How to run Python Code directly from the OS terminals
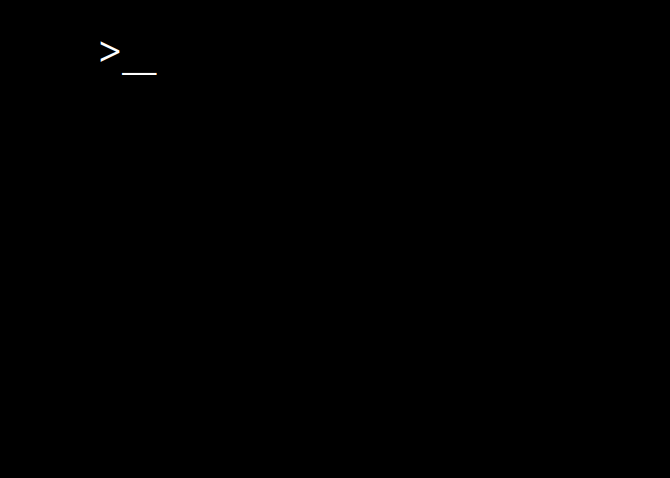
Python is a high-level, multipurpose programming language that’s also suitable for scripting. It’s high functionality per character makes it a perfect candidate for quick implementations that can be executed directly from operating system terminals using shell programs such as bash, zsh, cmd.exe or PowerShell.
So, is it possible to run Python code from an os shell such as bash or PowerShell? The answer is: Yes!
In this article, we will demonstrate the usage of Python code directly from the shell without even entering the Python Interpreter which can be a hassle sometimes for executing quick Python operations.
Let’s dive in and start checking out a few examples after defining the fundamentals…
What is Python code?
Python code simply is a text file which consists of object-oriented input (which can be functions, data, variables, statements etc.) based on Python’s syntax (rules and nuances of the language).
To read an in-depth analysis of what Python code is and how Python code works. You can review our article: What is Python code? In that article you can learn about the exact mechanisms that make Python code possible and Python’s evolution throughout the years as well as a few world-class enterprises that make use of Python code.
What is the Python interpreter?
Python interpreter is the program that interprets Python code written with Python’s syntax resulting in direct execution of the instructions in the code by the computer.
Interpreters don’t require additional compiling prior to running the program which makes Python more practical in most cases.
Standard Python Interpreter is written in Python language itself as well as C programming language which has useful libraries that translate Python code to a language that can be directly understood by the machine/computer called bytecode.
What is a terminal, shell & console?
Terminal is an interface where shell can be accessed. Although in today’s world terminal almost always refers to the GUI software that emulates a terminal installed on an operating system, in the 70s terminals were hardware devices what were used for entering data and programs and displaying related output.
Before terminals with screen, computer programs were executed through cards and paper. The actual name of the print function in programming languages comes from the tradition of printing output on paper after a computer program is successfully executed. These devices were called teleprinters or teletypes or tty for short.
First teletypes with screens as known as glass teletypes started hitting the market in mid-60s and the rest is history.
Today, terminal and console are also usually used interchangeably as consoles are virtual implementations where terminals run.
Shell on the other hand is the program that’s executed in the terminal. Sh (Bourne Shell), Bash (Bourne Again Shell), Zsh (z shell), PowerShell and cmd are the most popular shells used by operating systems today.
Console is another term with hardware connotations. It is used to cover the entire input/output device network of a computer such as keyboard and the monitor. Similarly if you have heard of the concept of “game consoles” it is used to refer to both physical hardware and software of video game platforms such as Xbox and Playstation.
However the Virtual Console (named tty in Linux) implementation in operating systems made this term pretty much synonymous with terminals or Virtual Terminals.
Long story short terminal, console, command line, command line interpreter (CLI), command line prompt and shell are often used interchangeably today and although they technically mean different things their synonymous usage by the end-user is not a very problematic approach in most cases. For the majority of coders and programmers all of these computation terms indeed serve the same function.
>_
* CLI (command-line interpreters) is a white text on black background environment that can execute/interpret commands through an input line.
Method 1: Executing Python code in terminal via Python -c
Python comes with an optional argument “-c” which allows a string to be passed to the Python interpreter directly from the command line without creating or saving any files.
This can be greatly useful for executing small Python scripts or practicing Python on the go in your local machine. All that’s needed is a terminal such as bash, zsh, CMD.exe or PowerShell. Then you can utilize python -c as following.
python -c <Python command>
Check out the examples below.
Various python -c
Examples
1) 2) 3)
Terminal Command
python -c 'print("Hello World!")'
python -c 'print(2**7)'
python -c "import math; print(math.factorial(10))"
Output
Method 2: Executing Python code in bash via <<< operator
This method is rather specific to bash command line environment.
This bash-specific operator has a couple of names. Triple left chevron or “Here String” operator (<<<
), works similar to python -c
command.
You can pass a string after python <<<
and the following Python command or Python script get executed directly from bash or a bash emulator without necessarily entering the Python interpreter itself. Check out our examples below regarding the “Here String” or <<< operator.
Various python <<<
Examples
Example 1: Opening a web page using Python in bash terminal
python <<< 'import webbrowser; webbrowser.open('google.com')'
Output:
Example 2: Getting Python's current working directory (cwd) in bash
python <<< 'import os; print(os.getcwd())'
Output:
Example 3: Converting hex to RGB using PIL directly in bash
python <<< "from PIL import ImageColor; print(ImageColor.getrgb('#ffffff'))"
Output:
Example 4: Converting string to hex using Python in bash
python <<< "print(b'ARTEMIS'.hex())"
Output:
Capital English letters are represented with two digit numbers in the hexadecimal number system. You can find below the hex to ascii conversion table and validate the conversion in the example above with 2-digit groups such as:
0x41//0x52//0x54//0x45//0x4d//0x49//0x53
(0x prefix denotes the digits are in hexadecimal number system)
41 | A |
42 | B |
43 | C |
44 | D |
45 | E |
46 | F |
47 | G |
48 | H |
49 | I |
4a | J |
4b | K |
4c | L |
4d | M |
4e | N |
4f | O |
50 | P |
51 | Q |
52 | R |
53 | S |
54 | T |
55 | U |
56 | V |
57 | W |
58 | X |
59 | Y |
5a | Z |
* Capital English letters Ascii - hexadecimal conversion table
Summary
In this Python Tutorial we learned how to run Python code directly from computer terminals and shells such as Windows PowerShell, BASH, Terminal and Zsh.
We also covered a few definitions to solidify what Python code is and Python code works. Finally we explained a few concepts that are involved in running Python code such as terminal, shell, console, command line and Python interpreters.
We demonstrated a few cool Python codes that are practical and suitable for running in terminal environments.
If you’d like to fish for ideas or inspiration for Python codes to run on your operating system’s terminal, you can check out our 100 Python Tips & Tricks article.