How to download Youtube videos with Python
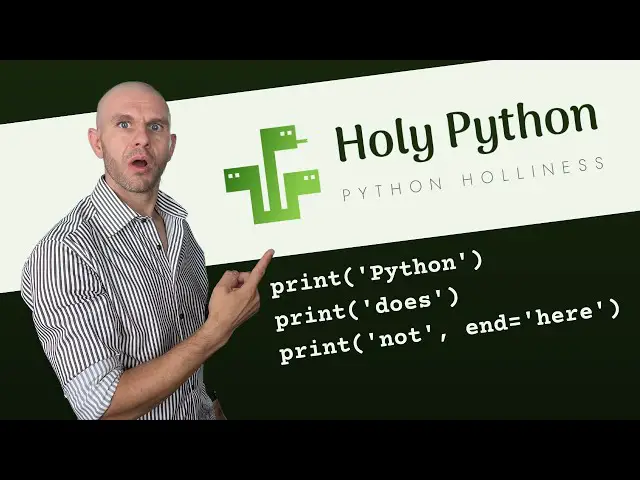
It’s been 17 years since Youtube founder Jawed Karim uploaded the first ever Youtube video titled: “Me at the zoo“.
The famous video is a 776 kilobyte tongue-in-cheek masterpiece where Jawed admires the trunks of the elephants in the background and history was made right there and then at the San Diego Zoo.
- learn
- exercise
- do makeup
- listen music
- make money
- fix things
- build things
- promote brands
- play video games
- share information
- share experiences
- consume entertainment
- watch (and not watch!) TV
- make purchasing decisions
- broadcast live events, news & shows
- ultimately, the way we live our lives…
Youtube Stats as of 2023
What is PyTube?
Pytube is an open-source Python library which can be used to download YouTube videos effortlessly. It has great documentation and straightforward Python functions and methods which makes usage a pleasant experience.
Using Pytube, you can filter various formats such as different resolutions, including 360p, 480p, 720p, and 1080p, filter based on audio-only streams, get titles and thumbnails of Youtube videos.
PyTube can also be used by educators and professors to create assignments, projects and examples either for teaching core capabilities of Python or more advanced
Python Desktop Applications (with frameworks such as PyQt, PySide, or wxPython), Python Web Applications: (Django or Flask), Python Mobile Applications (Kivy or BeeWare). If professionally made, these applications can also be sold on app stores like Apple App Store or Google Play or monetized through premium versions or subscription (see: How to make money using Python).
How to install PyTube
?
Pytube is quite lightweight and it can be installed using pip via the simple command below:
pip install pytube
Then, you can start experimenting with the PyTube library with Python as below.
How to download Youtube videos using Python?
Please note that this tutorial is for educational purposes only. By downloading YouTube videos without explicit permission from the video owner(s) and/or YouTube may be a violation of YouTube’s terms of service and potentially related copyright laws. If you know you have the legal right to download a YouTube video, then Python is a great option to specify different video parameters and download Youtube videos.
We will demonstrate this Python tutorial with various PyTube examples below.
Example 1: Downloading Youtube Video Titles video.title
- import necessary library/module
- create a video object
- call the
.title
method on the video object.
from pytube import YouTube
We need to start with importing the necessary libraries and/or modules as usual. Above we are importing the YouTube module from the pytube library in Python. You can scroll up to see “how to install the pytube library?” if you’re missing it.
When working with PyTube, first we need to create a video object (or Youtube video object if you will). That’s easy. We can create one as following:
url = "https://www.youtube.com/watch?v=5Iw6X5IDNC4"
video = YouTube(url)
All we are doing here is using the YouTube function from Pytube library and passing a Youtube video link to it as a string object.
Python variable named video is now our video object. Now, we can call the .title
method on it.
video_title = video.title
print(video_title)
Python print – [tricks you need to know]
Great job. Now we can explore another method. This time we will acquire the thumbnail url of a Youtube video.
Example 2: Downloading Youtube Video Thumbnails video.thumbnail_url
Getting a thumbnail url of a video can be useful for various reasons. If you are creating an app, you might want to show the user the thumbnail of the video they are dealing with. Additionally, some videos feature a thumbnail image where the image comes from external source and is never part of the video it represents.
Here is how you can acquire url of a Youtube video’s thumbnail:
Repeat Step 1 & Step 2 as in Example 1 above so you have a video object.
On that video object call the thumbnail_url
method.
from pytube import YouTube
url = "https://www.youtube.com/watch?v=5Iw6X5IDNC4"
video = YouTube(url)
video_thumb = video.thumbnail_url
print(video_thumb)
‘https://i.ytimg.com/vi/iwLM1s8MJhk/sddefault.jpg’
thumbnail_url method returns the link above and the link returns the thumbnail image below:
Now let’s see how to download Youtube videos using the pytube library.
Example 3: Downloading Youtube Videos video.download
get_highest_resolution()
method on this video object.We will narrow down from our video’s various formats and resolutions. Let’s call this new variable a stream and then let’s print the stream object and see what it actually contains.
from pytube import YouTube
url = "https://www.youtube.com/watch?v=5Iw6X5IDNC4"
video = YouTube(url)
via get_highest_resolution()
stream = video.get_highest_resolution()
print(stream)
<Stream: itag=”22″ mime_type=”video/mp4″ res=”720p” fps=”24fps” vcodec=”avc1.64001F” acodec=”mp4a.40.2″ progressive=”True” type=”video”>
stream.download()
From the output we can read that, the highest resolution that’s available for download for our video is 720p. We also see various characteristic of the video such as:
- frame per second – fps : “24fps”
- video codec – vcodec : “avc1.64001F”
- audio codec – acodec : “mp4a.40.2
- stream no : #22
Congrats! After running the .download() method as above, the chosen stream of your video will get downloaded on your current Python working directory.
via get_by_itag()
Alternatively, you can use get_by_itag() method to specify the stream by resolution options or its audio settings.
For example, 140 is a specific itag number which signifies 128 kbps audio-only stream of our video.
from pytube import YouTube
url = "https://www.youtube.com/watch?v=5Iw6X5IDNC4"
video = YouTube(url)
stream = video.streams.get_by_itag(140)
stream.download()
Pytube errors:
AttributeError: ‘StreamQuery’ object has no attribute ‘filters’. Did you mean: ‘filter’?
Please note, if your connection or the server suffers from a connection error you might receive an errors similar to following:
socket.gaierror: [Errno -3] Temporary failure in name resolution
urllib.error.URLError: <urlopen error [Errno -3] Temporary failure in name resolution>
You can wait about a minute and try again, in my experience it never lasted more than a minute so hopefully you won’t experience it for long durations either.
How to get appropriate itag numbers?
You can wait about a minute and try again, in my experience it never lasted more than a minute so hopefully you won’t experience it for long durations either.
itags = video.streams.filter()
for i in itags:
print(i)
<Stream: itag="17" mime_type="video/3gpp" res="144p" fps="12fps" vcodec="mp4v.20.3" acodec="mp4a.40.2" progressive="True" type="video">
<Stream: itag="18" mime_type="video/mp4" res="360p" fps="24fps" vcodec="avc1.42001E" acodec="mp4a.40.2" progressive="True" type="video">
<Stream: itag="22" mime_type="video/mp4" res="720p" fps="24fps" vcodec="avc1.64001F" acodec="mp4a.40.2" progressive="True" type="video">
<Stream: itag="313" mime_type="video/webm" res="2160p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="271" mime_type="video/webm" res="1440p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="137" mime_type="video/mp4" res="1080p" fps="24fps" vcodec="avc1.640028" progressive="False" type="video">
<Stream: itag="248" mime_type="video/webm" res="1080p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="136" mime_type="video/mp4" res="720p" fps="24fps" vcodec="avc1.64001f" progressive="False" type="video">
<Stream: itag="247" mime_type="video/webm" res="720p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="135" mime_type="video/mp4" res="480p" fps="24fps" vcodec="avc1.4d401e" progressive="False" type="video">
<Stream: itag="244" mime_type="video/webm" res="480p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="134" mime_type="video/mp4" res="360p" fps="24fps" vcodec="avc1.4d401e" progressive="False" type="video">
<Stream: itag="243" mime_type="video/webm" res="360p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="133" mime_type="video/mp4" res="240p" fps="24fps" vcodec="avc1.4d4015" progressive="False" type="video">
<Stream: itag="242" mime_type="video/webm" res="240p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="160" mime_type="video/mp4" res="144p" fps="24fps" vcodec="avc1.4d400c" progressive="False" type="video">
<Stream: itag="278" mime_type="video/webm" res="144p" fps="24fps" vcodec="vp9" progressive="False" type="video">
<Stream: itag="139" mime_type="audio/mp4" abr="48kbps" acodec="mp4a.40.5" progressive="False" type="audio">
<Stream: itag="140" mime_type="audio/mp4" abr="128kbps" acodec="mp4a.40.2" progressive="False" type="audio">
<Stream: itag="249" mime_type="audio/webm" abr="50kbps" acodec="opus" progressive="False" type="audio">
<Stream: itag="250" mime_type="audio/webm" abr="70kbps" acodec="opus" progressive="False" type="audio">
<Stream: itag="251" mime_type="audio/webm" abr="160kbps" acodec="opus" progressive="False" type="audio">
Filter mp4 files only
itags = video.streams.filter(file_extension='mp4')
for i in itags:
print(i)
Filter 3gpp files only
itags = video.streams.filter(file_extension='3gpp')
for i in itags:
print(i)
Filter audio-only streams
itags = video.streams.filter(audio_only=True)
for i in itags:
print(i)
Evolution of media
Around 45,000 years ago, ancient humans painted what is now recognized as the oldest known cave art in Indonesia, named Warty Pig. These early artists observed, processed, and transformed information into vivid visual representations using techniques and mediums available to them at the time. The resulting cave paintings provide a unique glimpse into the world of our ancestors and the ways in which they communicated and expressed themselves.
Admittedly, old communication ways of information come across as extremely primitive and low-bandwidth compared to today’s standards. Today, Youtube accommodates 8K 120 fps videos accompanied with 360 VR features that give extreme level of detail and visual and auditory immersion, which was unimaginable just a few decades ago.
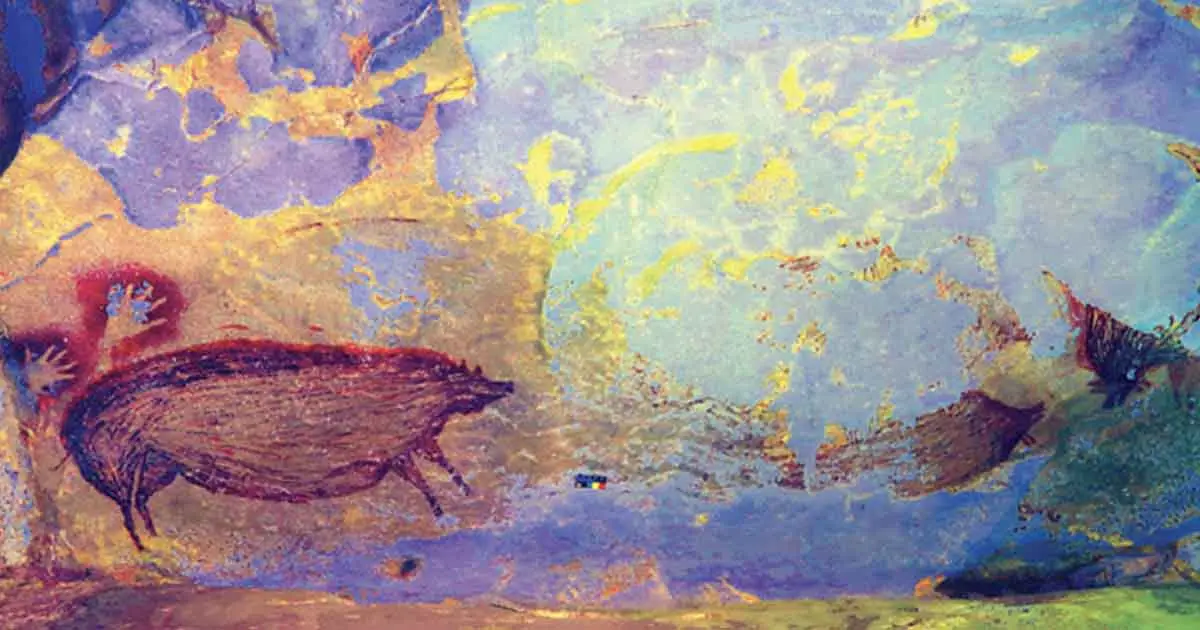
Reflecting on the historical evolution of how information has been represented throughout human history can provide us with a valuable perspective that emphasizes just how unique and transformative our current era truly is.
In the latest end of that transformation let’s look at a few fun facts about one of the heroes of the last decade, Youtube.com.
Youtube Fun Facts
1- Legal Quagmire with Utube.com
There has been a legal conflict between YouTube and Universal Tube & Rollform Equipment Corporation, a company that owns the domain name utube.com. In 2006, Universal Tube & Rollform Equipment Corporation filed a lawsuit against YouTube, claiming that the similarity between the domain name utube.com and the YouTube domain name caused confusion among customers and led to a significant increase in traffic to the utube.com website.
The lawsuit argued that the increased traffic overwhelmed the company’s servers, causing it to lose business and incur significant expenses. Universal Tube & Rollform Equipment Corporation sought to force YouTube to change its name or pay damages for the alleged infringement.
However, the court ruled in favor of YouTube, stating that the similarity between the two domain names was not sufficient to establish trademark infringement. The court also noted that Universal Tube & Rollform Equipment Corporation had made no effort to actively promote or advertise its website prior to the launch of YouTube, and that any increase in traffic was likely due to unrelated factors. The case was ultimately dismissed.
If you’re interested in gaining legal knowledge related to tech and particularly programming, you can check out our post on software licenses for further insight and guidance.
2- Greatest Media M&A of All Time
Only 18 months after being founded, Youtube was acquired by Google for $1.65 billion. Although it sounded like a massive amount of money to pay for acquiring a video sharing website, $1.65 billion sounds like a deep discount today considering Morgan Stanley valuation of Youtube brand was $160 billion in 2018 and another article suggests Youtube was already worth $500 billion in 2022.
3- First Videos to Hit Record Views
1M Views
Soccer superstar Ronaldinho’s video (in collaboration with Nike) was the first to hit 1M views in October 2005.
100M Views
Alternative pop singer Avril Lavigne was first to hit 100M views. Avril Lavigne had quite a few big hits in the years after Youtube was found which incorporated elements of punk and skater culture.
1B Views
Gangham Style by Korean pop and dancing star was the first video to hit 1 billion views on Youtube.
10B Views
Baby Shark Dance is the first and only video (as of 2023) to hit 10 billion views.
4- Tony Blair enters the scene
British Prime Minister Tony Blair was the first world leader to join the platform as he opened his Youtube account in 2007.
Summary
vid = "https://www.youtube.com/watch?v=5Iw6X5IDNC4"
vid = "https://www.youtube.com/watch?v=jNQXAC9IVRw"
yt = YouTube(link)
mtitle = yt.title
yt = yt.streams.get_highest_resolution()
#ytt = yt.streams.get_by_itag(140)
for i in range(1):
print("\n\nYour video: {} is being downloaded".format(mtitle))
try:
yt.download()
except:
print("An error has occurred")
print("\n\nYoutube video is downloaded successfully.")
In this Python Tutorial we learned how to download Youtube videos using PyTube library and Python. We also covered how to install PyTube using pip Python package manager and various parameters you can use when downloading Youtube videos with Python such as video quality, video format and various audio-only options.
Additionally, you can only get thumbnails of Youtube videos as well as titles of Youtube videos which we have demonstrated using Python code examples.
Summary
In this Python Tutorial we learned how to download Youtube videos using PyTube library and Python. We also covered how to install PyTube using pip Python package manager and various parameters you can use when downloading Youtube videos with Python such as video quality, video format and various audio-only options.
Additionally, you can only get thumbnails of Youtube videos as well as titles of Youtube videos which we have demonstrated using Python code examples.