Python Class Exercises
Let’s check out some exercises that will help understand Classes better.
Exercise 4-a: Simple Class Attributes (name and origin)
A class regarding an imaginary jet inventory is already defined for you. Also an instant of this Jet class is created and assigned to variable first_item.
Print the name of the first_item.
Exercise 4-b: Simple attribute (origin)
This time print the origin of the first_item.
Exercise 4-c: Jet Fighter Instances
Create new instances until the sixth item following this order: F14, SU33, AJS37, Mirage2000, Mig29, A10. You can check Hint 1 for the origins.
SU33: Russia
AJS37: Sweden
Mirage2000: France
F14: USA
Mig29: USSR
A10: USA
You can create an instance as following:
first_item=Jet(name, country)
first_item=Jets("F14", "USA") second_item=Jets("SU33", "Russia") third_item=Jets("AJS37", "Sweden") fourth_item=Jets("Mirage2000", "France") fifth_item=Jets("Mig29", "USSR") sixth_item=Jets("A10", "USA")
Need More Exercises?
Check out Holy Python AI+ for amazing Python learning tools.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
*Includes 14 more programming languages, inspirational tools and 1-on-1 consulting options.
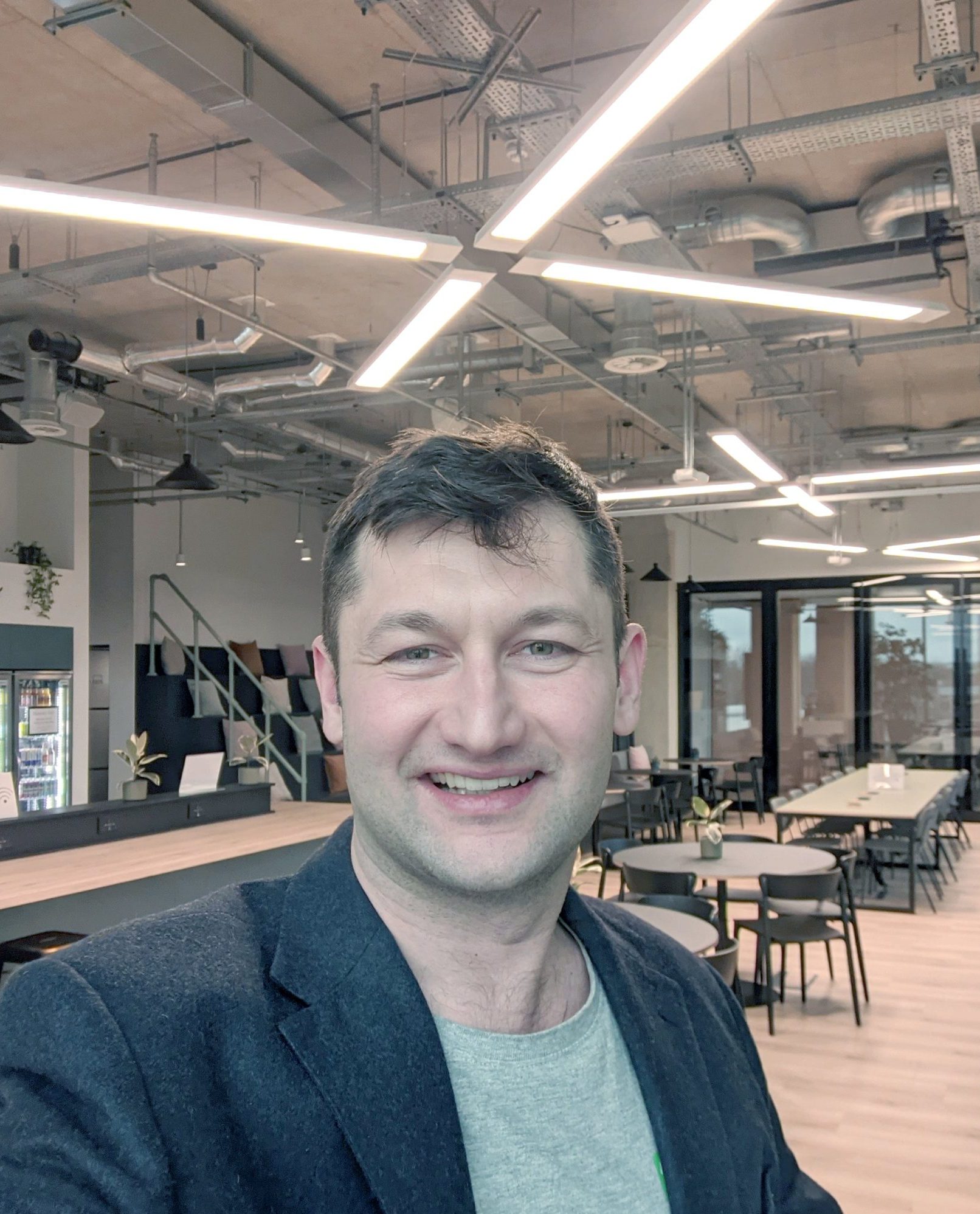
Umut Sagir
Finance & Data Science Professional,
Founder of HolyPython
Founder of HolyPython