Python Try / Except Statements
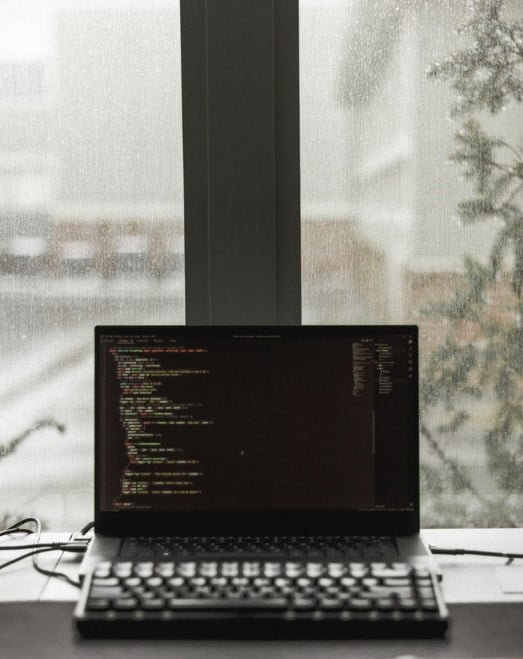
Try / Except statements are great methods for overcoming errors in your code.
The way it works is very simple.
- try statement gets tried. If there is no exception it runs and except statement will be ignored.
- If there is an exception, try statement won’t be executed and your code will skip to except part to execute it.
It will be more clear with a few examples.
Estimated Time
10 mins
Skill Level
Upper Intermediate
Functions
na
Course Provider
Provided by HolyPython.com
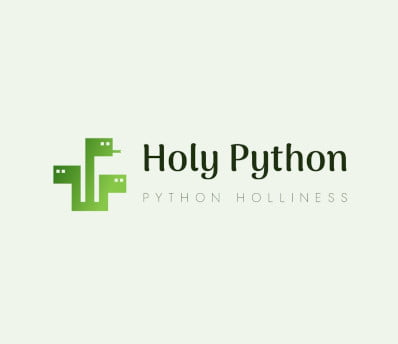
Used Where?
- A simple example is when you make data calls your data might not have the same shape and size. When you imply a try/except statement you’ll make sure your code doesn’t break down at the first mistake and actually keeps on running through the end.
- Another situation is when you make API calls you’ll often encounter json format with nested data in it. Similarly, different bits of data can have different keys or parameters and if this is the case your code will again break down. You can come over this with a simple try/except implementation.
- There can be countless situations where you may want to implement try and except. All the situations that would raise error types such as
- NameError,
- ValueError,
- AttributionError,
- IndexError,
- KeyError,
- SyntaxError,
- IOError,
- ImportError and so on.
If you’d like to see all the Built-in Python Error Types, you can click here.
Syntax do(s)
1) First block starts with try followed by an indentation and statement
2) Second block starts with except followed by an indentation and statement.
Syntax don't(s)
1) Don't forget the semicolons (:) after try and except statements.
Cool Tricks
Here are some Try / Except tips that will make coder’s life easier.
Avoid using BaseException:
- BaseException is at the top of the exception hierarchy.
- It will work but you probably want to avoid this type of implementation as it will catch even KeyboardInterrupt and SystemExit.
- It’s just not an elegant way to write codes and will potentially cause issues in complex and big implementations.
- You can also use
except Exception:
format and this will cause your code to overcome most of the exceptions. KeyboardInterrupt
andSystemExit
will still be executed with this method which can be welcome by the programmer as those statements might still be needed to stop or exit the program.- In the end, making vague exceptions like this is not a good programming practice and you should strive to be more specific with your exception unless you’re just practicing and having fun.
Advanced Details (Optional)
You can also record the error type or assign it to a variable to use it in a content or maybe a report or notification.
Error Type (Getting as an object or variable)
If you’d like to use the error type in your statement you can do so as well.
Also,
1) type(error)
will give you the class type of the error.
2) (type(error)).__name__
will give the exact name of the type of the error.
See example 5 below.
Key Takeaway Points:
- Operators are critical to know in coding
- They can make your life easier
- You can leave aside some operators in the beginning of your learning period or as long as you don’t ened them(i.e.:Bitwise)
- Some operators are important but easily confused or forgotten
//
division (floor):**
power:%
modulus:!=
not equal:>=
greater than:<=
lower than:in
in:not in
not in:
- Another confusion is “=” vs “==”. Former is used for assignments while latter is used in conditional statements: i.e.:
If a == 0:
Python Try / Except Statement Examples:
Here are some examples that can help you understand Python Operators and their use cases:
You can see free beginner Python lessons regarding data types, data structures, strings, integers etc. here.
Example 1
>>> a = 5
>>> b = 0
>>> try:
>>> print(a/b)
>>> except ZeroDivisionError:
>>> print(“You can’t divide with 0”)
“You can’t divide with 0“
try/except
where exception occurs and instead of throwing an error program executes whatever you want it to do. Example 2
>>> a = 5
>>> b = 0
>>> try:
>>> print(a/b)
>>> except:
>>> print(“You can’t divide with 0”)
“You can’t divide with 0“
Without specifying the ZeroDivisionError, it will still catch it with except statement.
Example 3
>>> a = [1, 3, 5]
>>> try:
>>> a.get()
>>> except:
>>> pass
Instead of specifying the AttributeError we used except and it will jump to the pass statement after the except. Please note that using a naked except is not good Python practice and can cause you trouble in sophisticated applications.
pass statement
, click here. Example 4
>>> a = “Hello World!”
>>> try:
>>> a + 10
>>> except BaseException as error:
>>> print(‘Exception occurred: {}’.format(error))
lst = [5, 10, 20]
Exception occured: can only concatenate str (not “int”) to str.
Example 5
lst = [5, 10, 20]
>>> try:
>>> print(lst[5])
>>> except IndexError as error:
>>> print(“Exception is: {}”.format(type(error).__name__))
IndexError
. Next Lesson: Python Classes