Turtle Spirals
Drawing with Python Turtles can be a lot of fun! You can draw nice turbines with Python turtle with the codes in this tutorial.
We will explain how you can twist the code to give more flavor to your drawings and practice coding while drawing or vice versa, who knows 🙂
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
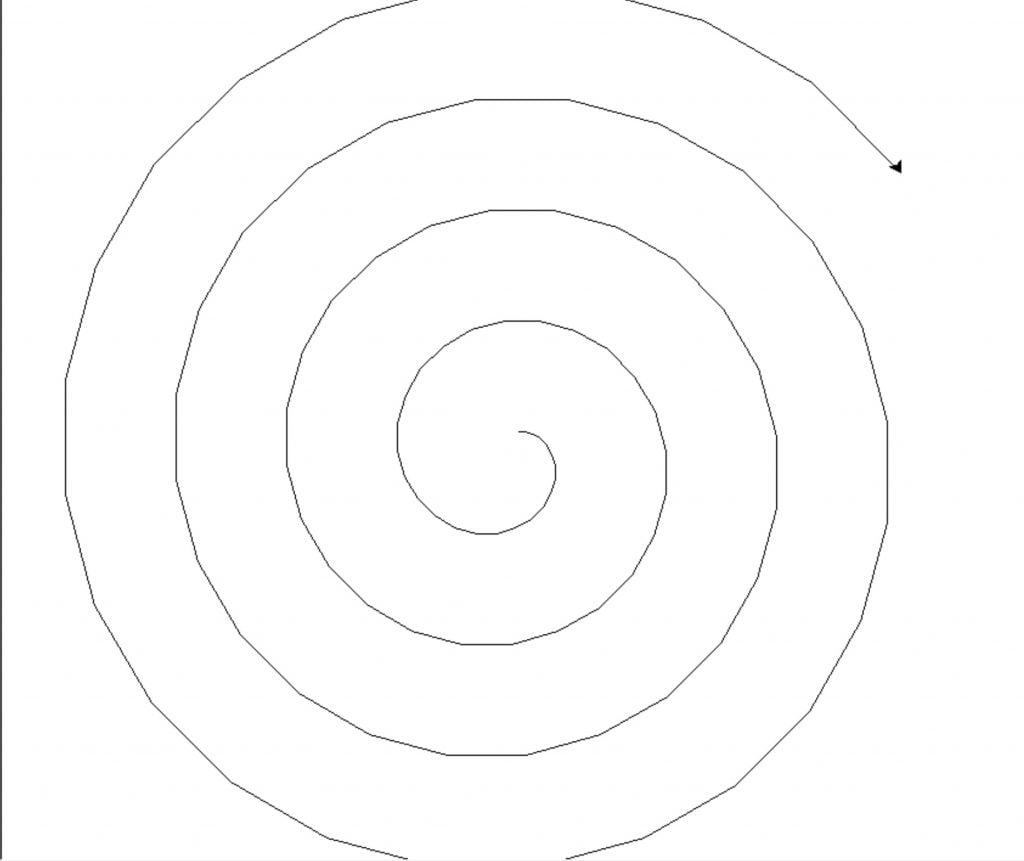
import turtle
a = turtle.Turtle()
for i in range(100):
a.forward(5+i)
a.right(15)
turtle.done()
Turtle Step and Direction Adjustment (forward, backward, left, right)
Let’s make the spiral more dense with decreasing the steps ( a.forward(2+i/4)
anda.left(30-i/12)
) and increasing the amount of turns (for i in range(240):
).
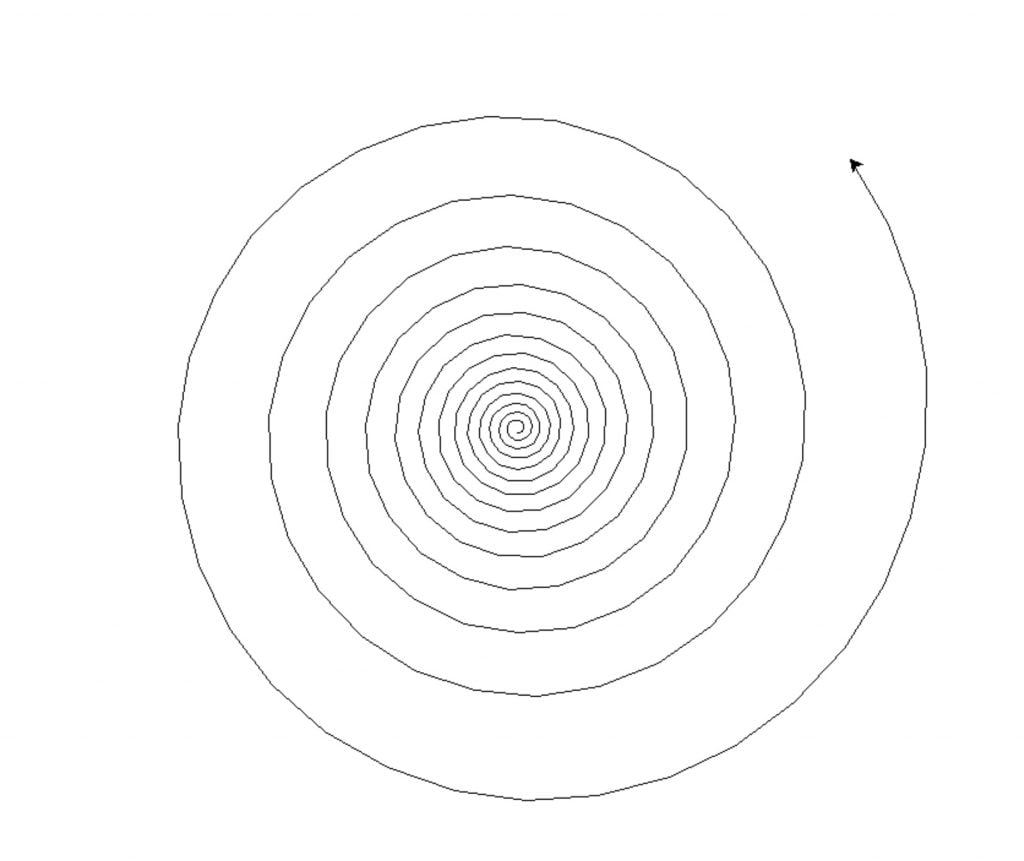
import turtle
a = turtle.Turtle()
for i in range(240):
a.forward(2+i/4)
a.left(30-i/12)
turtle.done()
Smaller Loop (Fibonacci Sequence)
Fibonacci sequence is an interesting number sequence, sometimes referred to as golden ratio, that can be traced in many natural patterns in universe such as flowers, shells, snails, trees, leaves, storms, galaxies and fingerprints.Â
Here is an attempt to draw on Fibonacci proportions. Not exactly it, but close.
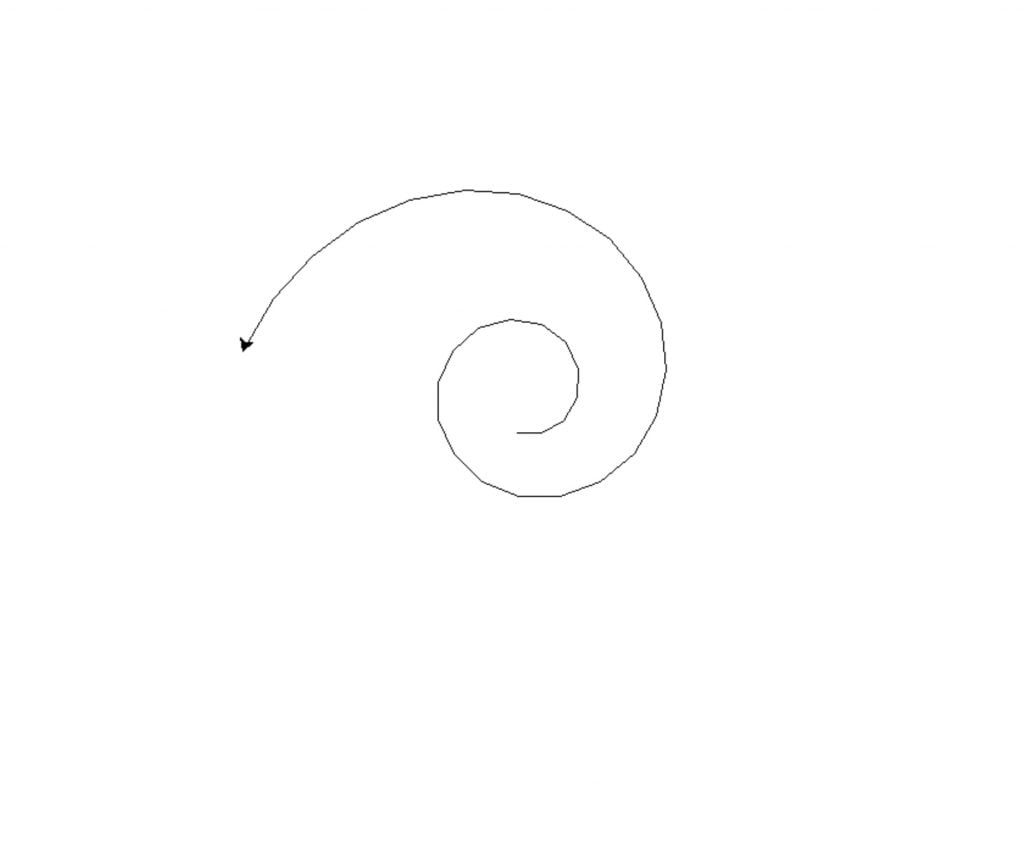
a = turtle.Turtle()
for i in range(30):
a.forward(20+i)
a.left(30 - i/1.5)
turtle.done()
Turtle with Colors
You can escape Black & White or Mono-Color drawings by implementing .color()
method of turtle.
Mono-color Turtle Drawing (Only implements one color throughout)
a.color(colors[0])
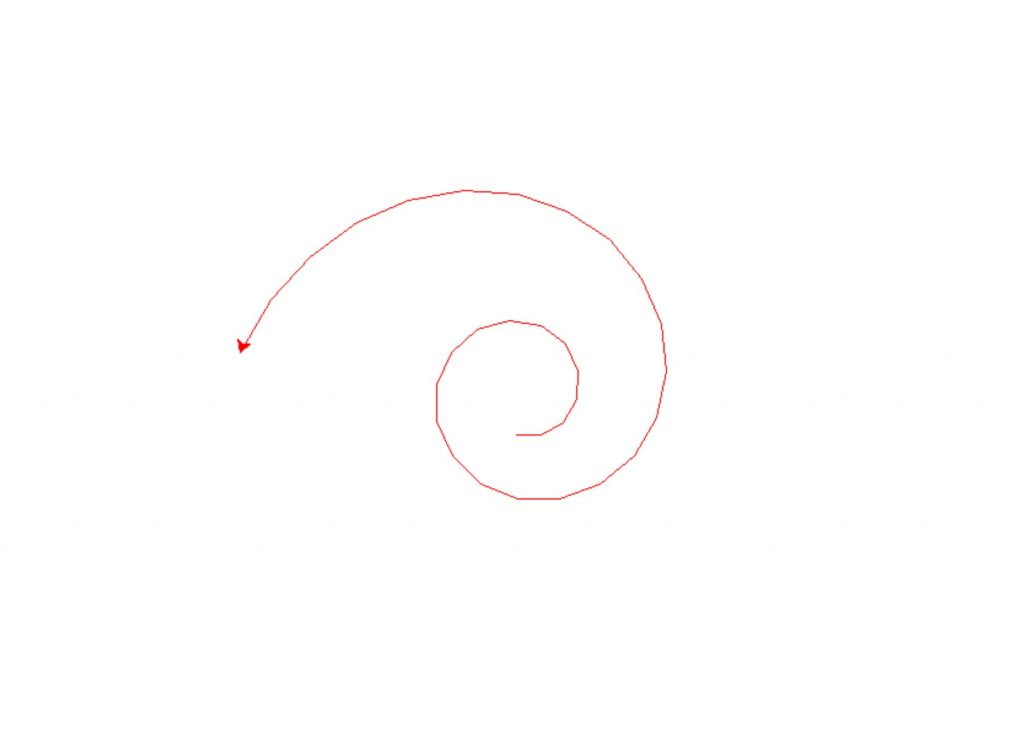
import turtle
colors = ["red", "blue", "green", "gray", "orange", "black"]
a = turtle.Turtle()
for i in range(30):
a.forward(20+i)
a.left(30 - i/1.5)
a.color(colors[0])
turtle.done()
Multi-color Turtle Drawing (Navigates through a list of different colors)
Another fun idea is iterating through different colors. This can be easily achieved by defining a set of color and some basic loop iteration with turtle.
Color list is iterated using the help of “Modulus” operator (%
) in Python. If you’d like to read an extensive article about Python operators including Modulus you can click here.
a.color(colors[i%6])
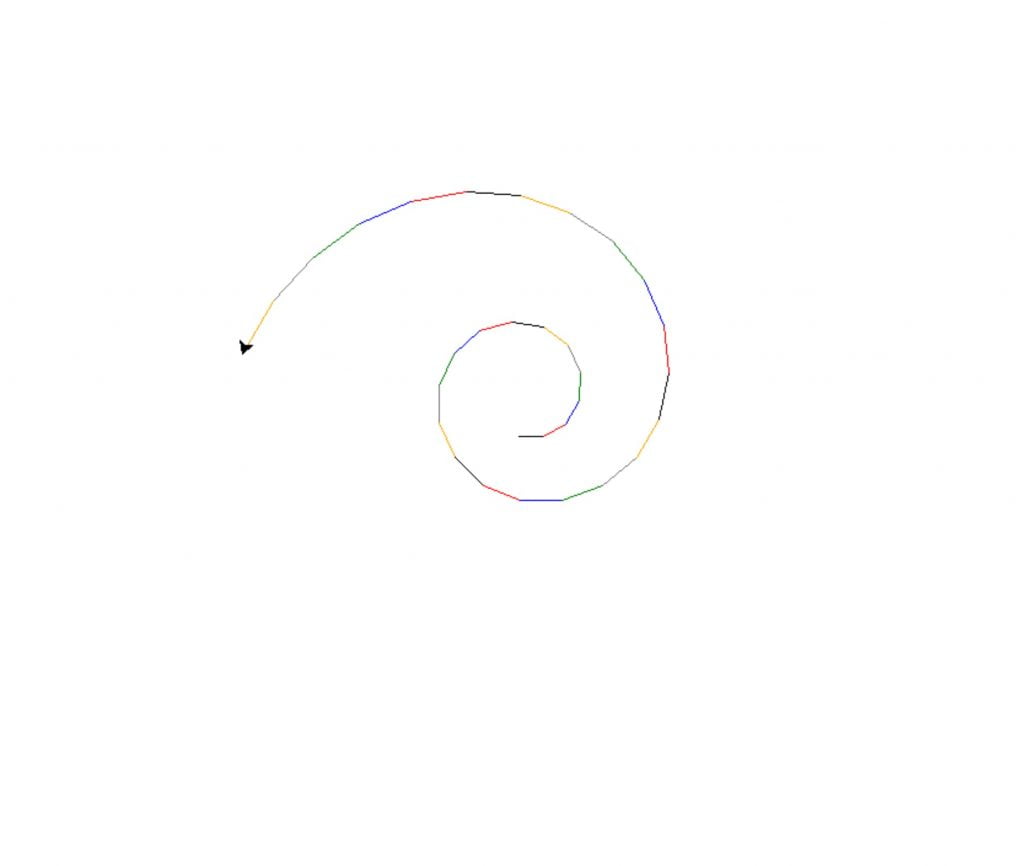
import turtle
colors = ["red", "blue", "green", "gray", "orange", "black"]
a = turtle.Turtle()
for i in range(30):
a.forward(20+i)
a.left(30 - i/1.5)
a.color(colors[i%6])
turtle.done()
That’s it. What you can do with Python Turtle is up to your imagination, so there is no limit. Try something that’s relevant to you and enjoy practicing!
If you find turtle interesting we have a very extensive tutorial that explains different Python concepts (such as if-else, user functions, user input, operators, data types, loops etc.) through turtle here: Python Turtle Tutorial.
ps: Don’t forget to include turtle.done()
in the end so your turtle window can be terminated.
pss: We recommend Spyder IDE which comes with Anaconda Open Source All-in-One installation solution. Although it’s an IDE specialized in scientific applications it’s also perfect for Python practice. You can read more about effortless Python Installation here.