Python API Exercises
Let’s check out some exercises that will help understand APIs with Python better.
Exercise 7-a
Try to print the suggested queries below by using:
- Requests library
- params parameter
- DataMuse API
Note: This exercise requires you to have your own Python setup. We recommend Anaconda Distribution as an easy and simple all-in-one solution, it’s also open source and free.
After importing requests library. You can use requests.get() to call data from the API.
If you remember the data that’s returned is requests type and if you try to print it “raw”, it will give you a code. (Some of the most common codes are 200: successful and 404: error)
To print the content in a readable way you need to try adding .text to your data.
params
parameter. i.e.:
import requests
par_list = ["words?sp=Kentucky"]
f = "https://api.datamuse.com/words?"
data = requests.get(f, params=par_list)
print(data.text)
This exercise doesn’t have one designated solution and the goal is that you experiment with the concept until you achieve a feeling of confidence with.
Exercise 7-b
loop
structure and .insert
method of the lists, add only the words in one of the queries that you choose to perform from above. for loop.
Inside your loop you can use .insert()
method to add items to your list.
Remember to create an empty list before you start your loop
Inside your loop you can use .insert()
method to add items to your list.
import requests
par_list = ["words?sp=Kentucky"]
f = "https://api.datamuse.com/words?"
data = requests.get(f, params=par_list)
print(data.text)
lst = []
for i in data1.text:
lst.insert(i[0])
print(lst)
Need More Exercises?
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
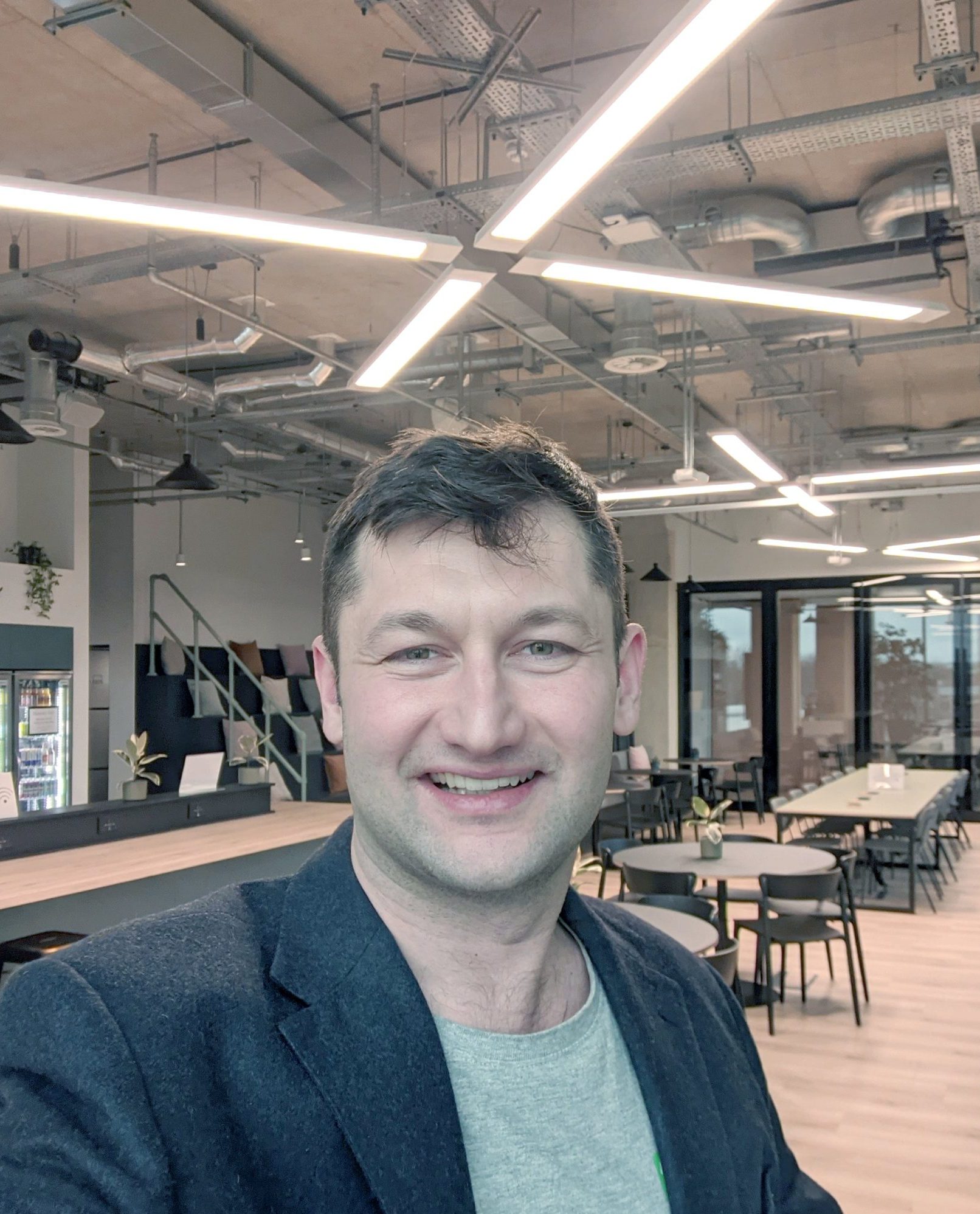
Founder of HolyPython