","orange", "yellow","pink","brown","green"]
turtle.color("black")
turtle.fillcolor(lst[random.randint(0,6)])
turtle.begin_fill()
for iter in range(3):
turtle.forward(i)
turtle.left(j)
turtle.end_fill()
def tents():
for i in range(1):
triangle_maker(random.randint(65,125),120)
for i in range(6):
turtle.penup()
turtle.setpos(random.randint(-300, 300), random.randint(-20, 0))
turtle.pendown()
tents()
turtle.exitonclick()
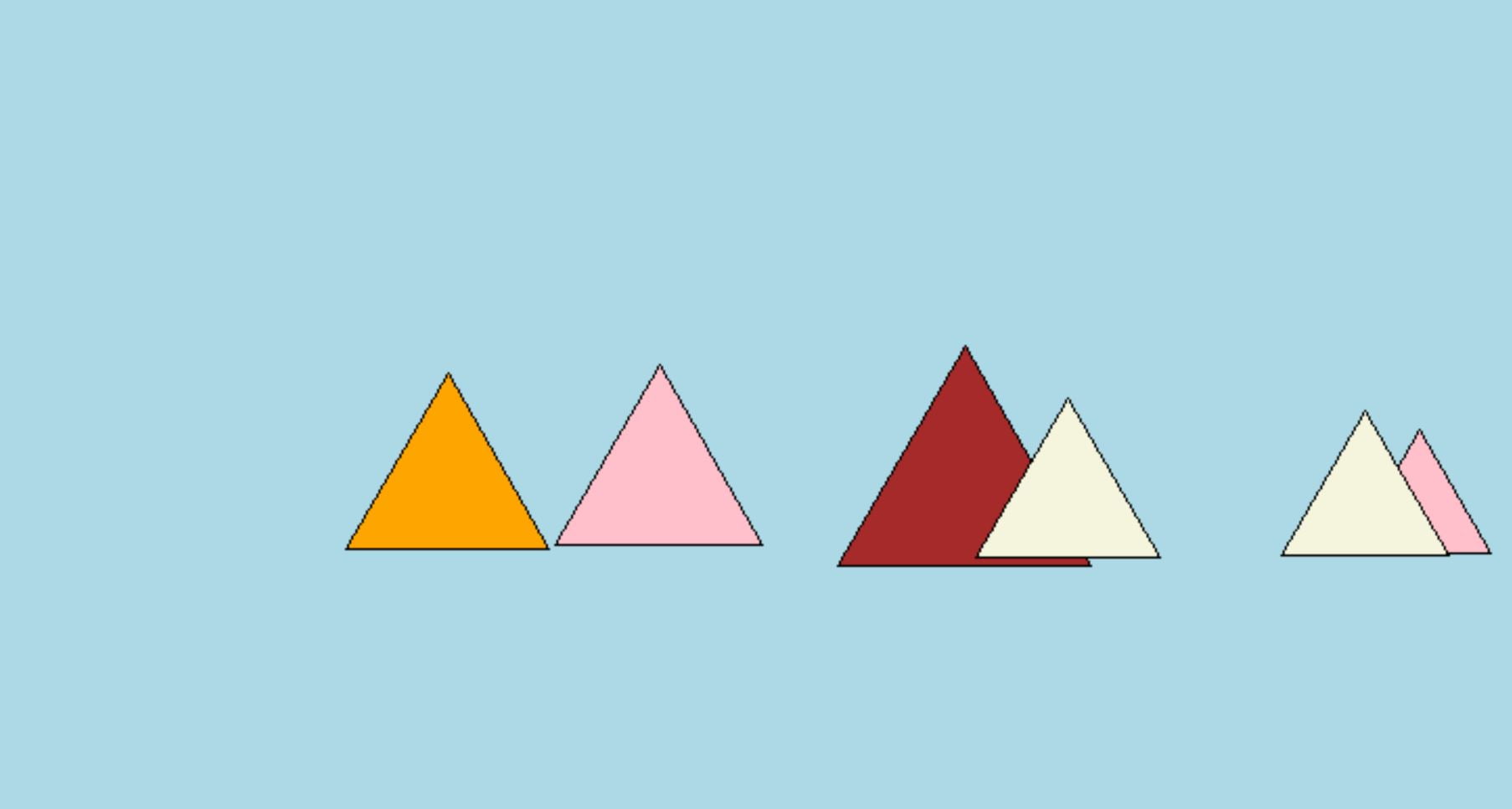
Not bad at all!
An important note: About python.color() position here. It matters if you place python.color() before begin_fill() or after begin_fill().
If you place it after begin_fill(), fillcolor() will be ignored (or supressed) and fill color will be the turtle drawing color and there won’t be any edge color.
So feel free to experiment with it, as long as you know that it matters if turtle.color() comes before or begin_fill() comes before you should be good.
Here is an example created with the code above just by changing swapping the .color, begin_fill positions as below.
#Only to demonstrate top three lines,
#which are swapped from previous Python code.
turtle.fillcolor(lst[random.randint(0,6)])
turtle.begin_fill()
turtle.color("black")
for iter in range(3):
turtle.forward(i)
turtle.left(j)
turtle.end_fill()
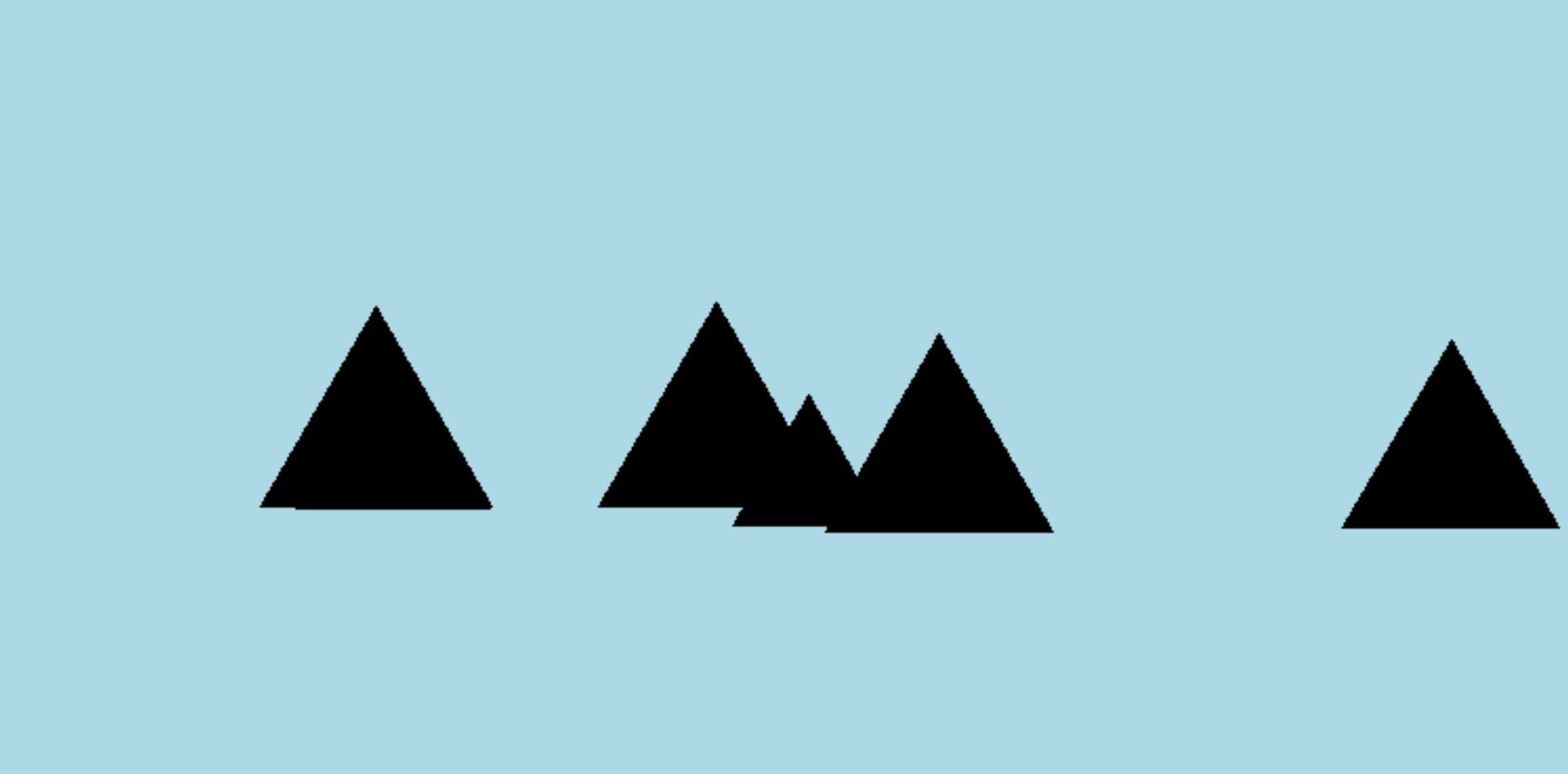
Summary
In this Python Tutorial, we have learned how to fill turtle drawings with color.
We have demonstrated the use of fillcolor(), begin_fill() and end_fill() with examples.
We have also seen a couple of relatively advanced methods to iterate through a list of colors to create shapes with multiple colors and iterate through a range to draw multiple objects.
We hope you enjoyed this Python turtle tutorial. Thank you.