If you’re looking to take your coding journey to next level, or if you’re specifically looking for a leap into GUI, jackpot! I will share with you the nicest, coolest, most practical GUI solution for Python.
I really think GUI adds a new dimension to the code and the coding as a learning journey. This can help you step up your programming in a way that it offers so many new benefits directly and indirectly!:
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
- Your code doesn’t look like some weird output that’d only be used in Matrix triology.
- You can have your program used via:
- radio buttons
- checklists
- menus
- dropdown lists
- value wheels
- value bars etc.
- You can actually call your code a program
- You can tap into a new source of imagination since things start getting visual
- You can decide on the level of GUI (you don’t have to get super visual, it’s up to you and your program)
- More opportunities to have your code used by a wide range of people from different backgrounds.
- Pretty graphs
- Games
- Monetization of your code / program / software (You can actually create a product or service that can be marketed and sold)
Estimated Time
15 mins
Skill Level
Intermediate
Important Parameters
.Button, .Window
.Text, .T, .theme
Libraries
PySimpleGui
GUI is a huge step forward in anyone’s coding journey.
I think if we consider a cost/benefit ratio for GUI methods where cost is the learning effort and time and benefit is the potential outcome opportunities, I must say ratio is very very high.
GUI adds a whole new dimension to coding that enables you to create buttons, menus, lists, checklists, messages, boxes, layout design etc. This obviously makes your code actually usable by a marginally larger demographics but I’d like to argue that benefits are even bigger than just other people being able to use your code.
When you start creating GUI applications, you might start to realize that you’re tapping into a new creativity resource with that visual experience. Albert Einstein says: Imagination is everything. GUI sort of boosts imagination and lets you get hand-onsy with code.
Now historically, GUI also had limitations and there were also limited amount of libraries. This could make GUI learning curve pretty steep for Pythonistas.
This doesn’t have to be the case. In this article We’re going to share with you the most straight-forward, practical GUI technology that you can start implementing right away without losing your precious hair.
Let’s get started.
Simple GUI Example (Getting Started)
Let’s create a layout and a button in it. We’ll do this in 4 baby steps:
- We’re gonna import the necessary library
- We’ll create a layout in list format with brackets: [ ]
- We’ll use
sg.Button()
method and put it inside the layout. - Then we’ll create a window using
sg.Window()
which takes- a title string
- layout object
1- Import the PySimpleGUI library:
If you don’t have PySimpleGUI library you can simple install it by executing the following command in Anaconda Command Prompt (or Command Prompt):
pip install pysimplegui
import PySimpleGUI as sg
2- Create a layout with a button in it.
layout = [[sg.Button('Hello World')]]
3- Create the window with a title and the layout we created earlier:
window = sg.Window('Push my Buttons', layout)
4- Reading the window to make it show up:
event, values = window.read()
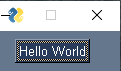
You’ll get a window like this. Now, we’ve already created the first GUI application. Let’s build up the tutorial for a few more interesting points.
GUI Size Settings (Layout Size, Button Size, Font Size etc.)
You can simply add tuples of size parameters to both the sg.Button
and sg.Window
.
It works so: size(width, height)
Here is the full code so far with a couple of size parameters added:
import PySimpleGUI as sg
layout = [[sg.Button('Hello World', size=(20,4))]]
window = sg.Window('Push my Buttons', layout, size=(200,150))
event, values = window.read()
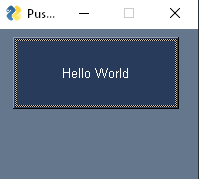
Boom! Our GUI window is instantly starting to look better. But there are more cool tricks to check out.
GUI Alignment Tricks (Row Alignment, Column Alignment)
You can simply add sg.T
or sg.Text
methods to add a text or add blank strings to move the buttons Left and Right:
layout = [[sg.Text("Primary Button: "), sg.Button('Hello World',size=(20,4))]]
window = sg.Window('Bunch of Buttons', layout, size=(270,100))
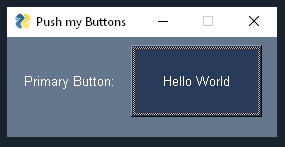
It looks like this.
So, here is the trick, inside the same bracket we’re altering the same row. Create new brackets and you’ll be creating new rows which can be used to alter column alignment. Let’s use sg.T this time and a string with blanks to create space above the button:
Note: Also pay attention to the windows size we’re changing that to create a more suitable window for the layout.
layout = [[sg.T("")], [sg.Button('Hello World',size=(20,4))]]
window = sg.Window('Push my Buttons', layout, size=(210,150))
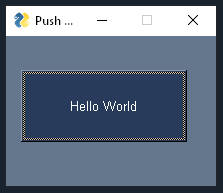
Now it looks like this. Including the text inside a new bracket created a new row above the button.
Themes (Prettify GUI real quick)
PySimpleGUI has great color themes that you can apply to your program to make things a little more colorful (or sometimes less)
These theme colors are really well prepared and makes styling so much easier.
We will use sg.theme()
method to implement various colors to the GUI window.
I find reddit color scheme really sleek. Let’s take a look at that:
import PySimpleGUI as sg
sg.theme('Reddit')
layout = [[sg.Text("Primary Button: "), sg.Button('Hello World',size=(20,4))]]
window = sg.Window('Push my Buttons', layout, size=(270,100))
event, values = window.read()
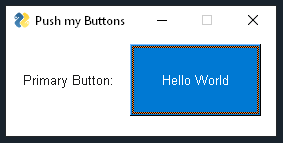
Here is a look at LightYellow, DarkBlue15, Tan, DarkTeal2, Topango, LightBrown5.
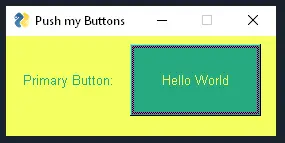
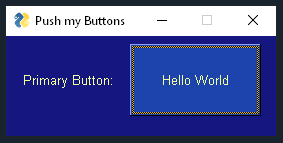
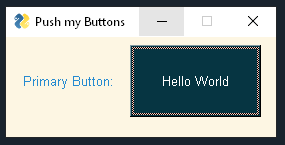
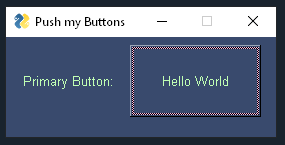
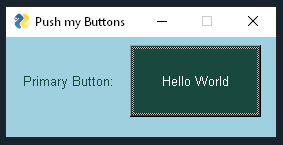
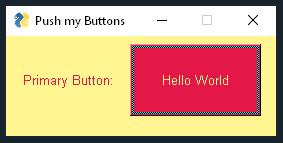
Here are some more ideas to check out:
Black
TealMono
TanBlue
LightGreen1
LightGreen2
LightGreen3
LightGreen4
DarkBlue15
Tan
SystemDefault
LightBlue5
LightBrown
DarkPurple
DarkGreen
LightPurple
LightGrey
Green
Dark
BrownBlue
BrightColors
BlueMono
BluePurple
DarkRed
DarkBlack
How to tie buttons to functions? (Action time)
Now, if you’re creating buttons you’ll likely want them to execute something. That’s the whole point right. We can simply create user defined functions or map Python’s builtin functions to the buttons we create with the PySimpleGUI library.
So, mapping buttons to functions is not that complicated. You just need some basic loop knowledge in Python.
Please feel free to these materials if you need to polish your For Loop and While Loop knowledge.
while True:
event, values = window.read()
if event == sg.WIN_CLOSED:
break
elif event == 'Hello World!':
print("Hello World!")
So what’s going on in this code snippet?
- Firstly, everything is structured inside an infinite while loop. This way GUI interface is continuously monitored and executions are carried out when needed.
- GUI windows is being monitored with
.read()
method. This means every time a button is pressed or a selection is made those values can be interpreted by the program. - if statement ensures that program is closed when close button is pressed. This is achieved by break statement. If you need a primer on that we have a great lesson focusing on break statement and its cousin try/except statement can be useful as well.
- elif statements manage each action that needs to be done when event equals buttons name. This is stated as:
elif event == "Hello World!":
Closing Thoughts (Conclusion)
That’s about it. Now, regarding a GUI application you know how to create these:
- layout,
- window,
- buttons,
- text(s),
- resize GUI window, button and text
- align GUI objects vertically and horizontally
- spice up the color scheme with PySimpleGUI themes
- read from the GUI application
- and map buttons to functions or various code.
You can apply this knowledge to create multiple buttons and text as well as other interesting object that’s usually included in software such as:
- sliders
- radio buttons
- checklist buttons
- dropdown menus
- forms etc.
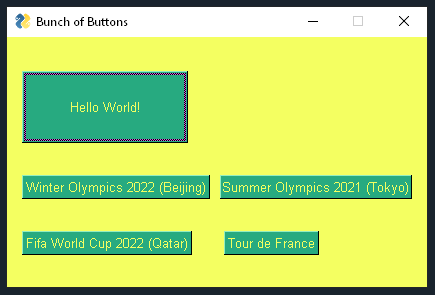
And here is the full code:
import PySimpleGUI as sg
sg.theme('LightYellow')
layout = [[sg.T("")],[sg.Button('Hello World!',size=(20,4))],[sg.Text(" ")],
[sg.Button('Winter Olympics 2022 (Beijing)'), sg.Button('Summer Olympics 2021 (Tokyo)')],[sg.Text(" ")],
[sg.Button('Fifa World Cup 2022 (Qatar)'), sg.T(" "*2),sg.Button('Tour de France')]]
window = sg.Window('Bunch of Buttons', layout, size=(420,250))
event, values = window.read()
##########Button Functions##########
while True: # Event Loop
event, values = window.read()
if event == sg.WIN_CLOSED:
break
elif event == 'Hello World!':
print("Hello World!") # call the "Callback" function
elif event == 'Winter Olympics 2022 (Beijing)':
print("Winter Olympics 2022 (Beijing)")
elif event == 'Summer Olympics 2021 (Tokyo)':
print("Summer Olympics 2021 (Tokyo)")
elif event == 'Tour de France':
print("Tour de France")
elif event == 'Fifa World Cup 2022 (Qatar)':
print("Fifa World Cup 2022 (Qatar)")
You can find this code in HolyPython Github repository. You can also find the official repository page of PySimpleGUI library here.
I’m so happy to create this tutorial because it can really help people from all backgrounds step up their programming skills and outcomes as a result of it. It also takes 5-10 minutes to learn and maybe a couple hours to master. Then you have a whole new skill-set to make use of your basic programming skills.
This was a simple GUI tutorial for Python using PySimpleGUI library.
You can also check out this tutorial about Task Scheduling with Python’s .py files which goes hand in hand with GUI applications sometimes.
Please share this post if you found it useful. Thank you!