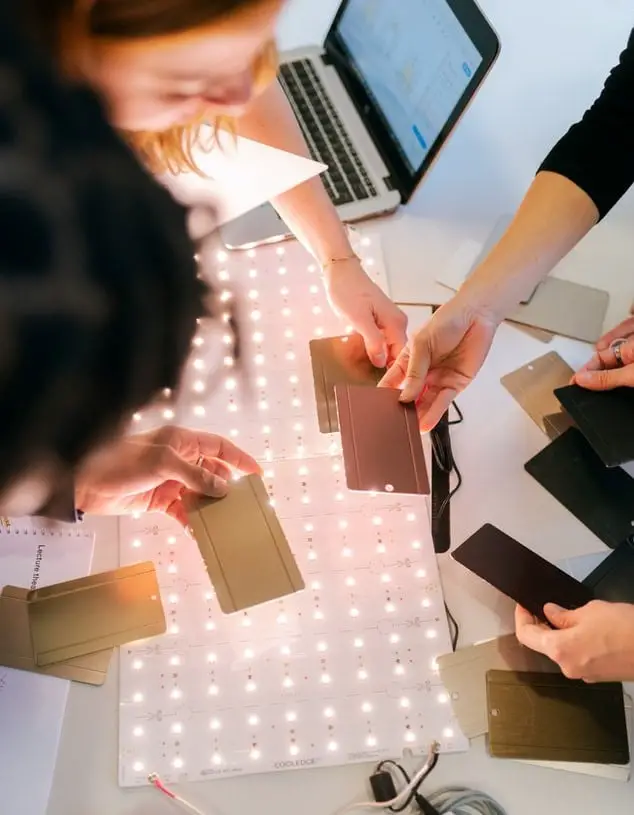
Locally installing and testing a Python library is such a good idea especially before uploading it to PyPI.
You might have tested the software itself during and after the development processes but there are so many new components after packaging. It’s particularly useful to build and install a library and you have multiple options that will help you understand packaging better and ensure a smooth operation when you’re finally uploading your library to PyPI.
Don’t worry we’ve tried hard to explain all these steps and options clearly below.
Estimated Time
10 mins
Skill Level
Upper-Intermediate
Exercises
na
Posts from the Series
Course Provider
Provided by HolyPython.com
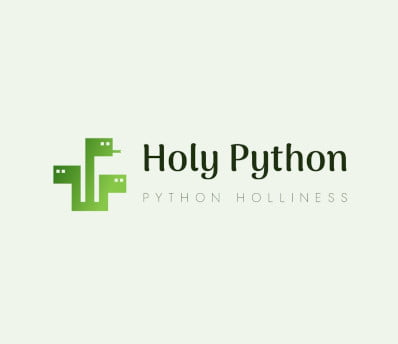
Install Library where it exists
install the library using the code below:
python setup.py install
Building VS. Installing
python setup.py install already takes care of two steps. It builds and then installs. But you just normally don’t see that build step take place as it happens.
python setup.py install
If you want to build separately without installing though it’s also possible. You just need to use this code:
python setup.py build
Install Library with pip Locally
This is the closest you get to a pip installation your audience will experience via pip command.
This way, your library will be added to site-packages folder in your Python installation folder. Python installation folder can differ based on the setup you’re using. For example, it’s normally under:
c:/Anaconda3/Lib/site-packages if you’re using Anaconda.
To proceed with installation this way, just ensure you are running command prompt (or Anaconda Shell) in the folder where your setup.py is residing. Then run the command below (Yes! There is a dot.):
pip install .
Uninstalling and Reinstalling with pip during the tests
As you proceed with your tests you may wanna make little changes and then test again. This might require uninstalling and re-installing your library to check if changes are taking place.
Please note that sometimes you might also need to close the Command Prompt, Anaconda Prompt, or Python IDE you’re working with, and then restart so that library loads up freshly without the old remains in the memory.
To uninstall a library simply run this command:
pip uninstall [LIBRARYNAME]
Testing __init__.py file
Another big aspect of local tests is testing the __init__.py files.
__init__.py file is responsible of loading up necessary modules and classes at appropriate stages. For examples if your source code is in a folder you don’t want to force user to do repetitive import tasks. And also, you don’t want to oblige user to import each class every time they import a library or module.
In that case we would have to do something like this every time we import a library:
- import folder
- import library
- import class a
- import class b
So, developers usually strive to eliminate unnecessary steps from the importing process and achieve something like this:
- import folder
- import library
- import class a
- import class b
and hopefully everything relevant gets loaded automatically. This depends on a proper structuring of __init__.py file. Don’t worry in practice it’s a couple of lines of code and not so difficult but it’s just important to get it right and sometimes it can be a bit complicated to do so. Explanations and examples below should help you get it right.
It makes perfect sense to test __init__.py file and see if its functioning as it should via a local installation. Other files such as Readme.md or setup.py will cause errors during installation or have visible flaws when something’s wrong but it’s possible to have a malfunctioning program due to __init__.py file despite a successfully executed installation. That’s why it’s wise to test the program locally in this sense as well.
Since this file’s behavior can be observed during and after the import it’s quite useful to take advantage of local tests to see if __init__.py is doing what it’s intended to be doing.
__init__.py file is used to load up modules, libraries and classes when a library is imported.
For instance when your library exists inside a folder, if you don’t include a proper __init__.py file, when your library is imported it will only import the folder name. Then the users will have to follow a repetitive calling structure such as
library.library.class.function
First library in this example is only the folder while the second library is the actual Python module.
You can also include multiple __init__.py files. It’s common practice to include a __init__.py file in the root folder to ensure proper importing of the main library and then several __init__.py files as needed to where modules exist. In the same folder with modules you will want to make sure classes of these modules are initialized inside the __init__.py files.
So, while __init__.py file in the root directory looks like this:
from .ABCDEF import ABCDEF
__init__.py file in the module’s folder may look something like this:
from .ABCDEF import MyClassName
This example is just to give an idea and your program might have specific needs regarding importing modules and classes. You will know it best as the developer and it can also help you find out to locally test the code and installation in that sense.
Also please note that after recent updates, including a dot before the importing source became a necessary step as in the code examples and forgetting that dot can cause problems.
So, while the first code is importing the actual library from the folder where it stays, in the second code certain Class is being imported from the actual library itself.
You can check out Watermarkd library in Holypython’s Github Repository to see __init__.py files in action both in the root folder and also in the Watermarkd folder where source code can be found.
Thanks for reading this turorial. Once you have everything down with the local tests and __init__.py files, next and final step for having a library on PyPI is Uploading a package to The Python Package Index PyPI.
Congratulations on your efforts and coming thus far!
Please share this article if you found it helpful.